Top Programming Tricks for Streamlined and Efficient Coding
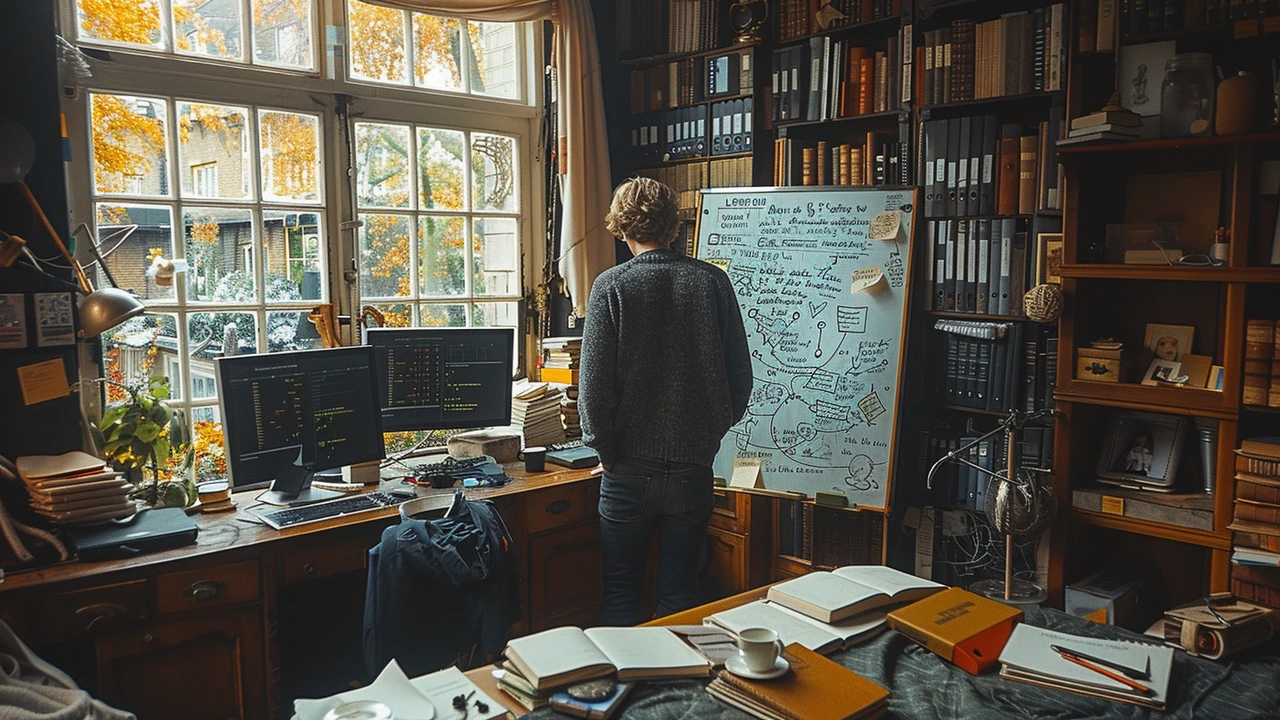
Coding can sometimes feel like solving a never-ending puzzle. But with the right tricks up your sleeve, it becomes a much smoother ride. This article dives into some of the best programming hacks that can make your coding not just easier, but more efficient. Whether you're a seasoned developer or just starting, these practical tips will help you improve your workflow and reduce errors.
Using Version Control
When discussing efficient coding, we must talk about using version control systems. It's a bit like having a magic undo button for your code, ensuring you can recover from any mistakes without losing progress. One of the most well-known version control systems is Git, used by developers worldwide. Git helps track changes in your source code over time, so you can revert to specific versions as needed, see who made changes, and collaborate seamlessly with other developers.
Version control is not just about individual benefits; it also fosters better collaboration. Imagine working with a team on the same codebase without stepping on each other's toes. With Git's branching and merging features, multiple developers can work simultaneously on different features. This ensures that everyone's efforts are integrated into the main project without conflicts.
Learning Git involves understanding a few basic commands. The 'commit' command saves your changes, while 'push' uploads them to a repository. Similarly, 'pull' retrieves updates made by others. These simple commands can significantly enhance your workflow, especially in collaborative environments. Keeping a well-maintained commit history is like documenting your project's life story, making it invaluable when debugging or adding new features.
"Version control is to software development what air conditioning is to modern architecture." - Paul Smith, Senior Developer
Another great feature of Git is the use of branches. Branches allow you to develop features or fixes in isolation from the main codebase. When you're satisfied with the changes, you can merge the branch back into the main project. This approach makes it easier to manage complex projects and ensures that the main branch remains stable and functional.
For those new to coding, platforms like GitHub and Bitbucket offer user-friendly interfaces to manage repositories. These platforms also provide additional features like issue tracking, project management, and code reviews, which can be incredibly useful for both individual projects and team collaborations. In fact, a survey by Stack Overflow revealed that over 87% of developers use Git for version control, highlighting its importance in modern software development.
Tools like GitHub Desktop offer a graphical interface for managing your repositories, making it even easier for beginners to get started. GitHub also offers extensive documentation and tutorials, so no matter your skill level, you can find resources to help you master version control.
Embracing version control can significantly improve your coding efficiency and workflow. Whether working on a solo project or as part of a large team, mastering version control concepts and tools like Git is essential for modern programming. Not only will it save you countless hours of work, but it will also make your codebase more organized and maintainable in the long run.
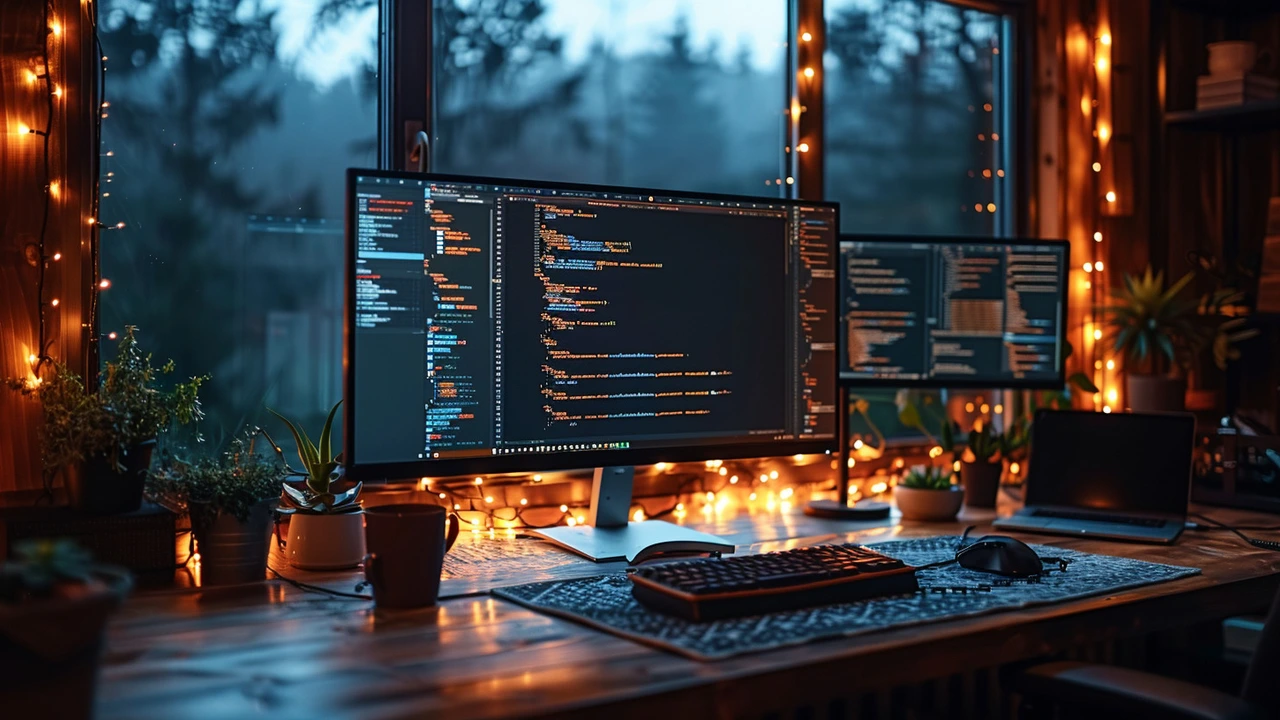
Mastering Shortcuts
When it comes to efficient coding, knowing your keyboard shortcuts can be a game changer. These shortcuts are designed to help you perform tasks quicker, eliminate unnecessary steps, and streamline your workflow. Whether it’s copying, pasting, or navigating through your code, mastering these combinations will save you precious time.
For instance, simple shortcuts like Ctrl+C and Ctrl+V for copying and pasting are just the tip of the iceberg. A seasoned coder might use shortcuts for opening, closing, and toggling between files. Learning these can drastically reduce the time you spend on mundane tasks and let you focus more on problem-solving and actual coding. Moreover, most integrated development environments (IDEs) provide a range of tailored shortcuts for specific actions—like Ctrl+Shift+R in Eclipse for refactoring code or Ctrl+P in VS Code to quickly open files.
Understanding these shortcuts is crucial because they enhance productivity and accuracy. When everything at your fingertips, you waste less time on navigation and avoid breaking your concentration. This can be particularly useful during intense coding sessions where every second counts. One interesting fact is that some studies have shown that programmers who heavily use shortcuts are up to 30% more efficient than those who rely solely on the mouse.
"Keyboard shortcuts are crucial for developers who want to maximize their productivity. They prevent you from getting interrupted by trivial tasks and keep you focused on coding," says John Resig, creator of the jQuery JavaScript library.
To get started, it’s helpful to write down the shortcuts you most frequently use and try to incorporate them into your workflow until they become second nature. Many developers say that once you’re accustomed to this new way of working, you’ll wonder how you ever coded without it. Another tip is to customize shortcuts in your IDE to fit your preferences. This can make them even more intuitive and increase your speed further.
So, make a habit of practicing these shortcuts. The initial investment in learning them will pay off significantly in the long run. Just remember, the goal is to make these actions second nature, so you type them without thinking—this is where true efficiency lies.
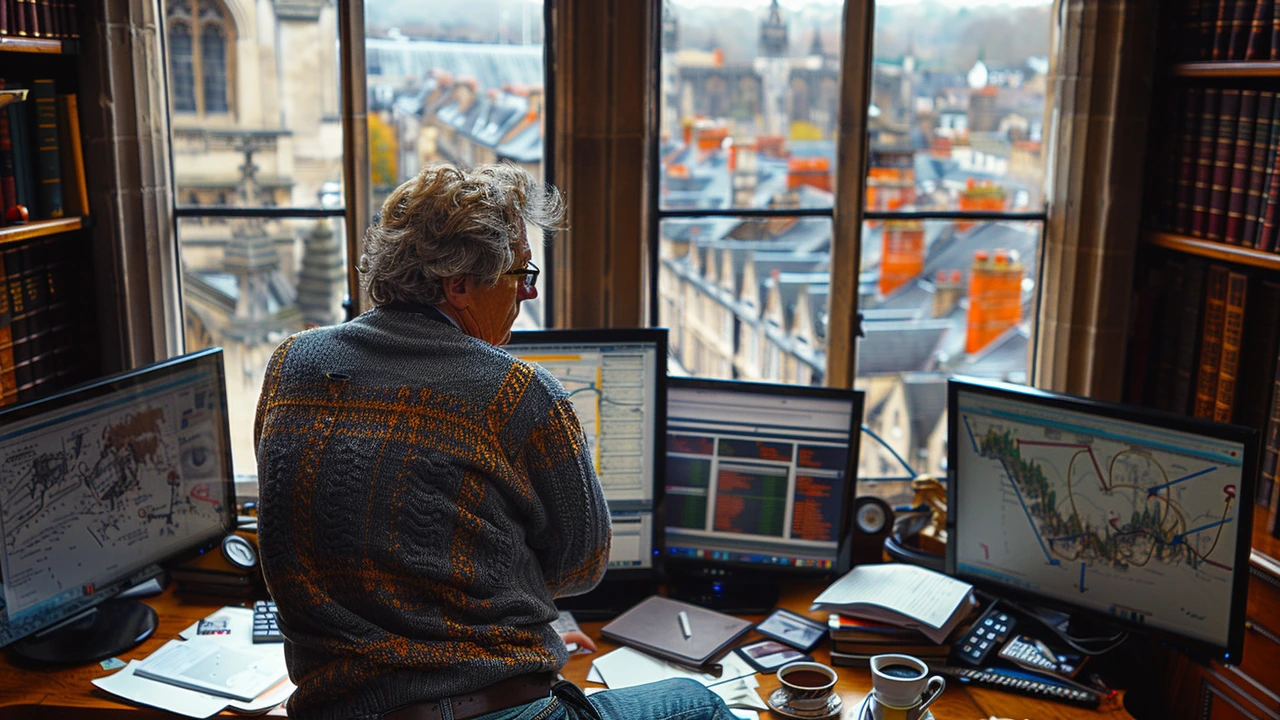
Writing Clean Code
Writing clean code is a fundamental practice that every programmer should master. It's not just about making the code work, it's about making it understandable and maintainable for others and even for your future self. One of the main principles of clean coding is simplicity. The simpler your code, the easier it is to read and maintain.
A great starting point is naming your variables, functions, and classes meaningfully. Names should reveal the intent behind the code. If a variable stores a user's age, naming it userAge makes it clear. Avoid using single-letter names or unclear abbreviations unless it's for loop counters or similarly limited scope usages.
In addition to naming, focus on keeping your functions small. Each function should perform one task and it should do it well. This concept is known as the Single Responsibility Principle. Smaller functions reduce complexity and make testing and debugging easier. When a function tries to do too much, it often becomes difficult to understand and maintain.
Alongside function size, proper indentation and spacing are also crucial. Code that is well-formatted is easier on the eyes and helps in understanding the structure at a glance. Modern code editors usually provide automatic formatting tools, so take advantage of them. Consistency in your formatting style is key, and many teams adopt style guides to standardize this across all their code.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
Another important aspect is avoiding code duplication. Duplicated code means more maintenance and higher risk of introducing bugs when changes are necessary. Refactor your code to eliminate duplications by creating reusable functions or classes. Refactoring is a powerful technique to improve the structure of your existing code base without changing its behavior.
Comments also play a significant role in writing clean code, but they need to be used judiciously. Comments should explain why something is done, not what is done, because well-written code can often be self-explanatory regarding what it does. Overcommenting or using comments to explain bad code is not a solution; instead, refactor the code to make it clearer.
Lastly, incorporating code reviews into your workflow is a great way to ensure clean code. Code reviews involve having another set of eyes look over your code, which can help catch mistakes you might have missed and provide insights into how your code can be improved. It’s a collaborative way to maintain high coding standards and share knowledge within the team.
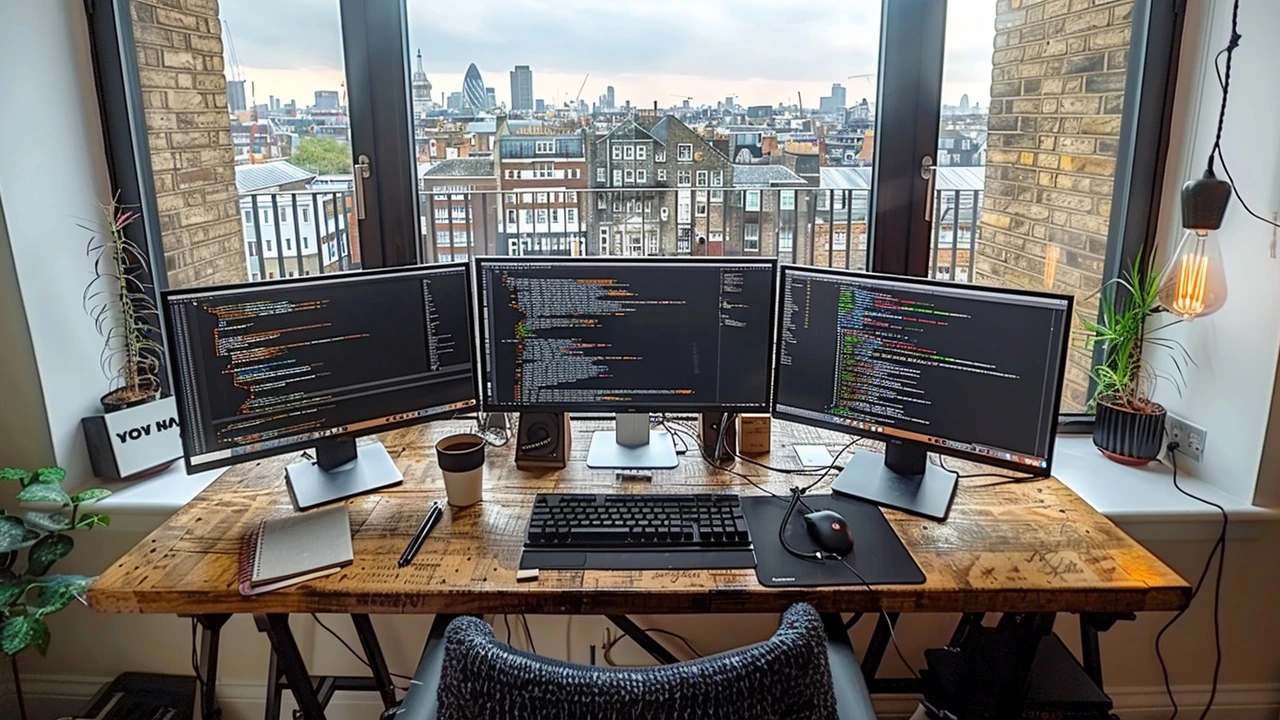
Automating Tasks
Automating tasks is one of the most effective ways to save time and boost efficiency in programming. By writing scripts or using tools that perform repetitive tasks automatically, you can focus on more complex aspects of your projects. One of the simplest and most used automation tools is a Task Runner. Tools like Gulp or Grunt allow you to automate tasks such as minifying JavaScript files, compiling Sass to CSS, or even live-reloading a webpage when you make changes to your code.
A script can be as simple as a few lines of code that automate routine tasks, or as complex as a full-blown application that handles multiple operations simultaneously. For instance, Continuous Integration (CI) tools like Jenkins or Travis CI can automate the process of testing code, building applications, and even deploying them. Automated tests can run every time you commit code to your repository, catching errors before they make it into production. This not only saves time but significantly reduces the risk of bugs.
Another great example of task automation is using Command Line Interface (CLI) tools. With CLI tools, you can write scripts that automate database backups, send out regular reports, or even generate boilerplate code for new projects. Git, for example, has many automated features. You can use hooks to run scripts before and after certain actions, such as committing code or merging branches. Automating with Git hooks can help ensure code quality by running linters and tests automatically before pushing changes.
According to a report by McKinsey, more than 45% of activities people are paid to perform can be automated. This is particularly relevant in software development, where many tasks are repetitive and rule-based. By embracing automation, developers can significantly improve their productivity. A famous quote by Steve Jobs also resonates with the power of automation:
“I don't want to spend my life developing software; I want to spend my life developing better software.”
Investing time early on to automate mundane tasks can result in huge time savings in the long run. For example, onboarding a new team member can be automated by scripts that set up their development environment, granting them the right permissions, and even sending welcome emails.
It's also important to document and maintain your scripts and automation tools. Regularly updated documentation can save you from potential headaches down the line when something breaks, or the automation process needs tweaking. A good starting point is to maintain a README file in your repository that details what each script does and how to run it.
In summary, automating tasks not only saves time and reduces errors but also lets you focus more on creative and strategic aspects of development. By leveraging task runners, CI tools, CLIs, and other automation technologies, you can vastly enhance the efficiency of your coding process.