Python Tricks: Must-Know Gems for Every Developer
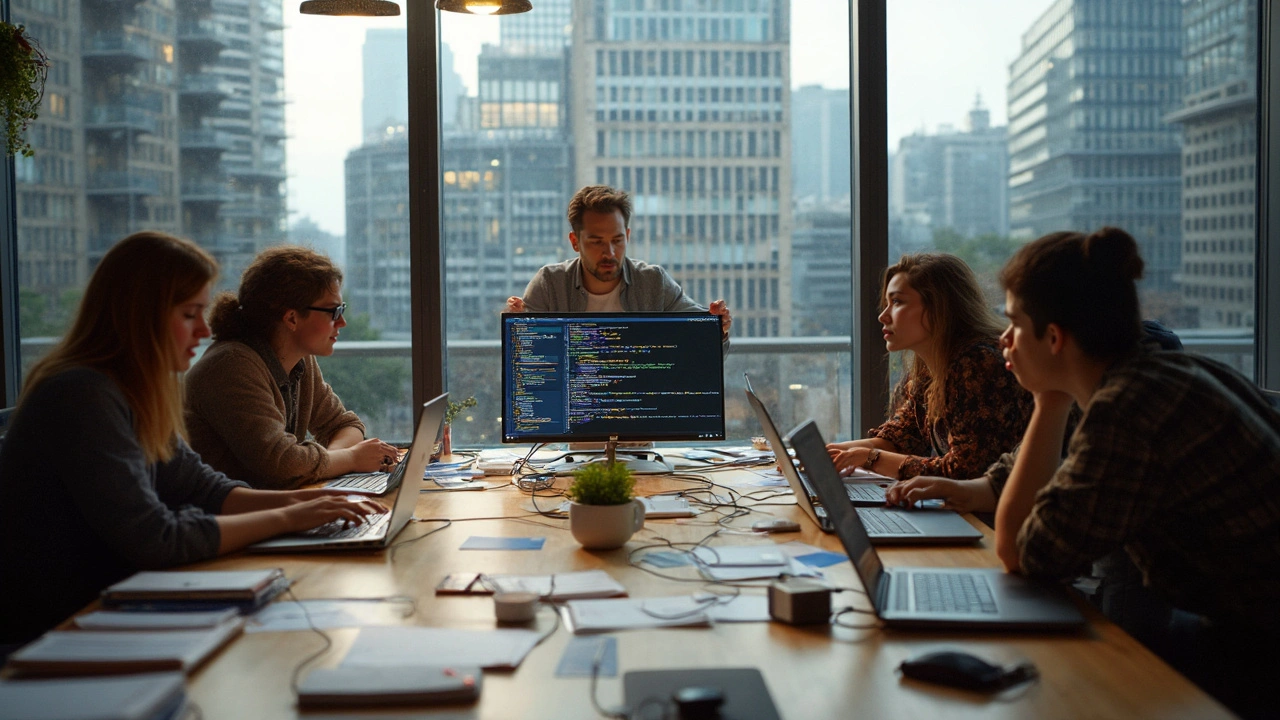
Did you know you can turn complex loops into one-liners with Python's list comprehensions? They're not just nifty; they're essential for writing clean, efficient code. Imagine swapping a bloated five-line loop with a sleek one-liner that does it all. That's the power of list comprehensions. Let's check it out.
Next up, lambdas. Don't let the name intimidate you—they're just tiny anonymous functions that fit right in when you need them. Whether you're sorting lists or pairing them with map functions, lambdas can streamline your code elegantly. If you've ever felt tangled up with function definitions, give lambdas a whirl.
- Unleash List Comprehensions
- Harness the Power of Lambdas
- Error Handling Like a Pro
- Simplifying with Pythonic Shortcuts
Unleash List Comprehensions
Let's talk about the magic of Python's list comprehensions. They're like your Swiss Army knife in coding, letting you perform loops, conditions, and even nested operations in a single, clean line. You can reduce a five-line block of code down to one that's easy to read and super efficient. No pointless redefining of variables or endless looping—just straight-to-the-point functionality.
Why Use List Comprehensions?
If you care about performance, you're in for a treat. List comprehensions not only look cleaner but run faster than traditional `for` loops. Sounds like a win-win, right? For heavy lifting like data manipulation or filtering lists, these comprehensions can make a noticeable difference in execution time. A study showed they can be up to 20% faster on certain tasks!
Getting Started with Examples
The basic form looks something like this:
new_list = [expression for item in iterable if condition]
So what does that mean? Here's a breakdown:
- Expression: Whatever you want to do to each item.
- Item: Each element in your list.
- Iterable: The original list you're looping through.
- Condition: (Optional) If you need filtering based on criteria.
Practical Uses
Let's say you have a list of numbers, and you want a new list of their squares:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x**2 for x in numbers]
Need to filter while you're at it? Try this:
even_squares = [x**2 for x in numbers if x % 2 == 0]
Just one line and you're cooked up efficient, readable code!
Watch Out for Pitfalls!
While list comprehensions are cool, avoid over-complicating them. If your expression starts looking like a mess, it might be wiser to switch back to traditional loops for the sake of clarity. Remember, the goal is cleaner, not fancier.
Harness the Power of Lambdas
Lambdas in Python are like your trusty, pocket-sized tools. Imagine a world where you can create functions on the go without the baggage of full-fledged definitions. That's exactly what lambdas do. These tiny, unnamed functions bring succinctness to your code, letting you do more with less.
What are Lambdas?
Simply put, a lambda is a function without a name. It's defined using the keyword lambda
, followed by parameters, a colon, and an expression. The magic of a lambda is in its compact, one-line existence. It's perfect for short, throwaway functions you need just once.
Why Use Lambdas?
Lambdas shine in situations where full-blown functions feel excessive. Need a quick sorting tweak? Lambdas are up for the task. Want a brief transformation within a map or filter? Lambdas are there. They're not replacing traditional functions, but they're unbeatable for their simplicity in many scenarios.
Getting Started with Lambdas
Using lambdas is as easy as pie. Here's what a basic lambda expression looks like:
add_five = lambda x: x + 5
Now, add_five(3)
will return 8. See the conciseness in action? It's almost like magic.
Lambdas in Practice
Let's look at some real-world applications:
- Sorting: Want to sort a list by the second item in tuples?
pairs = [(1, 'one'), (2, 'two'), (3, 'three')]
sorted_pairs = sorted(pairs, key=lambda item: item[1])
- Mapping: Easily apply a transformation to all list elements.
numbers = [1, 2, 3, 4]
squared = map(lambda x: x**2, numbers)
The power of lambdas extends well beyond just these examples. It's about turning complexity into clarity and transforming bulky code into a sleek vessel of logic.
Lambdas are your lightweight allies in the coding world. Next time something feels bloated, grab a lambda and watch it trim down like magic.
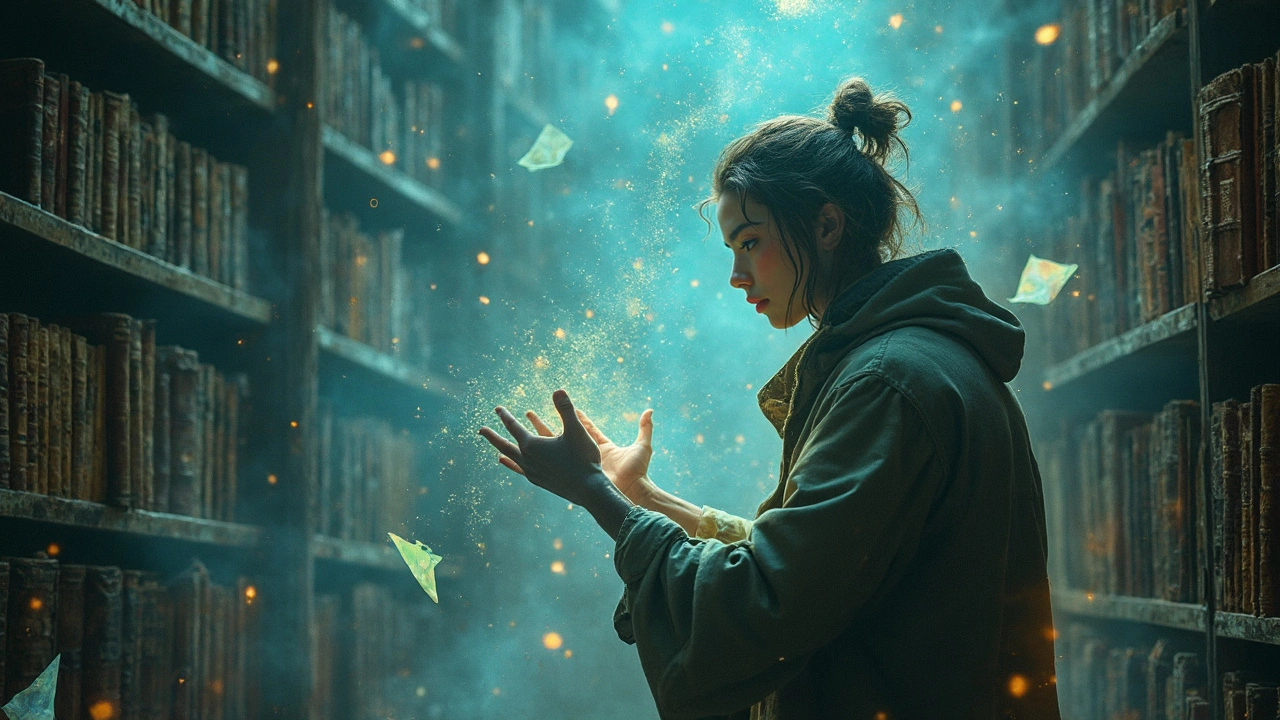
Error Handling Like a Pro
Handling errors in Python doesn't have to be the headache it often seems. Good error-handling is like having a safety net ready to catch you when something inevitably goes sideways. And trust me, it will. But you're not alone—Python's tools will be your best friend here.
Try and Except Blocks
First, familiarize yourself with try and except blocks. These are your go-to tools for catching exceptions. Think of try as where you hint at potential problem areas, and except as where you form a rescue plan when things go wrong.
Here’s a basic example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Can't divide by zero!")
Pretty straightforward, right? This snippet gracefully catches the notorious ZeroDivisionError, preventing your program from crashing.
Else and Finally Clauses
It doesn't stop with just try and except. Meet else and finally, two other heroes in error handling.
- Else executes a block of code if there's no exception. It keeps your non-error operations neatly separated.
- Finally runs no matter what, perfect for cleanup tasks like closing files and releasing resources.
Here's yet another snippet for the curious:
try:
my_file = open('file.txt', 'r')
content = my_file.read()
except FileNotFoundError:
print("File not found!")
else:
print("The file was read successfully.")
finally:
my_file.close()
Custom Exceptions
Sometimes the built-in exceptions just don't cut it. That’s where custom exceptions shine. Define the specific errors your application might face for more readable and debuggable code.
class MyCustomError(Exception):
pass
try:
raise MyCustomError("Custom error occurred!")
except MyCustomError as e:
print(e)
This code raises a custom exception and gracefully catches it. Easy peasy.
Robust error handling may feel a bit overwhelming at first—but master these tricks, and you’re well on your way to becoming a top-notch developer! Not only will it make your code more reliable, but it will also save you loads of debugging time down the line. So go ahead, handle those errors like a true pro!
Simplifying with Pythonic Shortcuts
Ever found yourself writing out long-winded code, only to feel like there must be a faster way? Welcome to the world of Python tricks that can trim down your code and boost efficiency. Python is all about simplicity and readability, and there are these nifty shortcuts you can use to keep your code clean and concise.
One of the simplest yet most underused features in Python is multiple assignment. Say goodbye to multiple lines of variable declarations. Instead, in a single line, just do:x, y, z = 1, 2, 3
. Voila! You've initialized three variables in one go. It's clean and saves time.
Using the Power of 'in'
Got a list and need to check if it contains a specific item? Instead of looping through each element, try using the in keyword. It's a straightforward way to check membership. For instance: if 'apple' in ['banana', 'orange', 'apple']: returns true, because—yep, 'apple' is indeed in there.
Swap Values without a Temp Variable
Remember the old-school way of swapping values using a third variable? With Python, you can swap effortlessly without that extra baggage:
x, y = y, x
In one line, your values are swapped, and it reads just like natural language.
Conditional Expressions
Sometimes you want to assign a value based on a condition. The Python way? Use a single-line conditional expression, known as a ternary operator. Instead of writing a full if-else block, do this:
result = 'Success' if score > pass_mark else 'Try Again'
. It simplifies your logic and fits well in statements where you need it most.
Joining Strings
Concatenating strings from a list? Instead of iterating, use join:sentence = ' '.join(['Python', 'tricks', 'are', 'cool'])
. It's efficient and typical of writing compact Python code.
Python tricks significantly reduce your code length, make code more readable, and even help you write like a pro with less effort. These tricks don't just save lines but also amplify productivity. So, next time you're coding, try wearing your Pythonic lens and see the difference.