Mastering Code Debugging: Essential Strategies for Developers
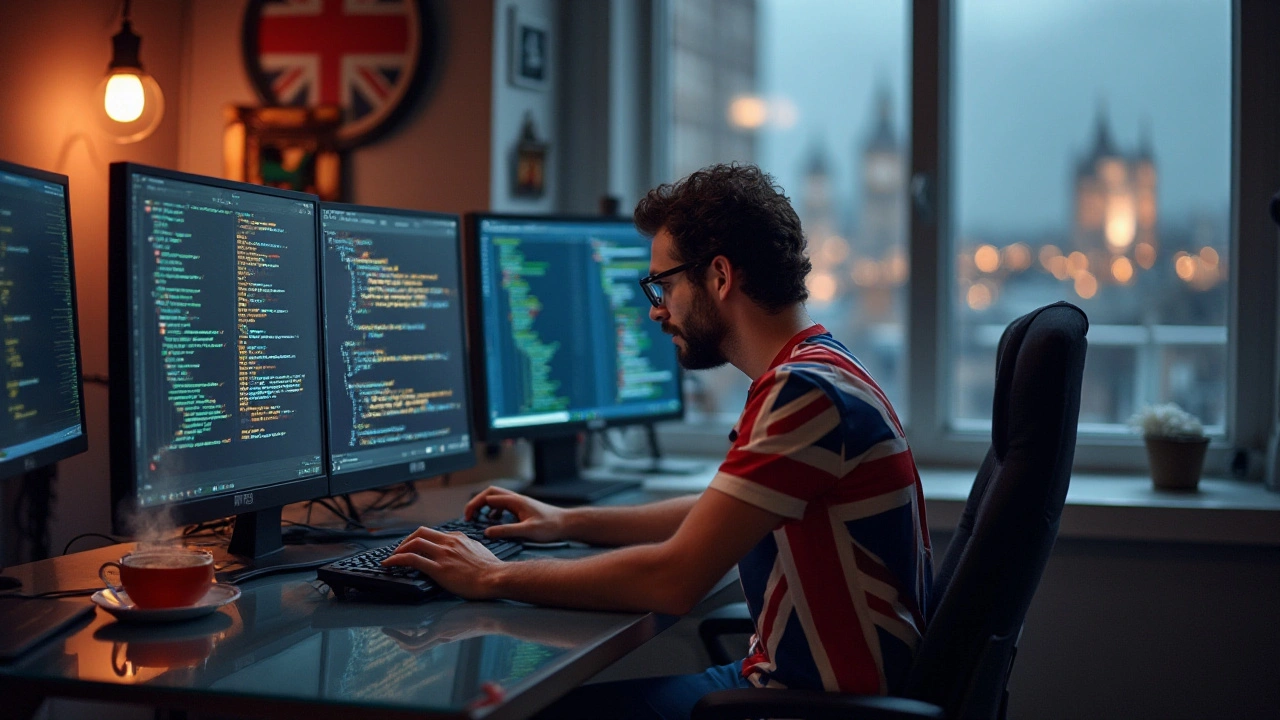
Debugging is an art form every developer must master. It can feel like searching for a needle in a haystack, but with the right approach, it becomes an exciting challenge rather than a chore. Whether you're a seasoned coder or just starting out, approaching debugging systematically can save you time and countless headaches.
This guide offers practical advice and effective strategies for diagnosing code issues. Exploring common error types, leveraging powerful tools, and adopting mindful practices will help you become a more efficient and confident debugger. Embrace the debugging journey and transform potential frustrations into learning experiences.
- Understanding Common Errors
- Tools and Techniques
- Productive Debugging Practices
- Dealing with Stress and Frustration
Understanding Common Errors
When diving headfirst into code, developers often face a seemingly endless barrage of errors. These glitches can appear daunting, but many are manifestations of recurring themes and common misconceptions. Identifying these typical snags swiftly can not only save precious time but also prevent the frustration often associated with the code debugging process. Grasping the essence of these errors starts with understanding the underlying architecture and syntax nuances that every programming language introduces. For instance, syntax errors, often typified by missed semicolons or mismatched brackets, scream at the coder immediately, demanding attention with verbose compiler errors.
Moreover, logic errors are notorious for slipping through the cracks during initial checks. These errors don't scream at you. Instead, they lurk in your code, waiting until the logic deviates silently. The tragic relatable example of ordering if-else conditions incorrectly serves as a cautionary tale. These sneaky discrepancies necessitate a developer's keen detective skills more than any other type of error. As Dennis Ritchie once quipped,
"The only way to learn a new programming language is by making mistakes in it."His insight highlights the reality that these errors, often perceived as hindrances, are actually indispensable learning opportunities.
To effectively tackle common errors, understanding type-related issues is pivotal. Languages like JavaScript embrace dynamic typing, leading to quirky bugs when unexpected data types muddy the waters. Variables designed to hold integers being fed strings can lead to bugs, driving even veteran developers to the brink of exasperation. Drawing from personal anecdotes and collective wisdom, a List of potential errors — and their diligent investigation — can be transformative. Reading documentation, focusing on typographical suggestions in IDEs, and recognizing infamous pitfalls enrich the coder's arsenal for battling errors. Through patience and practice, these routine mistakes morph into memorable learning hooks.
Often underestimated, error troubleshooting can involve combing through stack traces, log files, and sometimes, peer code reviews. Encountering new, unusual errors tends to yield rich insights. Establishing the root cause requires understanding the environmental setup and dependencies of the project. Different environments harbor different constraints, and oftentimes, the devil is in the details. Documenting these findings results in robust insights and can later aid developers facing similar challenges. Interestingly, data from development environments show that roughly 70% of developers encounter syntax and logical errors in the initial stages of code implementation, underpinning the universal nature of these obstacles.
By demystifying and recognizing common errors, developers cultivate not just technical acuity but also an elevated level of programming survival. They become code sleuths, equipped to dissect erroneous blocks of code with precision and purpose. When practiced consistently, the skill of debugging transcends into a craft, paving the path to more significant programming endeavors with grace and ease. And so, armed with the foresight and expertise from past errors, developers can look forward to sailing through code with confidence and clarity, leading towards the horizon of innovation.
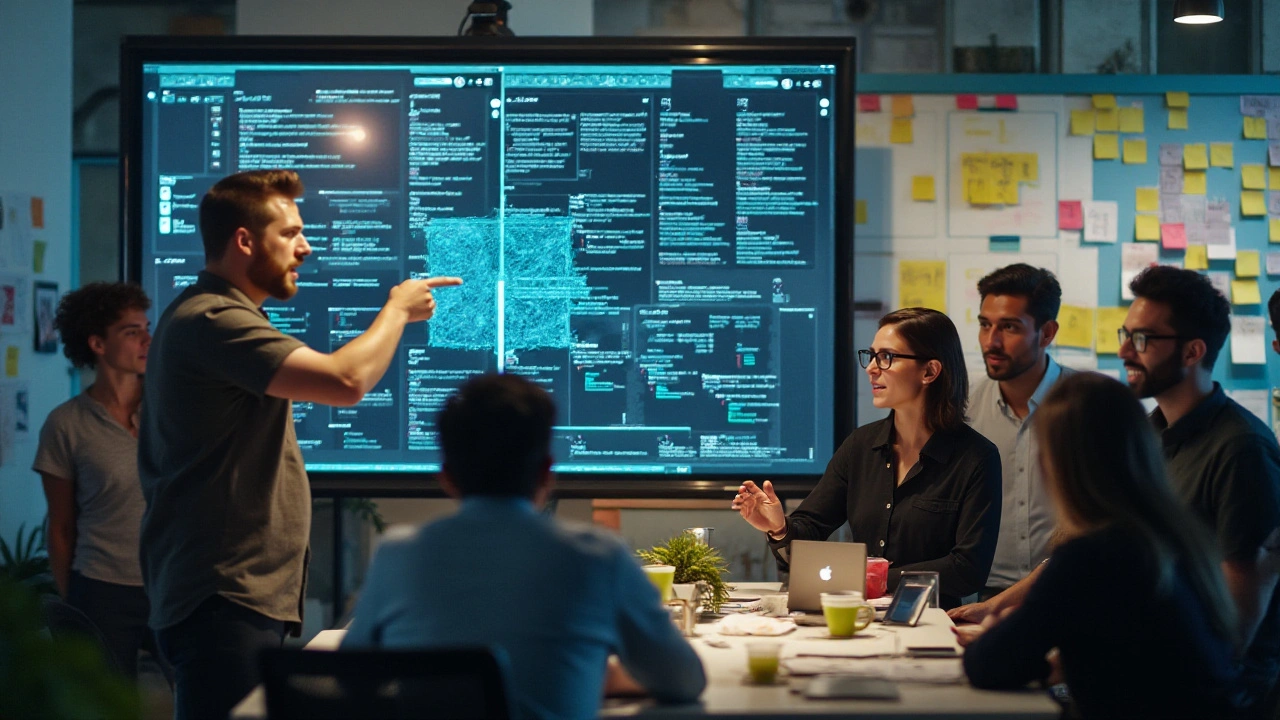
Tools and Techniques
Equipping oneself with effective debugging tools and employing the right techniques can make a world of difference in identifying and resolving code errors swiftly. Among the primary tools, integrated development environments (IDEs) like Visual Studio Code, IntelliJ IDEA, and Eclipse stand out for their comprehensive built-in debugging tools. They allow developers to set breakpoints, step through the code line by line, and inspect variables in real-time. Such features are crucial when diagnosing why a particular function isn't behaving as expected, offering insights into both syntax and logical errors.
Command-line debuggers, such as GDB for C or LLDB for Swift, offer more flexibility and are preferred by those who enjoy a more granular approach. They provide the power to dissect code execution apart from the visual ambiance of an IDE. While it might seem intimidating at first, mastering these tools arms developers with a profound understanding of program flow and resource management. Debugging techniques like binary search debugging and the 'print statement' technique are timeless and invaluable, requiring minimal setup yet offering major guidance in pinpointing the problematic code.
Utilizing version control systems, like Git, alongside collaborative platforms such as GitHub, enables developers to not only track changes that may lead to errors but also collaborate with peers to review their code. Often, a fresh pair of eyes can spot what might go unnoticed by the original author. Incorporating code linters and static analysis tools can preemptively catch a myriad of errors, saving hours of troubleshooting later. These tools analyze code for potential bugs and stylistic errors, suggesting improvements that enhance code quality and readability.
"The most important single aspect of software development is to be clear about what you are trying to build." - Bjarne Stroustrup
Proficiency in these tools and techniques translates into greater productivity and fewer sleepless nights for developers. As projects become more complex, automated testing services like Selenium for web applications or JUnit for Java applications are becoming indispensable. They allow repetitive, time-consuming testing tasks to be run automatically, allowing developers to focus their energies on innovation rather than routine checks. In a 2022 survey, it was noted that 87% of developers reported significant time-saving by integrating automated testing in their workflows.
Balancing these resources and techniques not only aids in immediate problem-solving but also fosters confidence and a deeper understanding of one's codebase. By investing time in learning and honing these debugging skills, developers can transform debugging from a daunting task into a rewarding part of the development cycle.
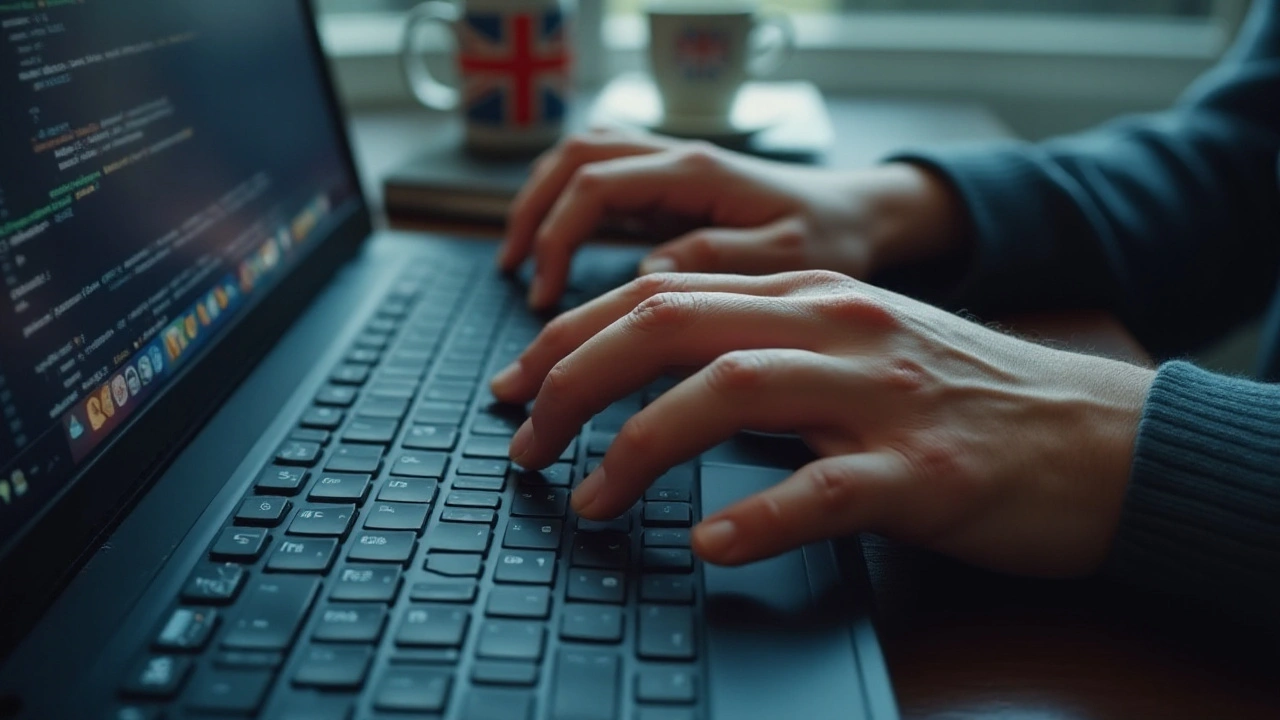
Productive Debugging Practices
Mastering code debugging is not merely about finding and correcting errors; it’s an integral aspect of the development process that can significantly impact your workflow and efficiency. A productive debugging practice begins with cultivating a mindset that sees error detection as an opportunity to enhance your problem-solving skills. Seasoned developers understand the value of patience and persistence, knowing that with each bug conquered, their knowledge base expands. This section provides insights and proven strategies to transform debugging into a seamless and productive experience. One fundamental technique is to familiarize yourself with the problem. Before diving into the code, reproduce the issue reliably. This not only confirms the presence of the bug but also narrows down the scope, making isolation of the error more manageable.
It's essential to weaponize yourself with an arsenal of tools to streamline your debugging process. Utilizing advanced debugging tools can substantially reduce the time spent on resolving issues. Many software environments include built-in debuggers that offer breakpoints, watch windows, and call stacks, helping you identify where the error troubleshooting should begin. By mastering these tools, you can navigate through complex code bases more efficiently. Pairing these with logging practices can be incredibly beneficial; logging provides a history of events that led to the failure, offering invaluable clues.
Debugging is like being a detective in a crime movie where you are also the murderer. - Filipe FortesAnother indispensable practice is writing clear and coherent code; it cannot be overstated how much clarity in your code can impact the debugging process. Well-commented code not only helps you but also others who might be part of the future troubleshooting journey.
The strategic approach extends to how you handle the debugging process itself. Rather than tackling extensive sections of code, focus on smaller units or modules. This segmentation simplifies the task by allowing you to methodically test individual components before integrating them. Employ a hypothesis-driven approach—make an educated guess about the error’s cause, test it, and refine your hypothesis based on the results. Applying this scientific method logic can save considerable time and effort. Incorporating automated tests can be another productive practice; unit tests, integration tests, and system tests ensure that your code behaves as expected across various scenarios. This not only catches errors early but also boosts your confidence in building larger systems without dreading the debugging phase. These practices, when adopted and honed, become second nature, ensuring your debugging journey is as smooth and effective as possible.
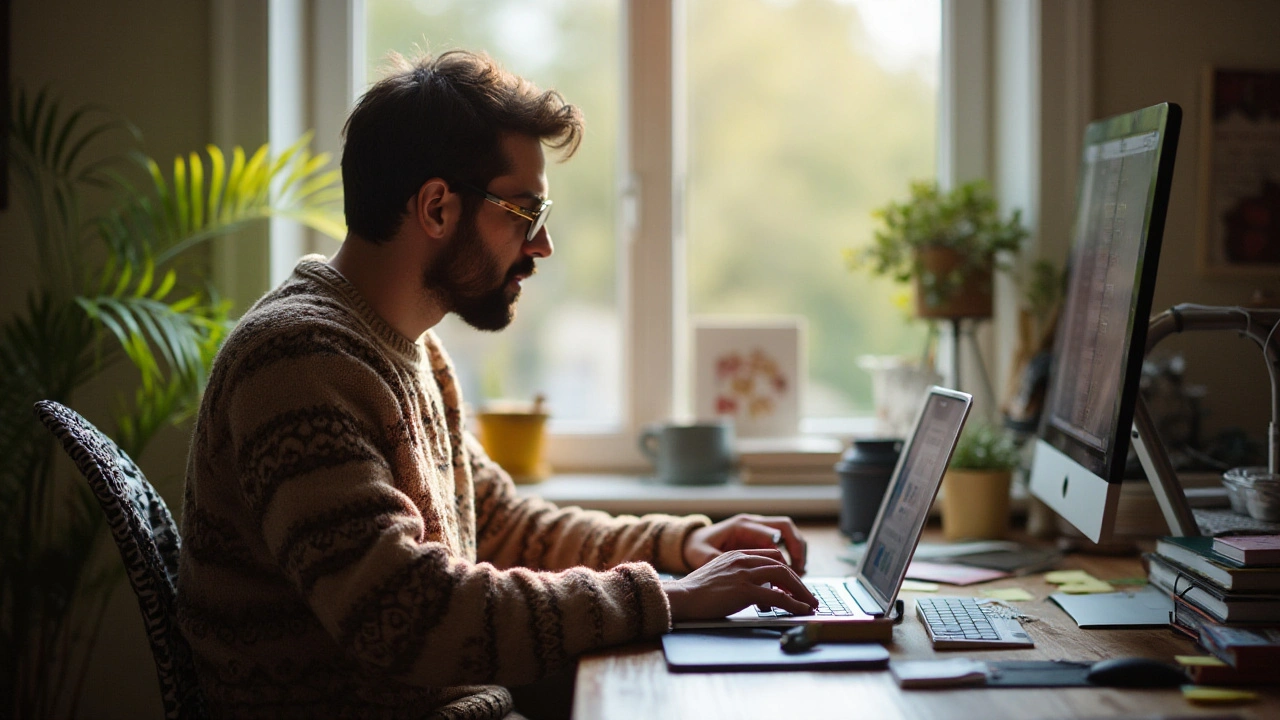
Dealing with Stress and Frustration
Any programmer can tell you that debugging is as much a mental game as it is a technical task. Long hours spent hunting down elusive errors can leave even the most experienced developers feeling overwhelmed. This stress often stems from the pressure to deliver flawless code within tight deadlines. To manage this, developers need to adopt techniques that help them tackle stress actively, turning a daunting debugging process into a manageable series of steps.
One effective approach is to practice mindfulness and take regular breaks away from the screen. Significant studies have shown that stepping away—even if just for a few minutes—refreshes the mind, offering a new perspective upon return. Engaging in activities such as walking, meditating, or simply getting a dose of fresh air can help in resetting the brain's focus. Prioritizing physical well-being through proper hydration, nutrition, and exercise is essential. Developers often find themselves in a zone, reaching for caffeine to power through. However, a balanced diet can actually improve mental clarity, making error troubleshooting a lot less strenuous.
Collaboration and support networks are another crucial element in managing the emotional toll of debugging. Sharing struggles with fellow developers can often lead to solutions, as peers may offer fresh insights or recall similar issues they have faced. According to a study by the University of California, over 70% of developers reported that discussing debugging problems with colleagues helped them resolve issues more efficiently. This not only lightens the emotional load but also builds a community spirit, strengthening professional bonds.
Loretta Poole, a senior software engineer at TechSolutions, emphasizes this point, "Debugging doesn't have to be a solitary battle. Engaging with a supportive team transforms potential frustration into collective effort and learning."
It's also essential to cultivate a resilient mindset, viewing each bug as a learning opportunity rather than a roadblock. Documenting successful strategies used in past debugging challenges can serve as a quick reference guide for future issues, reducing stress by providing a structured plan. Embracing mistakes as an integral part of the learning curve can significantly decrease frustration levels, turning setbacks into stepping stones for growth. Reinforcing this mindset involves celebrating small victories, which builds morale and maintains motivation.
In the high-pressure world of software development, dealing with stress and frustration effectively is a skill just as vital as any technical ability. By integrating lifestyle adaptations, seeking communal support, and fostering a positive outlook, developers can not only survive but thrive in their code debugging journeys, enhancing their productivity and overall job satisfaction.