Coding Tips for Aspiring Data Analysts
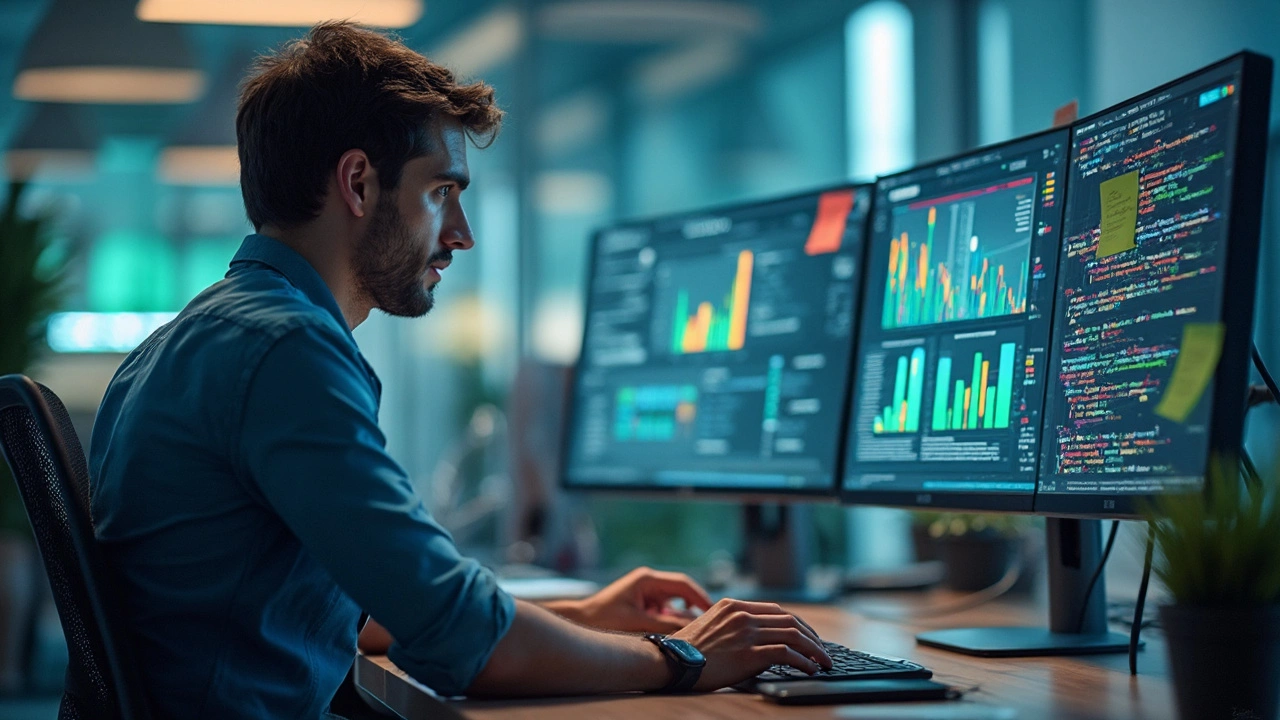
If you’re setting your sights on a career as a data analyst, you’ve probably noticed there’s a lot more to it than just dabbling in spreadsheets. Coding is non-negotiable. But don’t stress—no one writes perfect code at the start. The trick is figuring out what actually matters and where rookies often get tripped up.
First things first: don’t waste months chasing every language out there. Focus on one, like Python or SQL, because they’re everywhere in data jobs. When you know the basics really well, you’ll spend less time looking up error messages and more time actually learning from the data.
Shortcuts and fancy tricks look cool, but clean, clear code is worth so much more—especially when you have to revisit your old projects or explain them to someone else. Simple, well-commented code beats something no one can read every time.
- Start with the Right Programming Language
- Master Data Cleaning Basics
- Leverage Data Libraries and Tools
- Organize and Document Your Work
- Sharpen Your Debugging Skills
- Keep Practicing with Real-World Projects
Start with the Right Programming Language
Choosing the right language is the first real move in your journey as a data analyst. Skip the urge to learn five at once. Get good at one solid option before branching out—that’s how pros build skills you can use on real jobs.
Here’s the deal: Python is basically the industry standard for most aspiring data analysts. You’ll use it for everything—reading data, crunching numbers, even making charts. Plus, major data libraries like Pandas, NumPy, and Matplotlib are all built for Python and play nice together. SQL is just as important if you’re going to be digging through big company databases. Most job posts list either Python or SQL as a must-have skill.
- Python: Best for data cleaning, statistical analysis, quick scripting, and visualization.
- SQL: Dominates when you need to pull and organize data straight from databases—think big tables of customer info at a bank or sales data at an online shop.
- R: Good for heavy statistics and those headed into research, but most business roles stick to Python.
According to a 2024 Stack Overflow Developer Survey, 48% of data professionals say Python is their main language at work. SQL lands right behind—it’s in almost every big data job you’ll find.
Language | Main Use | Popularity Among Data Analysts (%) |
---|---|---|
Python | Data cleaning, analysis, visualization | 48 |
SQL | Database queries, data manipulation | 42 |
R | Statistical analysis, research | 7 |
If you’re unsure where to start, try running a few short Python scripts to see how pandas or matplotlib works with sample data. Or sign up for a free SQL sandbox online and practice basic SELECT queries. It’s way easier to learn with hands-on work than by reading big textbooks.
Master Data Cleaning Basics
Messy data is the number one headache for anyone working with data analyst skills. If you’re working with real datasets, expect missing values, typos, weird date formats, and columns that don’t make any sense. Before crunching numbers or running fancy models, you’ve got to tidy up.
Let’s talk about steps you’ll actually use. In Python, pandas is your best friend for most data cleaning work. Here’s a practical checklist for handling the most common issues:
- Deal with missing data: Use
df.isnull()
to spot gaps anddf.fillna()
ordf.dropna()
to fill or remove them. - Fix inconsistent formats: Convert all dates to a standard format with
pd.to_datetime()
. Standardize text to lowercase withstr.lower()
to avoid duplicates like “USA” and “usa.” - Remove duplicates: Get rid of repeated rows fast with
df.drop_duplicates()
. - Rename columns clearly: Don’t be scared to use
df.rename()
for clear, descriptive column names. You’ll save yourself headaches later. - Check for outliers: Outliers can throw off your analysis. Use
df.describe()
and simple plots to spot anything weird.
Coding tip: Document each cleaning change you make with comments or in a cleaning script. You’ll need to explain where the numbers came from, especially if your boss or a teammate asks.
Don’t underestimate the cleaning stage. According to real tech job postings in 2024, over 70% of data analyst work involves preparing data, not analyzing it. Honing these basics early will put you ahead in coding interviews and in the real world.
Leverage Data Libraries and Tools
If you’re aiming to be a strong data analyst, you have to get comfortable with data libraries and the right set of tools. These aren’t just extra bells and whistles—think of them as your basic toolkit, making things easier, faster, and honestly, way more fun.
Let’s start with Python. It’s popular in the data analyst world mostly because of its libraries. Pandas is your best friend for cleaning, sorting, and analyzing data frames (like Excel on steroids, but with code). NumPy handles mathematical stuff, and Matplotlib or Seaborn are for quick, nice-looking charts. Don’t skip scikit-learn either if you want to get your feet wet with machine learning.
Here’s a quick look at why these Python libraries matter:
Library | Main Use | Why It Matters |
---|---|---|
Pandas | Data Cleaning & Analysis | Super easy filtering, grouping, and wrangling tasks |
NumPy | Math Operations | Handles big numbers and matrices fast |
Matplotlib | Plotting & Visualization | Turns raw data into clear visuals |
Seaborn | Statistical Charts | Makes prettier, more advanced plots |
scikit-learn | Machine Learning | Easy entry to modeling and predictions |
SQL isn’t a library, but it’s a must-have tool. It’s how most companies store data these days, so knowing how to write strong SQL queries (using JOIN, GROUP BY, etc.) is non-negotiable. Even if you’re awesome at Python, employers expect you to be comfortable with SQL for database stuff.
R is another language often used for stats-heavy work. If you’re thinking about getting deep into analytics or research, learning R’s tidyverse (like dplyr and ggplot2) can open more doors. But don’t try to learn it all at once—pick what your target jobs need.
One underrated tip? Get used to Jupyter Notebook or Google Colab. They let you code, test, and share your projects all in one place. You’ll spot bugs easier and can show your work to others (or even future employers) without a hassle.
Last thing: bookmark library documentation. Seriously. Every question you have—someone’s asked it before. The docs are lifesavers when your code suddenly stops working and you’re out of ideas.
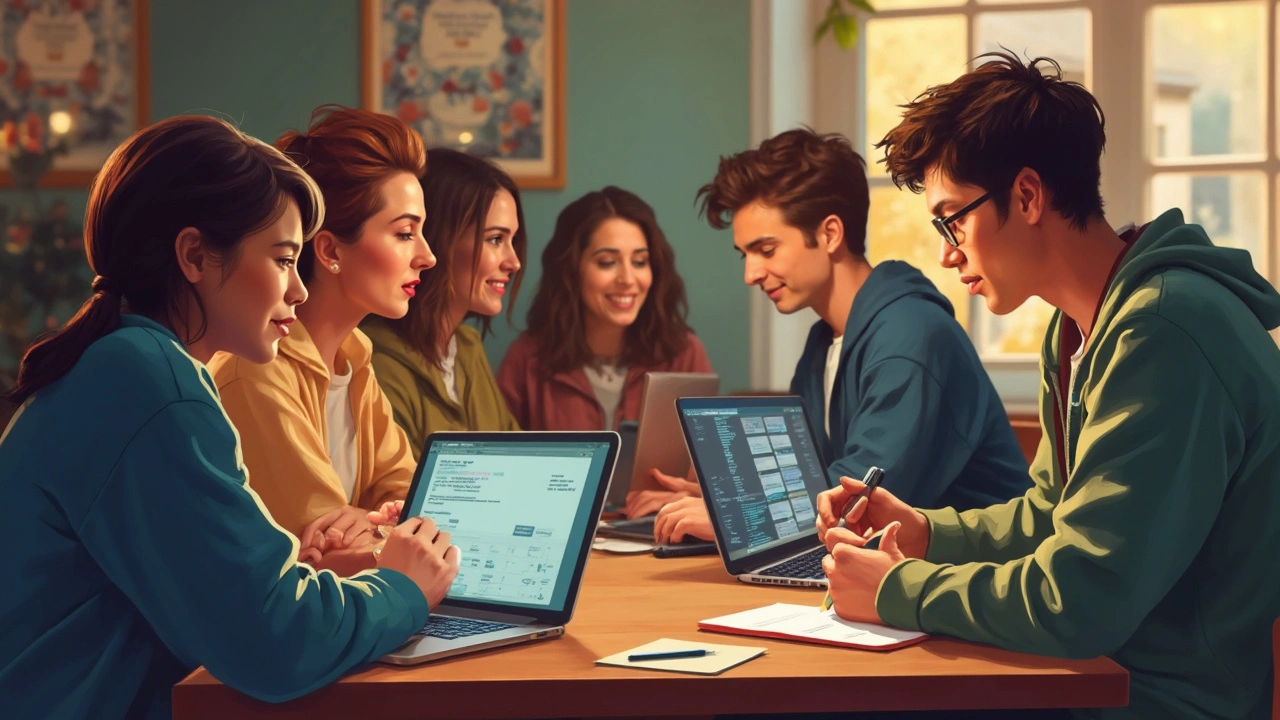
Organize and Document Your Work
No matter how clean your coding looks, chaos behind the scenes will trip you up fast. If you save everything as “final_FINAL_v3.py,” you’re headed straight for headaches. Make folder names, file names, and even variable names as descriptive as possible so you can actually find things later. Even big teams mix things up if organization slips.
Keep your code projects tidy by setting a clear structure from the start. Here’s a super basic layout lots of data analysts use:
- data/ for all your raw and processed datasets
- notebooks/ for quick tests and exploration (Jupyter sounds cool for a reason)
- scripts/ for any Python or SQL code you’ll want to reuse
- results/ for charts, tables, or exported data
- README.md so anyone (including future you) actually knows what’s going on
Don’t skimp on documentation. You might think you’ll remember why you did something, but two months later, it all looks foreign. A quick comment above tricky parts of your Python or SQL code can save hours. And yes, people do check comments—a 2023 survey by Stack Overflow found most developers say a lack of documentation causes way more bugs and wasted time than missing features ever do.
When you write a function, use a docstring to explain what it does, its inputs, and its outputs. If you hand off your code to someone else, this is what helps it make sense. Even a plain text file explaining the steps you followed can help another person (or yourself) retrace the workflow.
Version control is a lifesaver. Even solo projects deserve a simple Git repo, just so you can roll back after a mistake. Quick tip: write meaningful commit messages, not just “fix” or “update.” It sounds picky, but you’ll thank yourself later.
Sharpen Your Debugging Skills
Every data analyst hits bugs—no way around it. What separates rookies from sharp pros is how fast you can spot and squash them. Debugging isn’t just about stopping errors; it’s about understanding what went wrong and why. The faster you figure that out, the faster you get back to business.
Get comfortable with built-in debugging tools in your language. For Python, use print()
statements or dive into pdb
, the Python Debugger. It lets you pause scripts, check variable values, and step through code one line at a time. In SQL, break complex queries into smaller parts and test them bit by bit. Most SQL editors also highlight errors—don’t ignore those red squiggles!
Here’s what helps in real life:
- Read error messages—seriously, half the clues are right there.
- Reproduce the problem with a tiny test case. If your data import explodes, try loading just five rows.
- Check your assumptions: is your column really the data type you think it is?
- Use
assert
statements or built-in “describe” methods to peek at your data. - Keep version history. If you use Jupyter notebooks, you can backtrack to earlier cells or use Git for scripts. Undoing mistakes saves hours.
Data analysts spend up to 80% of their time cleaning and fixing data, according to a 2022 Kaggle survey. That means debugging isn’t just a side skill—it’s the main event. If your code is breaking, it’s usually something simple: typos in column names, wrong file paths, or missing data. You’ll spot these faster with practice.
The pros also know when to ask for help. Post clear, detailed questions on Stack Overflow or share code snippets with friends. Nobody remembers every function or bug fix, but knowing how to look for answers (and where) keeps you moving forward.
Keep Practicing with Real-World Projects
No textbook or tutorial beats hands-on experience, especially when you’re aiming to be a data analyst. Real-world projects force you to wrestle with messy data, find bugs, and dig out insights the way you would on the job. This stuff sticks way better than just following along with example code.
Start by grabbing open datasets—Kaggle, Data.gov, or even Google Dataset Search have tons to pick from. It doesn’t have to be complicated. Analyze something you care about: maybe local weather patterns, sports team stats, or music trends over years. When you’re personally interested, you’ll actually want to solve the problems that pop up.
- Download the dataset and look at what you’ve got. Don’t expect perfect, pretty data. Get comfortable with missing values, weird outliers, and columns with confusing names.
- Use coding tips like version control (Git is great) to track changes. It’s easy to break stuff when experimenting, and this will give you a safety net.
- Document as you go. Real analysts don’t try to memorize every step. Write good notes and keep your code organized—trust me, your future self will thank you.
- Share your work on GitHub or a personal portfolio. Recruiters actually look for this. Plus, real feedback from the data community can fast-track your progress way more than working in isolation.
Mess around with Python libraries like Pandas and NumPy, and try linking your data with SQL databases to mimic real job scenarios. If you hit a wall, Google the error message. You’d be surprised—almost every problem has been solved and explained somewhere online.
Project Idea | Skills Learned | Difficulty |
---|---|---|
Analyze city bike sharing data | Data cleaning, visualization, SQL joins | Beginner |
Scrape and study stock price trends | Python, data wrangling, time-series | Intermediate |
Build a movie recommendation tool | Pandas, algorithms, presentation | Challenging |
The more real-world projects you can tackle, the faster your skills and confidence grow. Showing these off is one of the best ways to stand out in the competitive world of data analysis. So, don’t be shy—get your hands messy and learn by doing!