Python Tricks: The Ultimate Path to Mastery
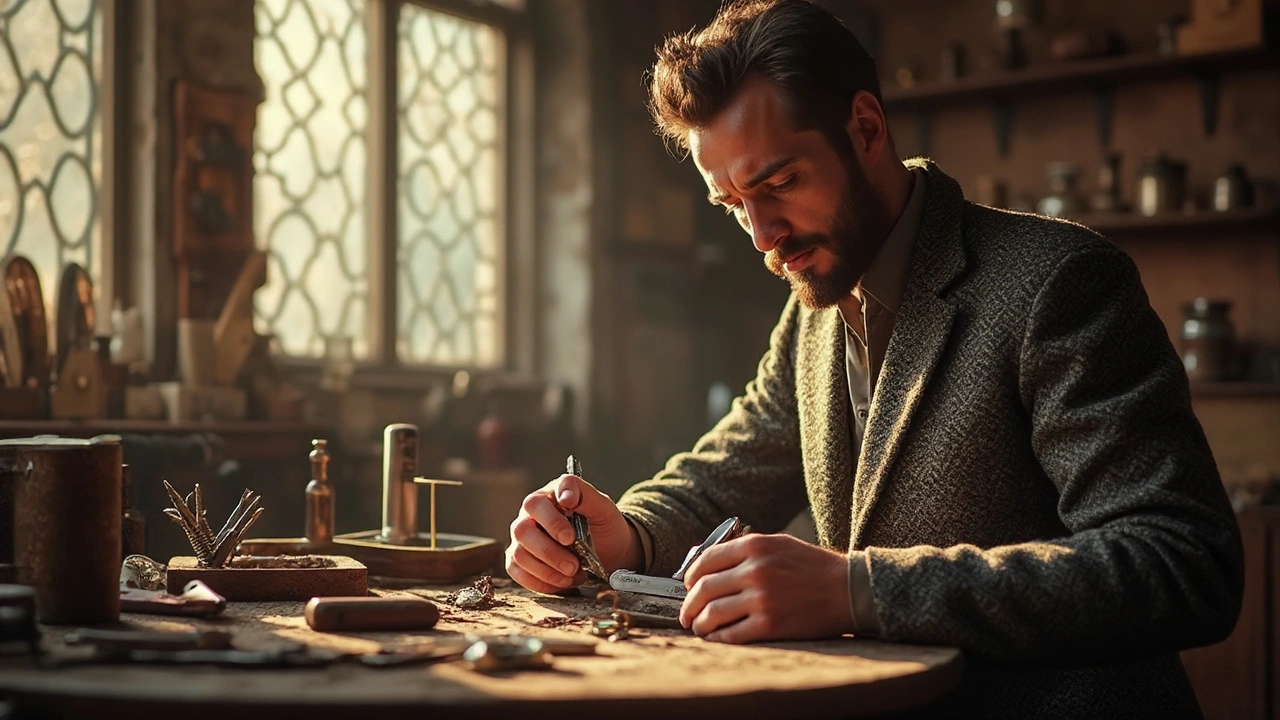
Diving into Python, you might think you're wielding a simple tool, but it's really packed with incredible tricks. If you've ever wondered how to make your code not just do the job but do it elegantly, grab a drink and get comfy, because you're in the right place. Let’s start with something that makes you feel like a coding ninja: list comprehensions. They’re not only a way to write less code but a smart way to up your efficiency game. Imagine turning a ten-line loop into a one-liner. That’s what they can do, and it’s not just about being fancy—it's about clarity and speed.
Now, let's chat about one of Python's coolest features: decorators. These little wrap-around gems make your functions shine by adding functionality without changing their core. Ever needed your function to notify when it’s completed? Or maybe you want to time it? Decorators can be your best friend, making modifications effortlessly and cleanly.
- List Comprehensions for Efficiency
- The Magic of Decorators
- Unpacking with Ease
- Lambda Functions Unleashed
List Comprehensions for Efficiency
List comprehensions in Python are like finding a fast lane on a highway. They let you create lists in a super-efficient way by combining loops and conditionals in just one line. Imagine you have a list of numbers and you need to square each one. With the traditional loop method, you’d probably write several lines of code. But with list comprehensions, you can do it in one fell swoop.
Here's a quick example to show how they work. If you want a list of squares for numbers 1 to 10, it’s simple:
squares = [x**2 for x in range(1, 11)]
Just like that, you've got your list! This isn’t just about making things look pretty; it’s about efficiency. Less code means less room for errors and easier readability.
Conditional Logic
What's even cooler is you can add conditional logic to your list comprehensions. Say you only want the squares of even numbers, you’d tweak it slightly:
even_squares = [x**2 for x in range(1, 11) if x % 2 == 0]
This way, you can filter and transform data in a single, neat expression.
Multiple Inputs
What if you want to create combinations? List comprehensions can handle that too. Here’s how you can shuffle through two lists:
combinations = [(x, y) for x in [1, 2, 3] for y in [4, 5, 6]]
This creates a list of tuples with all possible combinations of numbers from two different lists.
Real World Application
In real-world scenarios like data processing or web scraping, using list comprehensions can dramatically reduce the time your code takes to run and help you keep it tidy. For instance, processing large data sets becomes a breeze, and when paired with other Python tricks, they can significantly improve processing speed.
Here's a quick overview in Python tips:
Feature | Benefit |
---|---|
Loop and Conditional Integration | Simplifies and speeds up code |
In-Line Filtering | Removes unnecessary data quickly |
Compact Syntax | Improves readability and reduces errors |
The Magic of Decorators
If you've ever dreamed of code that adds functions without turning a simple task into a chaos of loops and definitions, welcome to the world of decorators! Think of them as the add-ons that bring more power and flexibility to your functions.
What Exactly Are Decorators?
Decorators are a game-changer in Python. Plainly put, they're functions that modify the behavior of another function. Picture putting your basic latte through a coffee machine that makes it a cappuccino with a simple button press—that's what decorators do for your code!
How They Work
When Python enthusiasts explain decorators, they often use the '@' symbol followed by the decorator function's name, placed above a function definition. This tiny symbol is like a magical wand that wraps additional functionality around the target function.
Here's a super simple example of using a decorator:
@my_decorator
def say_hello():
print('Hello!')
# my_decorator will add something extra to say_hello()
The my_decorator
function will transform the behavior of say_hello()
, altering what happens before or after it runs.
Why Use Decorators?
- Reusability: Once you create a decorator, you can slap it on multiple functions, saving you a ton of unnecessary repetition.
- Simplify Time-consuming Tasks: Imagine you need to log the time taken for numerous functions across your code. A timing decorator makes it a breeze.
- Separation of Concerns: Enhance capabilities without cluttering your primary logic.
Got a script that requires authorization? Decorators are like giving functions their very own VIP entry badges!
Real-world Applications
Decorators are already all around you. If you've ever used Flask for web development, you’ve seen them in action for routing URLs. They also work wonders in scenarios like:
- Implementing access controls in APIs
- Modifying data returns
- Filtration of errors or logging
Ultimately, decorators are about empowering developers to create more modular and maintainable code. Embracing decorators is like adding a versatile superpower to your Python skillset. Why not experiment a bit and see the magic unfold?
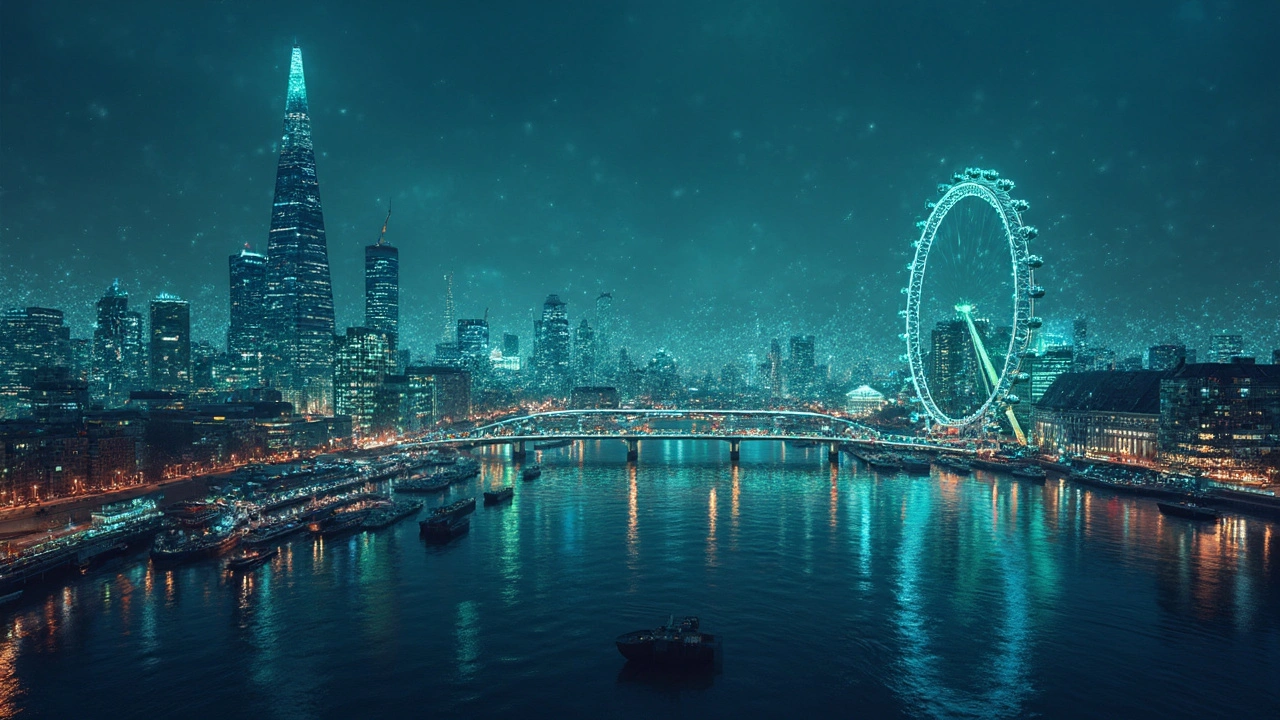
Unpacking with Ease
Ever felt bogged down by the tangled chaos of tuples and lists in Python? Enter Python's unpacking trick, a lifesaver for dealing with sequences. This gem allows you to break down collections into variables effortlessly, making your code cleaner and easier to read.
Let's say you have this list:
numbers = [1, 2, 3]
Typically, you might notice that assigning each number to a variable can get tedious. With unpacking, it’s as easy as:
a, b, c = numbers
Poof! Now a
is 1, b
is 2, and c
is 3. How slick is that?
Beyond Basics: Star Unpacking
Unpacking gets even cooler with the star (*
) operator. This is handy for grabbing multiple elements in one go. Take this example:
team_scores = [90, 85, 80, 95, 92]
Need the first score plus all the rest as a separate list? Easy:
first, *rest = team_scores
Now 'first
' will hold 90
, and 'rest
' will be a list of all the remaining scores. It's like magic, right?
"Python's destructuring is a neat way to slice and dice *data*, making it significantly more manageable." - Brett Cannon, Python core developer.
Unpacking can be a game changer in data analysis when you're dealing with shapes and dimensions. For instance, you can quickly assign values when importing CSV data or processing a batch of inputs in machine learning.
Practical Unpacking Tips
- Avoid using unpacking in functions with too many variables; it makes them hard to debug.
- Unpacking works nicely with dictionaries using
.items()
for quick key-value pair management. - Remember, the number of variables on the left should match or work with the data structure on the right, except when using star syntax.
Unpacking is a fundamental Python trick that makes your coding faster and cleaner. Once you get the hang of it, you'll wonder how you ever survived without it!
Lambda Functions Unleashed
Let’s talk about one of the most interesting features you might come across in Python: lambda functions. They're like those no-name superheroes—they work effectively without even needing a proper name. Think of lambda functions as a way to create small, anonymous functions on the fly.
Why would you use them? Well, if you just need a quick function for a short task, a lambda gets the job done without cluttering your code with function definitions. They're perfect for situations when you're passing functions around as arguments in your code.
So, What’s the Syntax?
The syntax might look strange at first, but it’s pretty straightforward: lambda arguments: expression
. You define them right where you need them. For example:
Imagine you want to sort a list of tuples by the second element. You could use:
sorted_list = sorted(my_list, key=lambda x: x[1])
No huge function definitions here, just a clean one-liner.
When to Use Lambda Functions
- They're really handy for short functions that you don't plan to reuse anywhere else.
- They make your code cleaner, especially if you're using functions as arguments in your higher-order functions like
map()
,filter()
, orsorted()
. - If you're doing data manipulation with Pandas, lambda functions can quickly transform data within
apply()
methods.
However, don't get carried away with them, as they are limited. They can only have a single expression and it’s best if they’re simple enough to understand at a glance. If your function starts getting complex, give it a name and use a def
statement for clarity.
A Glance at Lambda in Practice
Quick example: Say you have a list of words, and you want to filter out the ones that are less than five letters:
words = ['apple', 'bat', 'cat', 'dishwasher', 'elephant']
short_words = filter(lambda x: len(x) < 5, words)
print(list(short_words))
This code will output: ['bat', 'cat']
. Neat, right?