Mastering JavaScript: A Guide to Crafting Interactive Websites
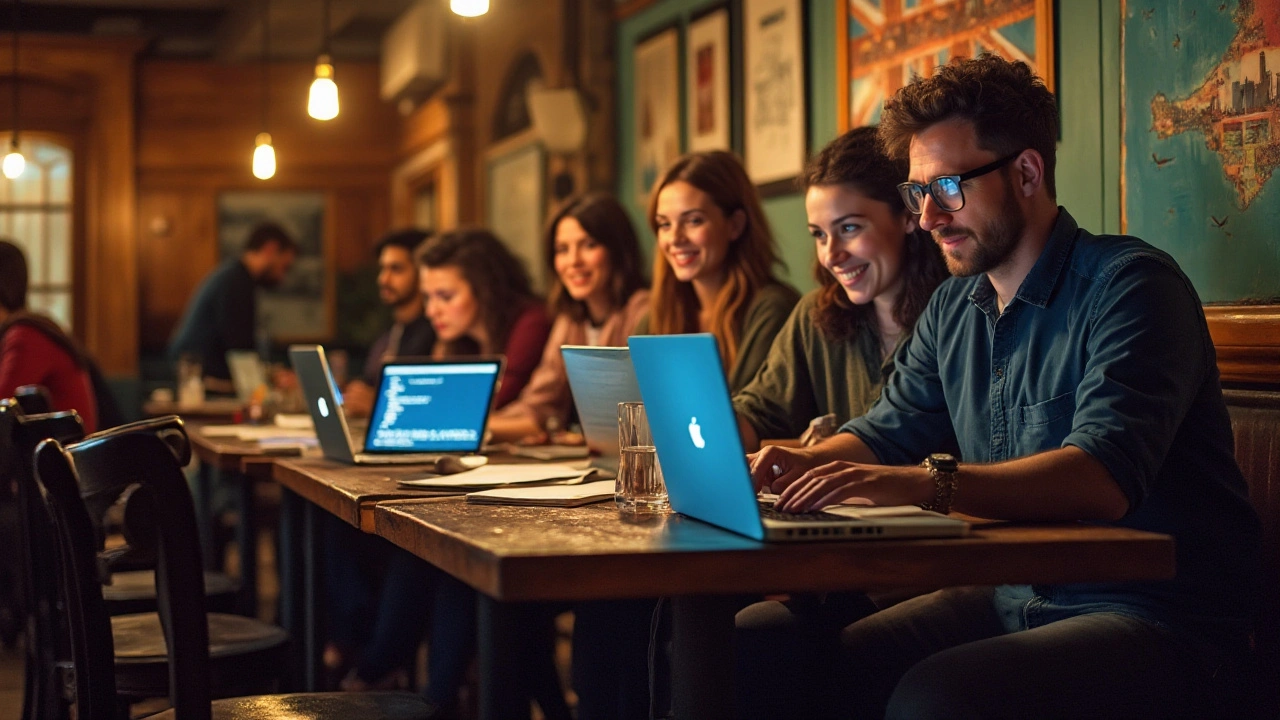
In today's digital age, creating interactive websites is more crucial than ever, and JavaScript stands as a pivotal force in the realm of web development. This programming language, known for its versatility and power, is key to crafting engaging user experiences on the web.
Whether you're just dipping your toes into the world of programming or looking to enhance your existing skill set, this guide offers insights and techniques that can elevate your coding prowess. By understanding the essentials and exploring advanced features, you'll learn how to manipulate key elements to bring your creative visions to life.
As we delve deeper, I'll share a blend of fundamental instructions and intriguing tips that are sure to surprise even seasoned developers. So, let's embark on this journey together and unlock the full potential of your web development projects with JavaScript.
- Introduction to JavaScript
- Basic Programming Concepts
- DOM Manipulation and Events
- Advanced JavaScript Techniques
- Tips for Building User-Friendly Websites
Introduction to JavaScript
JavaScript is a cornerstone technology in modern web development, playing a pivotal role in how websites function and interact with users. This dynamic programming language operates on all major browsers and integrates seamlessly with HTML and CSS, allowing developers to create interactive elements that enhance user engagement. JavaScript was initially developed by Netscape as a means to add dynamic features to static web pages, and over time, it's evolved into one of the most versatile tools in a web developer's toolkit.
The language's syntax is relatively easy to learn, especially if you already have a background in other C-like languages. JavaScript introduces concepts such as variables, functions, and loops that are common in programming, making it a great starting point for beginners. In fact, many programming enthusiasts celebrate JavaScript for its versatility and cross-platform capabilities, since it enables developers to write code that runs consistently across different environments. As a renowned author once said,
"The beauty of JavaScript is its ability to both keep the web simple and make it expressive".
One of JavaScript's most powerful features is its event-driven architecture, which allows developers to specify code that should be executed in response to user actions or other events. This fundamentally changes how websites respond and adapt to the needs of visitors, making the entire user experience more fluent and seamless. While JavaScript is often used to handle validation of form inputs and manage animations, it also stands as the backbone for many modern web applications, providing real-time updates without the need for page reloads—a hallmark of today's digital interactivity.
JavaScript engines, embedded in browsers such as Chrome's V8, Firefox's SpiderMonkey, and Safari's JavaScriptCore, have been optimized to run quickly and efficiently, supporting the rapid execution of complex scripts. The evolution of JavaScript has been driven by the ECMAScript standard, which ensures that new features are robust and maintain backward compatibility. With each edition, JavaScript becomes more powerful, introducing features like classes, modules, and asynchronous programming constructs that rival those found in more traditional languages.
For those embarking on the JavaScript journey, there is a multitude of frameworks and libraries to explore, including the likes of React, Angular, and Vue.js, each serving different purposes and application scenarios. These frameworks significantly streamline the development process, helping to foster robust, efficient, and maintainable codebases. When incorporating such tools, it’s crucial to understand the strengths and challenges they present, and how they fit within your specific project requirements. Aspiring developers must not only focus on mastering the fundamentals but also keeping pace with the lively ecosystem that surrounds JavaScript, as it is indeed an exciting world to be a part of.
Basic Programming Concepts
JavaScript is a language that breathes life into web pages, and understanding its basic programming concepts is where the journey begins. At its core, programming with JavaScript involves variables, functions, and control structures, which form the building blocks for any interactive website. JavaScript, being a dynamically typed language, allows flexibility in declaring variables, using keywords such as var, let, and const. While all three have their place, each serves a specific purpose: var for function-scoped variables, let for block-scoped ones, and const for constants that never change. Properly choosing these keywords is crucial for writing clear and effective code, preventing unpredictable behavior in larger applications.
Functions in JavaScript are not just mere blocks of code but powerful tools that can be customized to fulfill various tasks. They encapsulate logic, making code reusable and organized. One fascinating aspect of JavaScript is its support for first-class functions, meaning they can be passed as arguments, returned from other functions, and assigned to variables. Such flexibility opens doors to higher-order programming, elevating a programmer's ability to manage complex scenarios seamlessly. In fact, Brendan Eich, one of JavaScript's creators, once emphasized the importance of such features in maintaining code simplicity and elegance:
"Always bet on JavaScript."
Control structures like loops and conditional statements are fundamental yet powerful components of JavaScript, aiding in navigating an application’s flow. They determine how the code executes under different conditions, essentially making decisions on behalf of the programmer. JavaScript supports multiple loop types, including for, while, and do...while loops, each suited for distinct iterations. Mastering these can save time and reduce errors, adding precision to how tasks are executed repeatedly. Meanwhile, conditional statements, usually driven by if and switch constructs, empower developers to create versatile and adaptable code that responds well to user interactions and internal data.
Data Types and Structures
Understanding data types is foundational in JavaScript programming. The language supports primitive types like numbers, strings, and booleans, alongside complex structures such as objects and arrays. The distinction is crucial, as operations vary between data types, and mismanagement can lead to bugs. Arrays, for instance, are collections of items stored at contiguous memory locations, enabling multiple values in a single variable. They hold a special place in JavaScript for handling lists of data, supporting various methods for adding, removing, and manipulating elements.
Objects, on the other hand, are perhaps one of the most versatile and essential structures in JavaScript. They provide a blueprint for modeling real-world entities with attributes and behaviors. Using objects, developers manage more significant, interconnected data effortlessly, which plays a pivotal role in application scalability. A fascinating fact: JavaScript objects are inherently linked to each other, forming a prototype chain. This feature allows for inheritance, where an object inherits properties and methods of another, promoting code reusability and efficiency.
The importance of understanding these fundamental concepts cannot be overstated, as they establish a solid foundation for more advanced techniques that transform simple web pages into interactive websites.
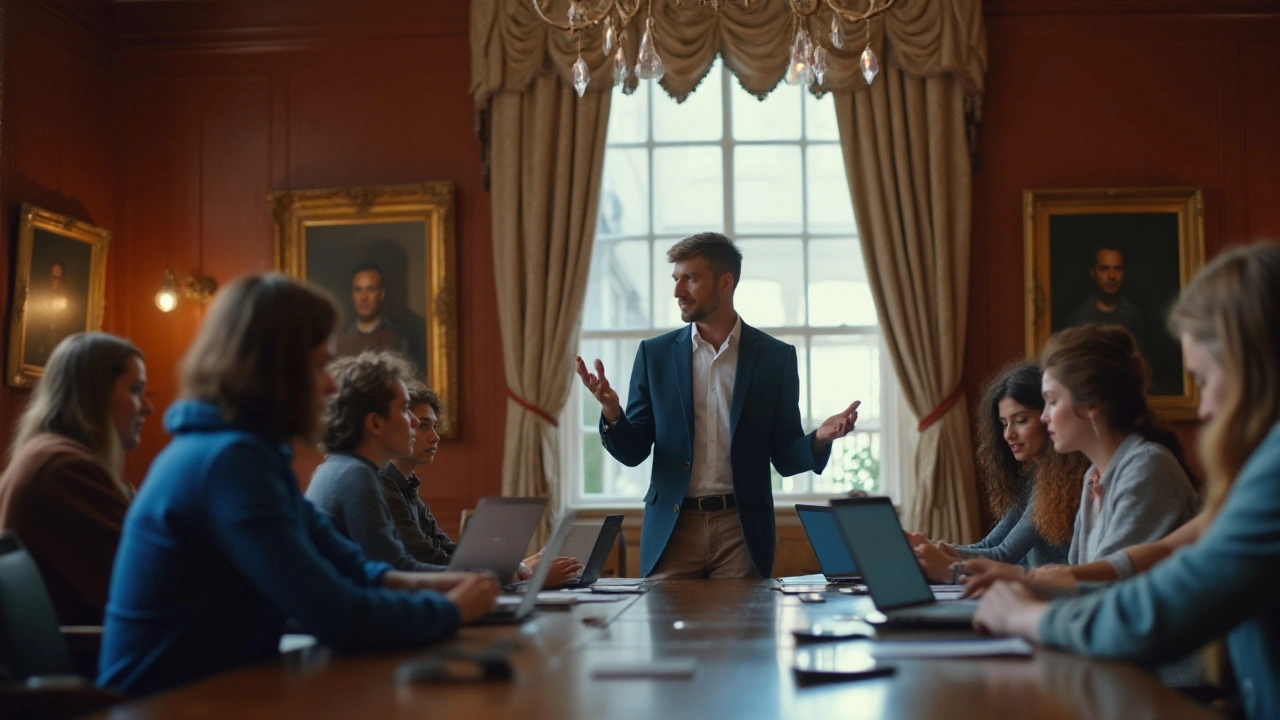
DOM Manipulation and Events
At the heart of creating interactive websites is the Document Object Model, or DOM. Essentially, the DOM is a programming interface that represents the structure of an HTML document in the form of a tree. Each branch of this tree ends in a node, and each node is an object representing a part of the document. This means that every time a webpage loads, the browser creates a model of that page’s structure, which is the DOM. Through the power of JavaScript, we are able to access and manipulate these nodes, allowing us to dynamically change the content and style of a webpage.
JavaScript's ability to manipulate the DOM is crucial for creating pages that feel lively and responsive. For instance, consider simple actions like changing the text inside a button when it's clicked or more complex operations like filtering visible items in a gallery. This kind of interaction is made possible by selecting specific DOM elements, usually through methods like document.getElementById()
or document.querySelector()
, and then altering their properties. One of the keys to effective DOM manipulation is understanding how events work. Events are actions that occur in the browser, and they can be triggered by user interactions such as clicks, key presses, mouse movements, or by the browser itself as elements load or update.
Handling these events appropriately makes your website interactive. An event listener is a procedure or function in a computer program that waits for an event to occur. Using JavaScript, you can set up event listeners for each action you want your site to respond to. For instance, attaching an event listener to a button can look something like this:button.addEventListener('click', function() { alert('Button was clicked!'); });
This small snippet, when implemented, will cause a popup alert to trigger each time the button in question is clicked. By understanding the DOM's structure and how to interact with it through events, you can build components that offer unique and tailored experiences for end-users.
“In JavaScript, DOM is a means to connect web pages to scripts or programming languages by representing the structure of a document; and once you master it, your webpages can become vibrant and dynamic platforms.” — JavaScript Programming Guide
In modern web development, the complexity of DOM manipulation has been significantly reduced thanks to libraries and frameworks like jQuery, React, Angular, and Vue. Each framework provides its own way of handling the DOM, with optimizations and abstractions that make code cleaner and easier to maintain. React, for instance, uses a virtual DOM which is a copy of the actual DOM. When changes are made, the virtual DOM is updated and is then compared to the previous state of the virtual DOM before being applied to the actual DOM. This allows React to make these operations more efficient and significantly speeds up applications.
In the quest to build user-friendly websites, it is crucial to consider performance when performing DOM manipulations. JavaScript engines are pretty fast but manipulating the DOM can be slow if not done correctly. Each time you manipulate the DOM, it can trigger reflows and repaint processes in browsers, which can affect performance. As a rule of thumb, minimize the frequency and the magnitude of DOM manipulations. It can be achieved by batch DOM changes, using document fragments, or by reducing the size and scope of each DOM change. It's fascinating how much you can accomplish once you become adept at wielding the power of the DOM alongside JavaScript events.
Advanced JavaScript Techniques
Diving into advanced JavaScript techniques opens up a realm of possibilities that can transform your interactive websites from functional to phenomenal. Understanding these concepts not only refines your development skills but also enhances the experience of users interacting with your sites. Let’s delve deeper into some of the pivotal advanced concepts that will level up your programming game.
Asynchronous JavaScript and Promises
Asynchronous programming in JavaScript is essential for operations such as API calls or file handling, where you want to ensure that the rest of your code runs smoothly while awaiting certain processes. This is primarily achieved through Promises. With Promises, you can handle tasks like fetching data without bogging down your interface. Imagine a scenario where you're developing a dashboard that must pull real-time data from an online source. Using Promises effectively ensures that users won't experience delays or freezes. This allows your applications to be more responsive and engaging, maintaining the fluidity that users desire in modern web applications.
“The significant problems we face cannot be solved at the same level of thinking we were at when we created them.” - Albert Einstein
ES6 and Beyond
The advent of ECMAScript 6 (ES6) introduced a plethora of features that drastically improved the efficiency of JavaScript code. Arrow functions, template literals, and destructuring assignments are just a few enhancements to consider. These features simplify code, making it more readable and maintainable. Transpilers like Babel allow developers to use the latest syntax while ensuring compatibility across all browsers. This progression means you can take advantage of improved functionality without the headache of cross-browser issues. Crafting efficient code with ES6 can be the key to unlocking rapid and powerful web development.
Function Programming and Higher-Order Functions
Functional programming in JavaScript encourages the creation of predictable and reliable code by using pure functions and avoiding shared state. Higher-order functions, such as map, filter, and reduce, enable developers to manipulate arrays and objects with less code and more clarity. This style of programming is especially useful when handling complex data transformations and ensuring your codebase remains efficient and clean. By embracing the principles of functional programming, you open up a more structured and determined approach to solving problems in JavaScript. Moreover, it allows easier debugging and testing.
Tools for Real-time Applications
Real-time applications have grown in demand, fueled by faster internet speeds and the need for immediate data updates. Technologies like WebSockets and libraries such as Socket.IO provide developers with tools to create applications that respond instantly to user inputs. These tools enable two-way communication between the server and client, ensuring that users get the data they need the moment it’s available. Whether it’s a live chat application, collaborative editing software, or live feed updates, understanding how to implement these tools effectively can significantly enhance interactivity. In fact, according to recent studies, real-time updates can improve user retention rates by up to 50%, providing a compelling reason to invest in these technologies.
Working with APIs
Finally, a vital part of advanced JavaScript is working with APIs. Application Programming Interfaces (APIs) allow different applications to communicate with each other, enabling the integration of services like social media, data analytics, and map functionalities directly into your website. Mastery of Fetch API or Axios library, for instance, allows you to send HTTP requests and easily manage asynchronous data. For developers, this means more robust, versatile applications that can seamlessly integrate a wide array of functionalities, expanding the capabilities of what your web applications can do significantly.
- Master asynchronous programming with Promises and async/await syntax.
- Utilize ES6 features like arrow functions and let/const for cleaner code.
- Apply functional programming principles for predictable and reliable applications.
- Integrate real-time data updates using WebSockets and associated libraries.
- Utilize APIs to enhance application feature sets dramatically.
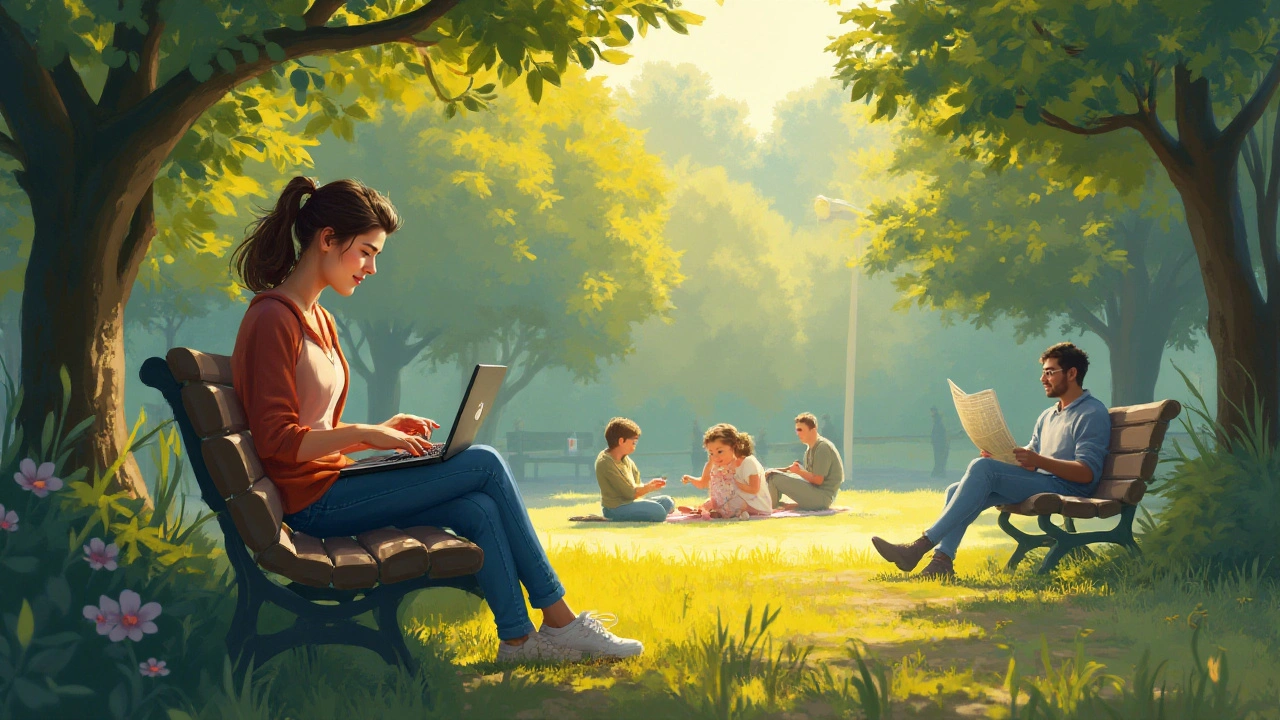
Tips for Building User-Friendly Websites
Creating a user-friendly website is a cornerstone of successful web development. Whether you're a novice or a seasoned developer, understanding how users interact with your site can significantly enhance engagement and satisfaction. It's not just about flashy graphics or trendy designs; at its core, a user-friendly website is intuitive and navigable. Start by keeping the JavaScript functionalities streamlined. This means ensuring that your scripts load efficiently and don't bog down the user's experience. Fast, responsive sites hold the key to retaining visitors. In fact, research from Akamai suggests that a mere two-second delay in webpage load time can increase bounce rates by as much as 103%. So, it's clear—time is of the essence.
Another critical component is accessibility. Ensuring that your site can be used by individuals with disabilities is not only ethical but also widens your audience. Consider employing web development practices that follow established accessibility standards such as the WCAG guidelines. Simple steps like adding alt text for images and ensuring keyboard navigability can make a world of difference. It's not only beneficial for users but enhances SEO performance, as search engines reward accessible sites. Remember, Google's algorithms consider accessibility as a ranking factor, thus improving your site’s visibility in search results. As Timothy Berners-Lee, the inventor of the World Wide Web, once said,
“The power of the Web is in its universality. Access by everyone regardless of disability is an essential aspect.”
Usability Testing
Conducting usability tests is an invaluable practice for web developers. These tests allow you to witness firsthand how users interact with your site, revealing any potential issues in the process. Consider organizing test groups that represent a variety of user demographics to gain a holistic view. Observing users as they navigate your pages will provide insights into the effectiveness of your interactive websites. Noticing a pattern of errors or confusion can point to areas that require a redesign. Structured feedback sessions can also yield invaluable suggestions from users themselves. Document these findings carefully, and always be prepared to iterate on your design. Remember that the key to remaining competitive is continuous improvement based on objective user feedback.
Content Readability
Lastly, content readability is a crucial element that can make or break user engagement on your website. Utilizing language that is clear and straightforward ensures that your content is accessible to the broadest possible audience. This doesn't mean dumbing it down; it means writing in a way that communicates effectively. Pay attention to typography by selecting fonts that are easy to read on screens of all sizes. Additionally, appropriate spacing and contrast between text and background not only enhances readability but boosts the overall design aesthetic. A key tip is to ensure paragraphs don’t overwhelm readers; breaking up text with subheadings and bullet points can make navigation easier. Thus, strong content coupled with thoughtful design practices can significantly enhance the user experience on your interactive website.