PHP Tricks: Your Path to Better Development Skills
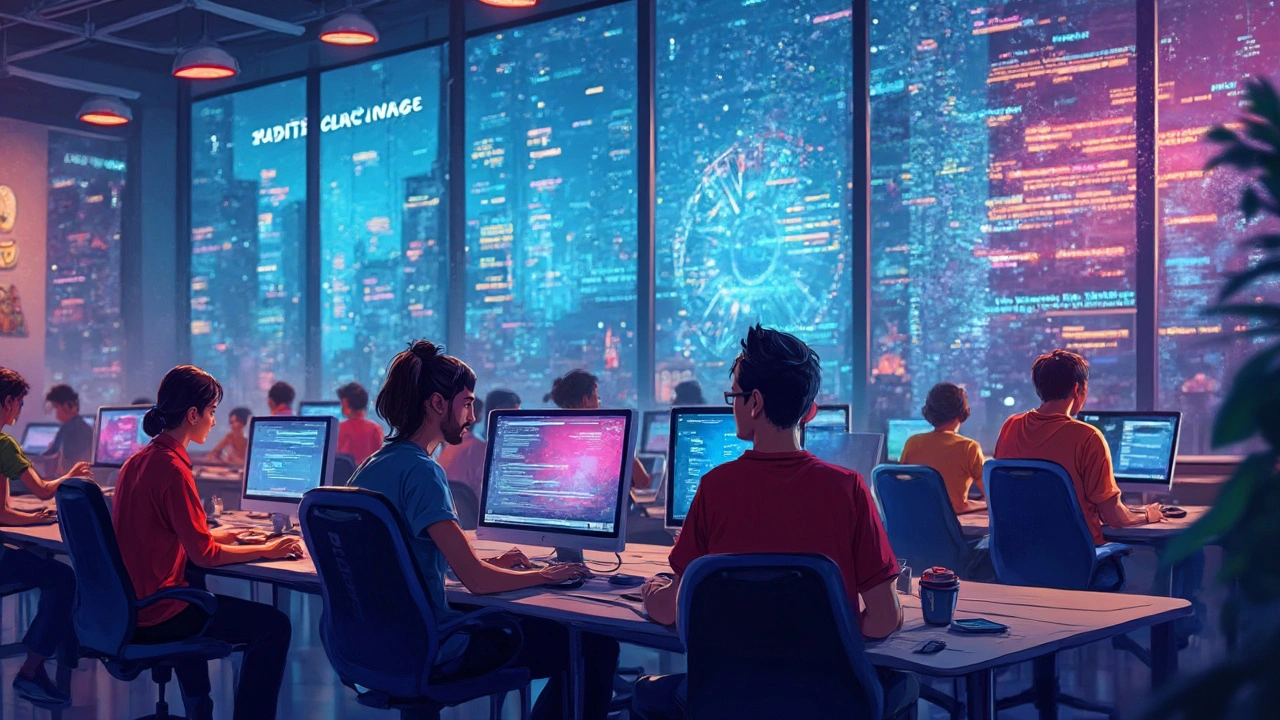
Feeling overwhelmed by PHP? Don't sweat it, we've got some tricks up our sleeves to help you become a PHP whiz in no time. Whether you're scribbling your first lines of code or have managed a few projects, knowing these secrets can save you tons of time and hassle.
First off, it's crucial to get friendly with PHP's core functions. They might not be new, but they're rock-solid reliable and can tackle a lot of tasks with ease. Using built-in functions means less bloat, more speed, and fewer bugs. For example, when handling arrays, native functions like in_array()
or array_merge()
are your best friends.
But let's get real—errors happen. Instead of pulling your hair out, use PHP's error handling techniques like a smarty-pants. Controlling error display, using try-catch blocks, and custom error handlers are techniques that can turn cryptic errors into friendly nudges toward a solution. You'll spend less time guessing and more time coding.
- Understanding Core Functions
- Error Handling and Debugging
- Performance Optimization
- Enhancing Security
Understanding Core Functions
When you start working with PHP, getting a good handle on its core functions is key to boosting your productivity. PHP comes packed with handy built-in functions that streamline your coding experience. Why reinvent the wheel when these already exist? Plus, using these native functions often means more efficient code execution.
Let's talk about strings. Need to mess with them? PHP has your back with functions like strlen()
for length measurement or str_replace()
for swapping out specific words or phrases. These can make string manipulation a breeze.
Array Handling Like a Pro
Arrays are everywhere in coding, so mastering them is essential. In PHP, you’ve got a whole suite of functions dedicated to this task. For instance, array_push()
and array_pop()
add or remove elements from the end of an array, super useful for managing data dynamically.
- in_array() checks if a value exists in an array. Handy for validating user inputs.
- array_merge() combines several arrays into one, making it easier to work with large data sets.
- array_diff() finds the difference between arrays. It’s great for catching changes or discrepancies.
All these functions allow you to manipulate data structures effectively, something that can make or break an application’s performance.
Date and Time Handling
Another essential area is managing dates and times. PHP provides strtotime()
, which converts human-readable date formats into timestamps, and date()
for formatting date outputs. With these, you can handle schedules and timestamps without getting tangled in time zone madness.
Here's a fun fact: PHP's date functions have built-in timezone support, which is indispensable for global applications. So you won’t have to wrestle with time conversions manually.
By knowing these PHP tricks, you're building a solid foundation that lets you handle typical coding scenarios with grace. So, don’t skip over these core functions—dig in and make them your go-to tools.
Error Handling and Debugging
We're diving into the not-so-glamorous but super crucial world of error handling and debugging in PHP. Knowing how to manage errors efficiently can save your project from going haywire and keep your sanity intact. It's all about being prepared and having a plan when things don't go as expected.
Why Error Handling Matters
Ever spent hours scratching your head over a mysterious bug? Proper error handling is your ticket out of that mess. By default, PHP might not give you the best clues about what's going wrong. So switch your configurations to display errors on your development environment. Just remember to flip that switch off when you go live because exposing too many details can be a security risk.
PHP Error Reporting
Let's talk about reporting. You can control what types of errors you want to see using error_reporting()
. It's like a filter for what's important:
E_ALL
: Shows everything, including notices.E_ERROR
: Just the critical stuff.E_WARNING
: Non-fatal issues you might wanna fix.E_NOTICE
: Not urgent but good to know.
Fine-tuning this lets you focus on what really needs attention.
Using Try-Catch Blocks
Think of try-catch blocks as your safety net. If you suspect a chunk of code might misbehave, wrap it up in a try block. Then, catch the potential exception so your program doesn't just halt unexpectedly. It's like giving PHP a heads up to handle hiccups gracefully. Here's a quick example:
try {
// Code that may throw an exception
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "\n";
}
Custom Error Handlers
If you've got unique needs, custom error handlers are your answer. With set_error_handler()
, you can catch and log details in a way that suits your workflow. Consider spinning up a simple logging strategy to write these errors into a file or send alerts for critical problems.
Debugging Tools
Last but not least, don't underestimate the power of good debugging tools. Xdebug, for instance, is a popular choice among developers. It provides in-depth stack traces and can even track function calls, making debugging less like a wild goose chase.
Taking control of error handling in PHP might feel like a chore, but it's smarter in the long run. Your code will thank you, and you'll spend more time building, not fixing.
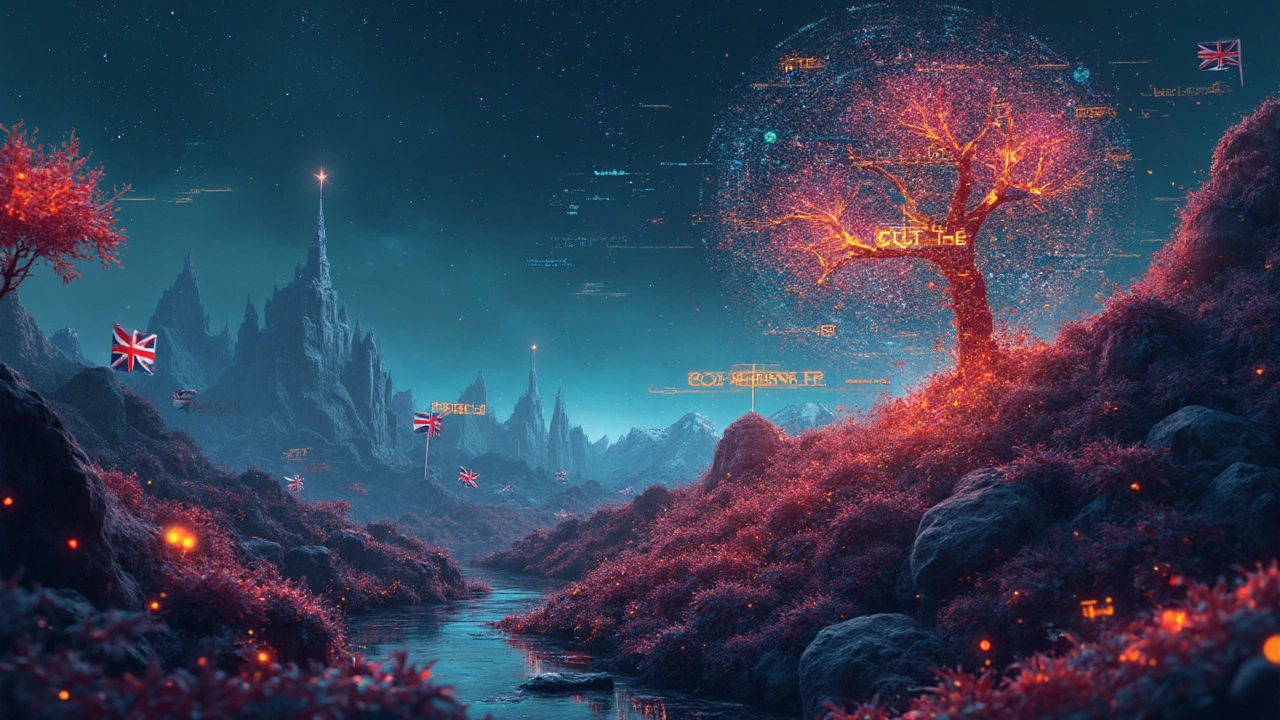
Performance Optimization
Let's face it, we all want our PHP applications to run faster than a speeding bullet. Who's got the time to wait for slow-loading pages, right? When it comes to squeezing every bit of performance from your PHP scripts, there are some go-to moves you can make.
1. Caching is King
If you're not caching, you're missing out on a huge performance booster. Tools like Memcached or Redis can store data in memory for super-fast access. You'll see immediate load-time reductions as your PHP apps won't need to repeat database-intensive operations.
2. Go for the Opcode Cache
Ever heard of an Opcode cache? This nifty feature, like OPcache in PHP, saves precompiled script bytecode in memory. This means your server doesn’t have to compile PHP scripts for each request, trimming down the execution time big time.
3. Trim the Fat: Optimize Your Code
Too many developers overlook code bloat. Optimizing loops, choosing the right data structures, and minimizing function calls can make a substantial difference. Swap those heavy, complex loops with efficient algorithms whenever possible.
4. Avoid Unnecessary Calculations
PHP developers often use calculations that could be done once and stored. If a value doesn’t change with each request, calculate it once and stash it in a variable. Simplicity is your ally.
5. Database Hits: Keep Them Minimal
Direct database queries can slow things down. Make use of batching or fetch data only once instead of hitting the database multiple times. When feasible, pull what you need in a single query and work from there.
Implement these techniques, and you'll feel the speed difference. Your users will thank you, and so will your servers. Performance is paramount, especially when you're fine-tuning those PHP scripts for maximum efficiency.
Enhancing Security
When it comes to web development, security isn’t just a buzzword—it's a necessity. Using PHP wisely can shore up defenses against many common threats. But hey, you need to be on top of your game because hackers sure are.
First off, never trust user input. Whether it's a form, a comment box, or anything else, always validate and sanitize data. Using functions like filter_var()
can save you a lot of headaches, keeping those nasty injections at bay.
Guarding Against SQL Injection
One major threat is SQL injection. An attacker sneaks into your database using unsanitized input fields. Avoid it by using prepared statements and parameterized queries with libraries like PDO or MySQLi. They handle the escaping for you, which means no more runaway SQL queries!
Cross-Site Scripting (XSS)
This sneaky bugger involves bad scripts running on your site, and it's more common than you'd think. Output escaping is your defense. Always escape output with functions like htmlspecialchars()
before rendering data in HTML.
Session Management
Manage your sessions carefully. Attackers love session hijacking. Regenerate session IDs after a person logs in to a new environment and always store sensitive data securely. Use session_regenerate_id()
to confuse unwanted guests.
HTTPS Everywhere
Finally, use HTTPS. Seriously, if you haven't yet, do it now. It encrypts data between your users and servers to keep info safe from prying eyes. Plus, Google favors secure sites, giving you a slight SEO boost.
PHP security isn't rocket science, but it demands vigilance and best practices. By following the above steps, you'll build a solid foundation, reducing risks and letting you sleep a bit better at night.