Python Tricks: The Essential Guide to Python Programming
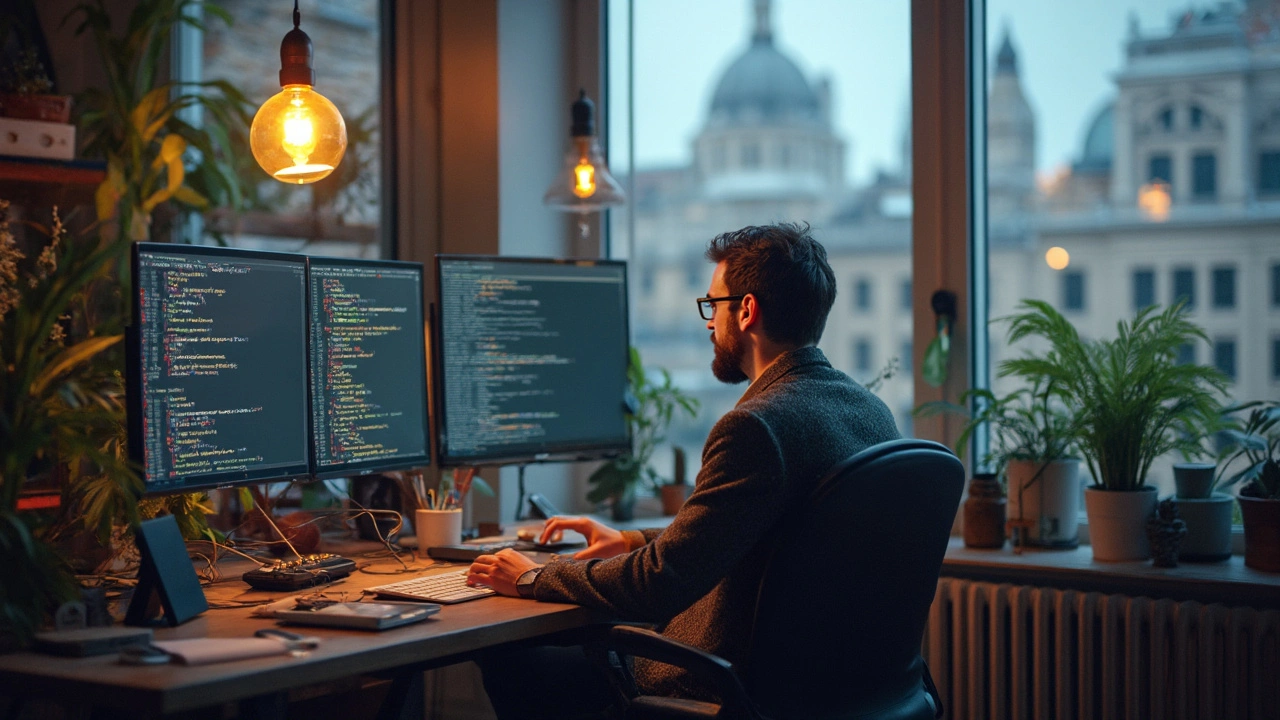
If you’re diving into the world of coding, Python is your new best friend. It's not just popular; it's a robust and versatile language that's great for beginners and pros alike. Why? It's easy to read and write, which means you can focus more on solving problems and less on complex syntax.
But let’s face it, even with Python's simplicity, we sometimes hit those pesky roadblocks that can throw us off track. That's where knowing a few helpful tricks can save loads of time and frustration. Imagine quickly spotting the bug in your code or speeding up your functions with just a tweak or two! Interested? Stick around, we’ve got some cool hacks to share.
- Why Python Rocks
- Time-Saving Shortcuts
- Mastering Debugging
- Libraries You Should Know
- Advanced Tips You Can't Miss
Why Python Rocks
Python might just be the coolest language out there for both beginners and experienced coders. One of the absolute best things about Python programming is its simplicity. You don't need to write tons of code to get things done. The syntax is straightforward and almost reads like English, which means you can focus on solving the problem instead of wrestling with the language.
And it’s not just about ease of use. Python is incredibly versatile. You can use it for web development; think of frameworks like Django or Flask when building amazing web apps. If you're into data science, libraries like NumPy and pandas let you crunch numbers at lightning speed.
But wait, there’s more! Python is also a darling in the world of automation. With just a few lines, you can automate boring, repetitive tasks. Imagine setting up scripts that automatically rename files or scrape data from the web. It's like having a useful little robot do the tedious stuff for you!
- Easy to Learn and Use: Its clean and simple syntax is a big hit among new learners.
- Wide Range of Applications: From web apps to AI, it's everywhere.
- Huge Community: Tons of support and a wealth of pre-existing libraries to make your life easier.
As for popularity, Python just keeps climbing the charts. It's consistently ranked as a top language year after year. Just check out the annual reports from the TIOBE index, and you'll see Python often scooping top honors. It's safe to say, anyone into Python tricks is diving into something rewarding!
Time-Saving Shortcuts
When you're knee-deep in Python programming, every second counts. That’s why knowing some handy shortcuts can make a huge difference. Trust me, these aren't just about saving time—they're about boosting your productivity and keeping you in the zone.
One of the best shortcuts is using list comprehensions. If you're not using them, you’re missing out. They let you create lists in a single, readable line of code, keeping things neat and tidy. Say goodbye to multi-line loops and hello to cleaner code!
Ever heard of the 'Enumerate' function? It's a lifesaver when you need to loop through a list but also keep track of the index. Instead of going through the hassle of setting up both a count variable and a for loop, ‘Enumerate’ does it all for you in one swift motion.
- List Comprehensions: Transform loops into a simple one-liner.
- Enumerate: Loop over indexed lists without extra fuss.
- Zip: Iterate over two or more lists at the same time effectively.
And let's not forget the ‘Zip’ function. If you’ve ever had to loop over multiple lists at once, ‘Zip’ will become your go-to. It groups elements from each list into tuples, helping you work with them side by side.
If you're into stats, take a look at this handy table showing how much time these shortcuts can save:
Task | Time Without Shortcuts (seconds) | Time With Shortcuts (seconds) |
---|---|---|
Creating a list | 5 | 2 |
Indexed looping | 7 | 3 |
Multi-list iteration | 8 | 3 |
Using just these few tricks, you can already start cutting down on your coding time significantly. So, why not give them a try during your next Python programming session? You'll be whizzing through your projects in no time.
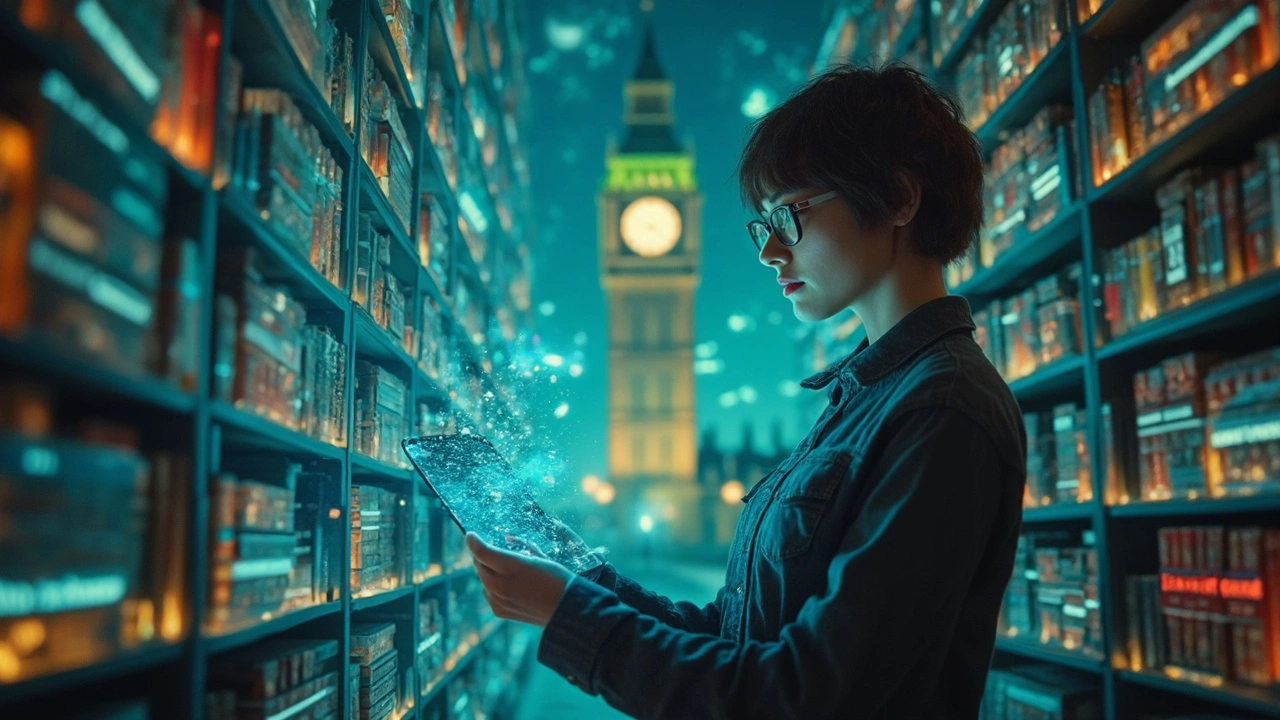
Mastering Debugging
Got a bug that just won't quit? Don't worry, it happens to everyone. Debugging can feel like trying to find a needle in a haystack, but with some smart tactics, you can track down even the sneakiest of bugs. A strong debugger tool integrated within most IDEs can be your best ally when it comes to Python programming.
Start with the trusty Python debugger: pdb
. It's a built-in module that comes packed with most Python installations, ready for action. You can set breakpoints, step through your code, and evaluate expressions. Here’s a simple way to use it:
- First, insert
import pdb; pdb.set_trace()
at the point in your code where you need to pause and look around. - Run your program, and when it hits the breakpoint, it enters interactive debugging mode.
- You can now inspect variables, execute expressions, and truly dig into what’s going wrong.
An even easier option for beginners is the IDE’s built-in debugger. Just point and click your way through lines of code. Most popular IDEs have this feature and provide a visual representation of the debugging process.
Logging is another powerful technique. Instead of guessing what's happening inside your code, use the logging
library to print out messages detailing your program's flow. This way, you can follow the program’s journey without stopping it.
Finally, keep an eye on common syntax errors. A missing colon here or an extra space there can throw the whole thing off. Consider using linters like pylint
or flake8
to catch these little gremlins before they cause problems.
According to a 2023 study by Code Institute, developers save about 30% of their time by effectively using debugging tools. That’s enough extra time to start a new project or finally get that coffee you've been craving!
Libraries You Should Know
Diving into Python programming without leveraging its robust libraries is like owning a fancy toolbox and only using a screwdriver. Whether you're a newbie or a seasoned coder, these libraries can seriously turbocharge your productivity.
First up, let's talk about NumPy. This library is the backbone for numerical computations in Python. It's essential if you're working with arrays and matrices. Need to perform operations faster and with less code? NumPy has got you covered.
Next, check out Pandas. If you're dealing with data, this one is a must-have. It’s fantastic for data manipulation and analysis. Think of it as your Swiss Army knife for dataframes, allowing you to clean, transform, and analyze with ease. Plus, it works hand-in-hand with data files like CSVs or Excel spreadsheets.
Got some plots to draw? Matplotlib and its buddy Seaborn are your go-tos. Matplotlib is the veteran in data visualization in Python, while Seaborn sits on top and makes pretty statistical graphics that'll impress any audience.
If you’re venturing into the world of deep learning or AI, TensorFlow and PyTorch are among the top contenders. TensorFlow, developed by the Google Brain team, is perfect for working with machine learning models. PyTorch, on the other hand, is loved for its simplicity and flexibility, particularly when just starting out or working on research projects.
- Requests: A lifesaver for making HTTP requests simpler and more human-friendly.
- SciPy: Built on NumPy, it provides more advanced operations; ideal for scientific and technical computing.
- BeautifulSoup: When it comes to web scraping, this is your tool of choice. Extract data from HTML and XML files without a hitch.
Knowing these libraries can make a world of difference in how you tackle problems and build solutions. So, which one will you use first in your next project?
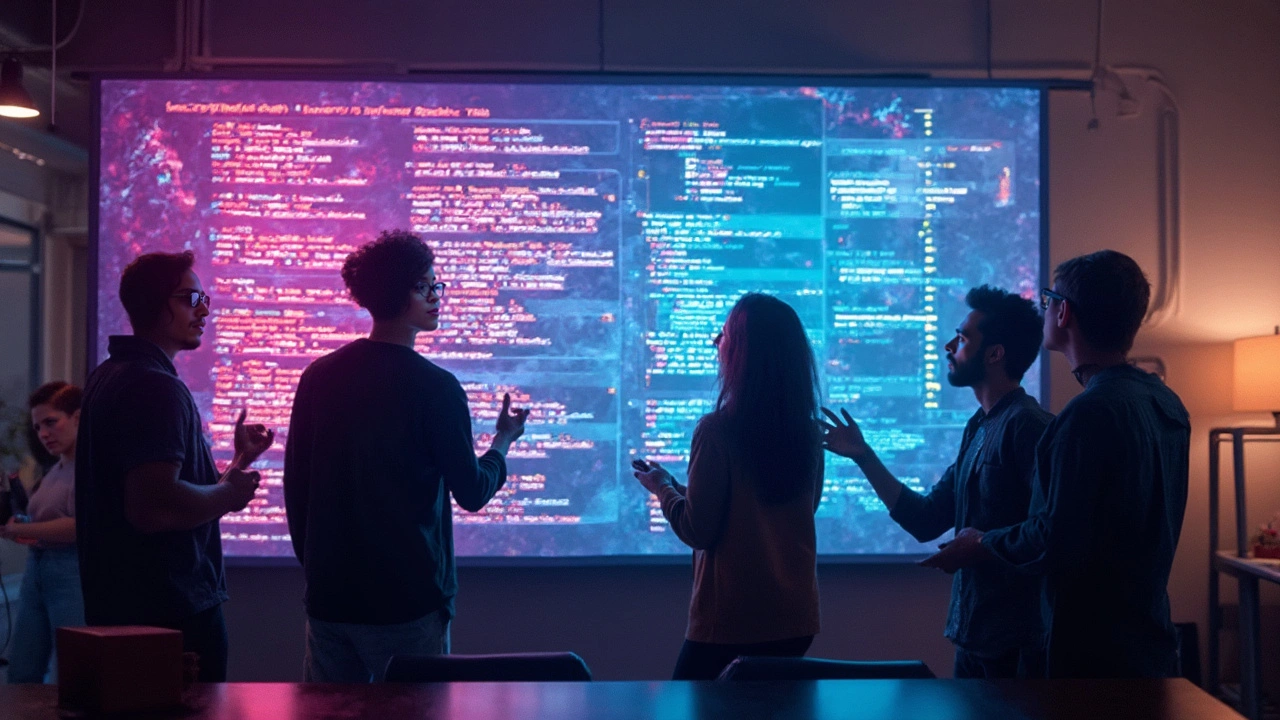
Advanced Tips You Can't Miss
Ready to take your Python programming skills up a notch? Great, because we’re diving into some advanced techniques that will save you time and maybe even impress your peers. Let’s get right into it.
Ever heard of generators? They're like a secret weapon in Python that can save you memory and boost performance. Instead of storing everything in memory, generators let you loop over data on-the-go. This is a game-changer when you're dealing with large datasets. Just use the yield
keyword in a function and watch the magic happen.
Now, let's talk about list comprehensions. They’re not just a way to make cleaner syntax; they can also speed up your code. If you’re looking to create lists in just one line, this is the go-to trick. Something like [x*2 for x in range(10)]
is not just concise but efficient.
How about some quick tips on handling JSON data with Python? The json module is your buddy for parsing and converting JSON. Use json.loads()
to decode JSON strings and json.dumps()
to encode JSON objects back to strings. It’s a breeze once you get the hang of it.
Do you want even more bang for your buck? Then decorators in Python will blow your mind! They let you modify the behavior of a function or class. Think of them as a powerful way to add custom functionality without changing the entire function code.
Here's a quick tip on time measurement in Python to optimize performance. Use the timeit
module. Just wrap the code you’re testing in a function, and timeit.timeit()
can tell you exactly how fast it runs. This helps in comparing different approaches and choosing the best one.
Finally, don't underestimate the power of Python's built-in functions. Knowing when to use functions like map()
, filter()
, or reduce()
can drastically reduce your code clutter and enhance speed.
Remember, mastering these Python tricks isn't just about knowing them, but knowing when to use them. The more you experiment, the more intuitive they become. Give them a try in your next project!