Python Tricks: The Python Programmer's Secret Weapon
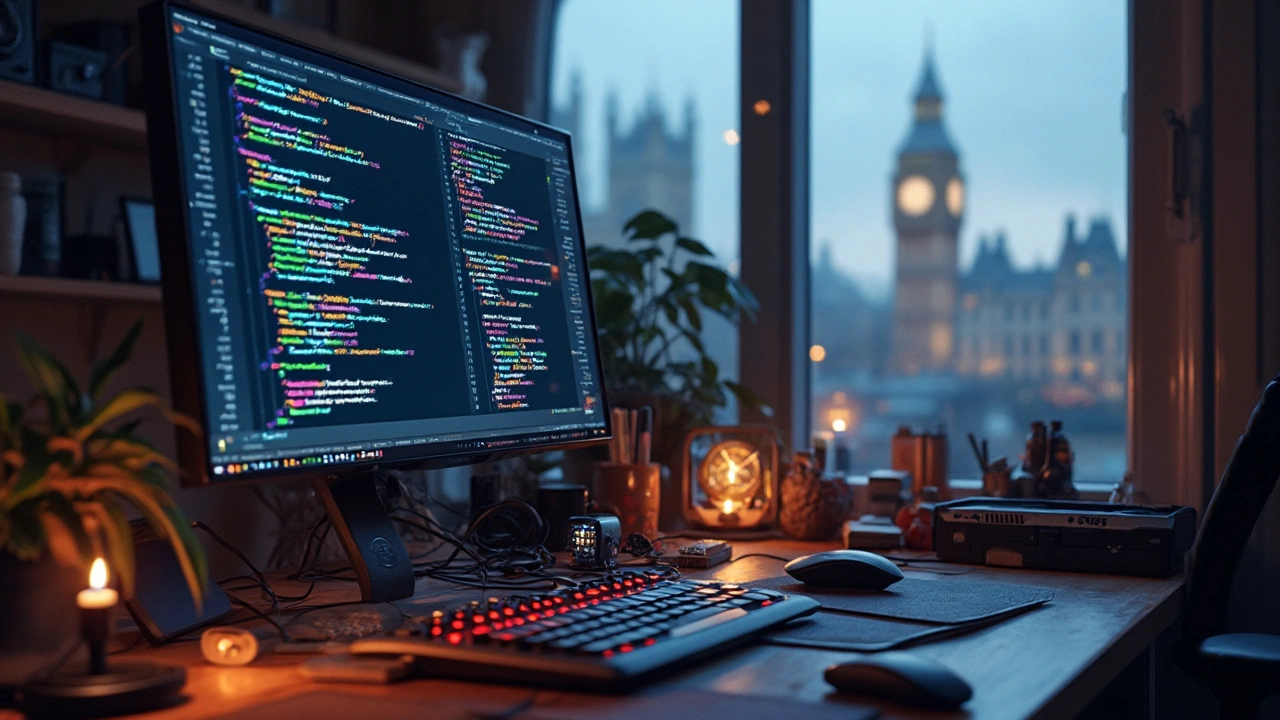
Python is popular for its simplicity and readability, but there's more lurking beneath the surface. It's packed with tricks that can streamline your workflow and boost your coding game! Imagine writing less code to do the same task, all while looking like a coding genius. Sounds cool, right?
Let's start with list comprehensions. They're like a Swiss Army knife for lists. Instead of writing loops the old-school way, you can create lists in a single line! For example, instead of looping through numbers to build a new list, you can just use: [x * x for x in range(10)]
. Bam! You've got a list of squares from 0 to 81 in one line. Simple and tidy.
- List Comprehensions Magic
- Leveraging Built-In Functions
- Understanding Pythonic Habits
- Debugging Like a Pro
List Comprehensions Magic
So, you've been using Python for a while, and you're probably familiar with loops. They're essential but can sometimes feel a bit clunky, right? Well, list comprehensions are here to save the day! They make your code cleaner, faster, and way more efficient. Remember that time you wrote ten lines to create a list? Now you can do it in just one.
The beauty of list comprehensions is that they can express a loop in a single, clear line. In Python, they let you build new lists by applying an expression to each item in an iterable. Their syntax is straightforward: [expression for item in iterable if condition]
. That conditional at the end? Top-notch for filtering items without resorting to multiple lines of code.
Let's dive into an example. Suppose you have a list of numbers, and you want to find all numbers greater than 5 and multiply them by 3. You could do this:
numbers = [1, 6, 8, 3, 9, 4]
result = [x * 3 for x in numbers if x > 5]
Easy peasy! And what if you need to work with nested lists? No sweat—you can include more loops inside a comprehension. Picture this scenario: flatten a list of lists. Through looping, it could get messy. But with a list comprehension, it looks like this:
nested_list = [[1, 2, 3], [4, 5], [6, 7]]
flattened = [item for sublist in nested_list for item in sublist]
That's right, all flattened in just one line. Imagine how neat your code will be. Plus, Python's interpreter is optimized for list comprehensions, making them quicker than standard loops for big datasets.
Using list comprehensions isn't just about saving time—it's about writing Python code that's easy on the eyes. You get a performance boost while keeping your code readable—a double win. So next time you're looping through lists, think about simplifying with Python's **secret weapon**.
Leveraging Built-In Functions
One of the best parts about Python is its awesome collection of built-in functions. These are like ready-to-use tools that let you do a ton of stuff without extra coding. Knowing how to use these can really speed up your work and make your code cleaner and more efficient.
Take Python's built-in function sum()
for instance. If you've got a list of numbers and need to find the total, you don’t need to write a long loop. Just use sum(your_list)
, and voilà, you get the sum in a jiffy. This is especially handy when you’re crunching a lot of numbers.
Another cool function is len()
, which helps you get the number of items in a list, tuple, or even characters in a string. Need the length of a string? Just do len('Hello World')
and you’ll get 11. Easy as pie!
If you're juggling with lists, map()
is a powerhouse function. It applies a function to every item of an iterable, like a list. Let's say you want to double every number in a list. Instead of writing a loop, you can do it in one liner with:map(lambda x: x*2, your_list)
.
Here's a handy table that highlights some of Python's most popular built-in functions:
Function | Purpose |
---|---|
sum() | Returns the sum of all items in an iterable |
len() | Returns the number of items in an object |
sorted() | Returns a sorted list of the specified iterable |
max() | Returns the largest item in an iterable |
min() | Returns the smallest item in an iterable |
With these built-in functions, you’ve got a bunch of shortcuts right at your fingertips. Learning to leverage Python tricks like these can make you a ninja coder in no time.
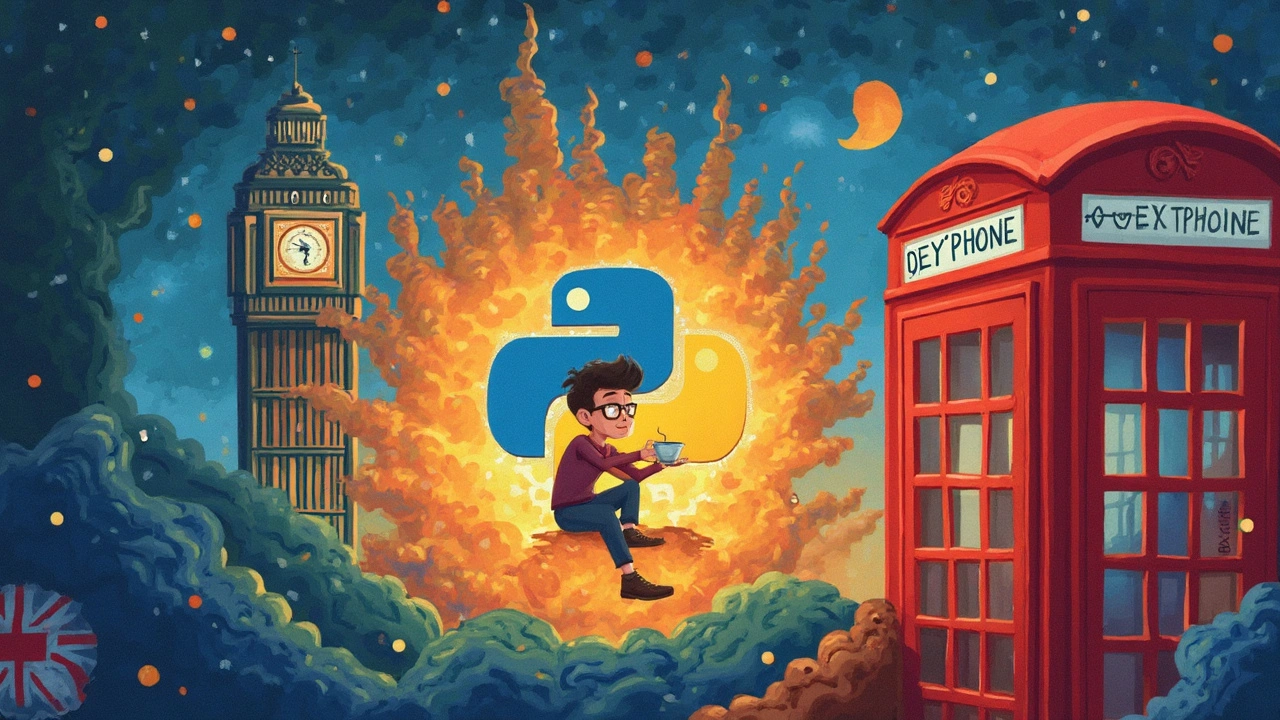
Understanding Pythonic Habits
So, what makes code truly "Pythonic"? It's all about embracing the idioms and styles that the Python community loves. A good place to start is the Zen of Python. You can check it out with a simple command: import this
. Boom! You'll see a list of guiding principles that help developers keep clean and efficient code.
The Zen of Python emphasizes readability and simplicity, which means writing code that's clear to others and easy to understand. A famous quote from Tim Peters, the author of the Zen, goes:
"Beautiful is better than ugly. Explicit is better than implicit. Simple is better than complex."
These are just a few lines from the Zen, but they highlight the essence of writing Python in a way that aligns with its community values.
One Pythonic habit is using built-in functions wherever possible. These gems are usually optimized for performance. Let's say you want to find the sum of a list. Instead of writing your loop, just use: sum(my_list)
. Clean, right?
Another trick is using the Pythonic "EAFP" principle—Easier to Ask for Forgiveness than Permission. Prefer handling exceptions over checking for conditions beforehand. For example, if you're accessing a dictionary, just try to get the value and handle the KeyError
if it pops up.
Here’s a quick table showing some common Python habits:
Traditional Approach | Pythonic Habit |
---|---|
Looping to sum | sum() |
Checking if key exists before getting | Using try-except block |
Using range(len(list)) for iteration | Using enumerate() |
Coding in a Pythonic way also means contributing to maintainability. Clean and clear code isn't just for your benefit; it makes life easier for anyone who reads or maintains your code in the future. So, embrace the habits, and you'll be writing beautifully efficient and effective code in no time!
Debugging Like a Pro
Ah, debugging—every coder's best frenemy. It's part art, part science, and when handled right, it takes you from scratching your head to a eureka moment in no time. Here’s how to channel your inner detective and solve coding mysteries like a pro.
First thing's first: know your errors. Python errors usually tell you exactly where things went off the rails. So, when you spot an error, make a habit of reading the traceback message. It's like a roadmap telling you how you ended up in a ditch.
Want a quick-win? Use Python's pdb
module. This built-in debugger is like having a backstage pass to your code. You can step through the script line by line, inspect variables, and figure out what’s going wrong. To start it, insert import pdb; pdb.set_trace()
right before the trouble point and watch the magic unfold.
Don't underestimate the power of print statements. As old school as it sounds, printing variable values at critical points lets you see what’s happening in real time. It's simple but effective!
Sometimes the bug isn't in your code but in your assumptions. So, always test with fresh eyes. Swap your data, try edge cases, or get a colleague to look over your work. Fresh perspectives often catch what you miss.
Consider using a linting tool. Tools like PyLint give you a heads-up on potential errors and bad practices before they spiral into bigger problems. It's like having a vigilant co-pilot.
Tool | Usefulness |
---|---|
pdb | Step through code and inspect variables |
PyLint | Identify errors and improve code quality |
Lastly, keep your cool. Debugging can be a grind, but every problem solved makes you sharper. Keep chipping away, and soon you'll be debugging like it's second nature.