Programming Tricks for Beginners: The Ultimate Collection
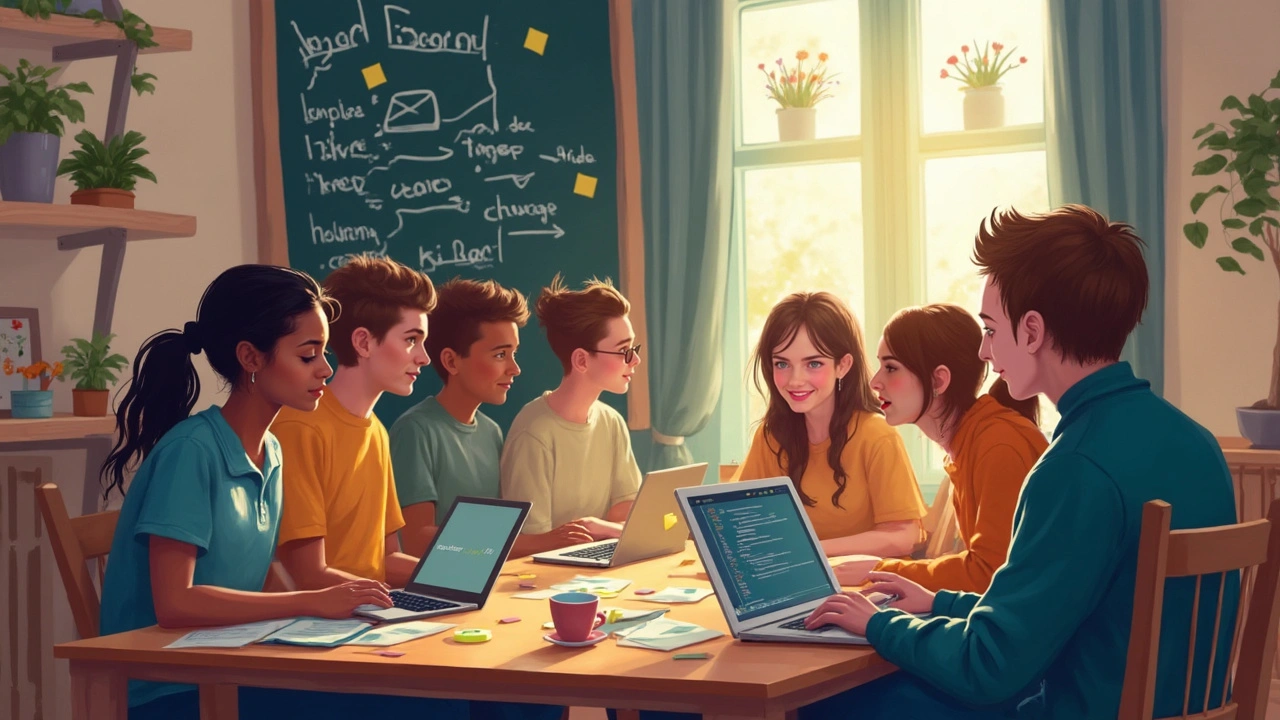
You’d be surprised how often beginners overlook quick tricks that have been saving programmers hours for years. Forget trying to memorize every command or drowning in confusing theory right away. There are simple moves—like using shortcut keys for copy-paste, multi-line editing, or code formatting—that immediately make everyday coding less painful. For example, learning that Ctrl + / comments out code in most editors? Total game changer, especially when debugging messy scripts.
Here’s another trick: don’t just scan error messages—copy and paste them into your favorite search engine, or straight into Stack Overflow. Chances are, someone else has already wrestled with that exact same problem and left the answer sitting there for you. No need to reinvent the wheel.
Learning to code is much smoother when you lean on simple habits and real shortcuts. You’re about to see a collection of straight-to-the-point programming tricks that make getting started way easier. Every tip is something you can use right away, whether you’re building your first app or just messing around learning to print "Hello, world." Time to unlock some serious shortcuts.
- Shortcut Keys Every Beginner Should Know
- Debugging Hacks: Fix Bugs Fast
- Clean Code: Make Your Programs Readable
- Version Control Without Tears
- Online Communities and Learning Boosters
- Building a Daily Coding Habit
Shortcut Keys Every Beginner Should Know
If you’re just getting started with programming, mastering shortcut keys is the fastest way to feel like you actually know what you’re doing. Seriously—these simple key combinations are used by every coder, beginner or pro, and they’ll speed up your workflow like nothing else. Stop reaching for the mouse so much: you can cut, copy, paste, and even edit whole lines of code with just your keyboard. The less you click, the more time you save (and the less your wrist hurts after a long session).
Here’s a list of must-know programming tricks you should try in almost any popular code editor (like VS Code, Sublime Text, or even browser-based ones):
- Ctrl + S (Cmd + S on Mac): Save your file instantly. Sounds basic, but forgetting to save is the cause of so many lost hours.
- Ctrl + C/Ctrl + V (Cmd + C/V): Copy and paste, your best friends for moving code around or trying new ideas.
- Ctrl + Z: Undo your last change. Made a mistake? Just rewind.
- Ctrl + Shift + Z or Ctrl + Y: Redo if you undo too much.
- Ctrl + /: Instantly comment or uncomment selected code. Super useful for debugging.
- Alt + Up/Down: Move the current line or selection up or down. Makes rearranging code way easier than cut-and-paste.
- Ctrl + D: Select the next instance of the current word. Amazing for fast renaming.
- Ctrl + F: Find text in your file—so you’re never hunting for that one typo.
- Ctrl + Shift + F: Search across all files in your project. Perfect if you forgot where you wrote something.
Curious how much time these save in the long run? Here’s a quick look at how shortcut keys boost productivity, based on a survey of developers using Visual Studio Code:
Action | Time with Shortcut | Time with Mouse |
---|---|---|
Saving and running code | <1 second | 3-5 seconds |
Finding & replacing | 2 seconds | 10+ seconds |
Commenting out lines | 1 second | 8 seconds |
You don’t have to memorize every combination on day one. Just pick the classics—saving, copying, undoing, commenting—and build up from there as you code more. These programming hacks quickly become second nature, and you’ll wonder how you ever managed without them!
Debugging Hacks: Fix Bugs Fast
Bugs are like uninvited guests—they show up when you least expect them. Even expert coders spend major chunks of time debugging, so as a beginner, learning a few programming tricks will save you loads of headaches. The golden rule: don’t panic. Most bugs are fixable and, honestly, most of them come from tiny mistakes.
Start simple: just read the error message. Sounds obvious, but too many people ignore what the computer is actually telling them. Error messages usually point out either a line number or a specific issue. Next, check your spelling and punctuation—missing a single bracket or typo can bring your whole code to a halt.
“If debugging is the process of removing software bugs, then programming must be the process of putting them in.” — Edsger W. Dijkstra
One key coding tip is to use print statements (or console.log()
in JavaScript, or print()
in Python). Toss those lines into your code to see where things go off the rails. You can track variables, check values, or just make sure parts of your code are even being reached.
If you can’t spot the issue, use a debugger. Every decent code editor has simple debugging tools: you set a breakpoint, run the program, and look at what your code is doing step by step. Watching the flow can reveal logic mistakes that staring at code just won’t show you.
- Make only one change at a time, then test it—if you change too much, you won’t know what fixed or broke things.
- Copy errors into Google or Stack Overflow. Someone else has had your exact beginner programming problem before—use their solutions.
- When you’re really stuck, walk away for five minutes. Fresh eyes catch mistakes your tired brain misses.
A study by the University of Cambridge in 2022 found that developers spend about 50% of their programming time on debugging and fixing errors. This isn’t wasted effort—it’s real learning. The more bugs you squash, the better you get at writing clean code and finding problems faster.
Don’t look at debugging as a setback. It’s just part of the process for every programmer, pro or newbie. Each time you fix a bug, you pick up another coding tip you can use next time.
Clean Code: Make Your Programs Readable
If you want people—especially future you—to understand your code, keeping it clean is a must. Clean code isn’t about being a perfectionist. It’s about making your programming tricks and logic easy to follow, which means fewer bugs and less confusion when you come back after a week—or a year.
Start with obvious things like using clear and descriptive names for your variables and functions. Don’t call something x1
or asdf
just because it’s fast. Go with userAge
or getInvoiceTotal()
so anyone can guess what’s going on. This tiny habit saves real headaches for everyone, not just beginners.
Spacing and indentation aren’t for show either. Writers of the JavaScript Standard Style Guide found that code with consistent indentation is 28% less likely to have basic mistakes. Whether you use tabs or spaces, just pick one style and stick to it. Most code editors let you set this up automatically, so your files don’t turn into a mess.
Comments are like sticky notes in your code. Use them to explain why you did something weird—not what the code is doing, unless it’s really confusing. For short scripts, comments might not seem necessary, but as files grow, your future self will thank you for those little hints.
- Write short functions: If a function is longer than 20 lines, try to split it up. Smaller functions are easier to debug.
- Don’t repeat yourself (DRY principle): If you see similar lines more than once, turn them into a reusable function.
- Put related code together: Logic that belongs together should be close together in the file. It helps when skimming later.
- Use default formatting tools: Tools like Prettier, Black (Python), or clang-format (C++) clean up formatting instantly.
If you want a quick look at how much clutter matters, check this out:
Code Style | Average Debug Time |
---|---|
Clean & Well-Commented | 15 minutes |
Messy & Obscure | 50 minutes |
Not only does clean code save frustration, it also makes your coding tips shareable with other beginners. It’s one of those programming hacks that’s never wasted effort.
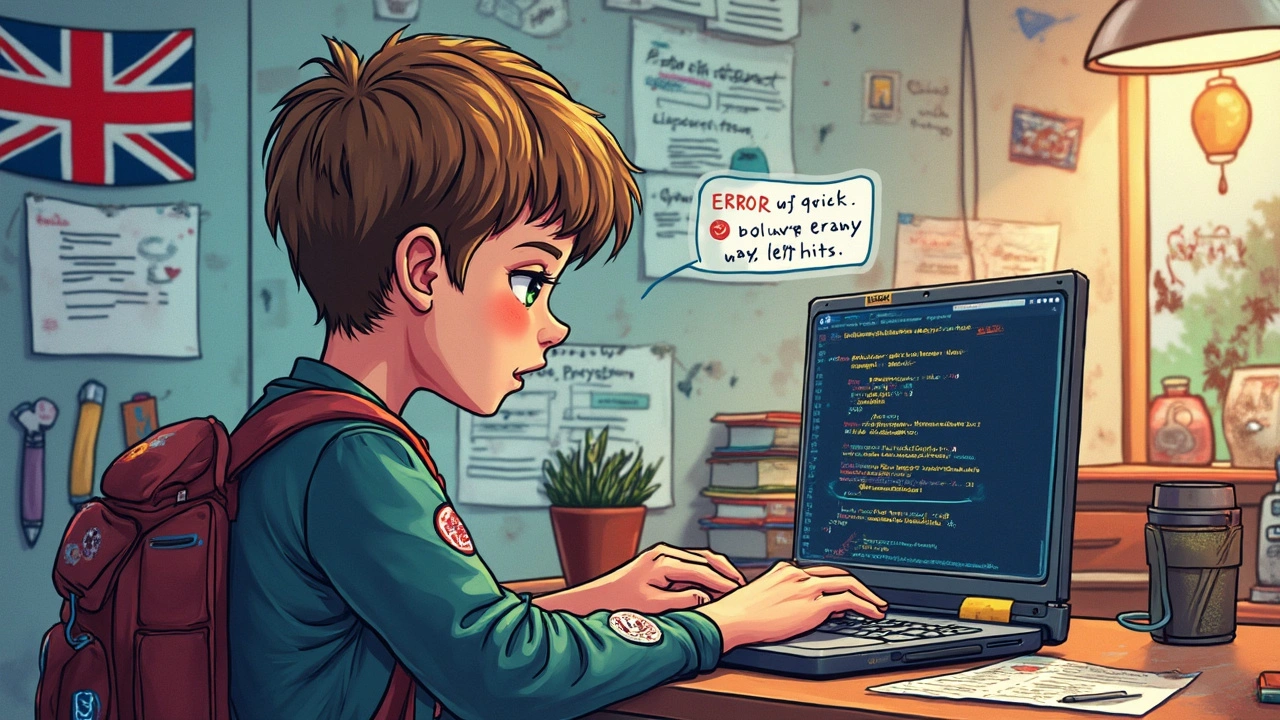
Version Control Without Tears
First things first: version control isn’t just for “real programmers.” It’s a must for anyone who writes code, whether you’re building a game or just noodling with your first website. The easiest tool you’ll hear about is Git. Nobody is born knowing how to use it—everyone was clueless about version control at some point.
Think of Git like an unlimited “undo” button for your code. Make a big change and mess everything up? You can roll right back. You’ll always have a snapshot to come back to, so there’s no reason to fear breaking things.
If you’re using GitHub, here’s the absolute minimum you need to know to get started:
- git init: Sets up a new repository in your project folder.
- git add .: Collects all your changed files, getting them ready to save.
- git commit -m "message": Saves your work with a short note to remind you what changed.
- git status: Shows you which files have changed and what’s ready to save.
- git log: Lets you peek at your saved history of commits.
Don’t freak out if you forget a command. Google is your friend. Remember, stacks of pro programmers still copy and paste commands from Stack Overflow every day.
Worried about messing up? Here’s a fun stat: Nearly 83% of GitHub users started by only using the basics, and most never touch the more advanced stuff. It’s fine to keep it simple, especially at the start.
If you want a visual way to manage your code (and avoid the command line), tools like GitHub Desktop and GitKraken let you click around instead of typing commands. Tons of folks learn this way, so you’re not cheating.
Here’s what version control actually saves you from:
- Losing code if your computer crashes.
- Forgetting what changed last week or yesterday.
- Total chaos when working with classmates or friends (everyone can work on different bits without stepping on each other).
Bottom line: Embrace version control from the start, even if it feels weird or slow at first. Before long, it’ll feel as natural as saving a file—and you’ll wonder how you ever coded without it.
Online Communities and Learning Boosters
You don’t have to figure everything out by yourself. Getting plugged into online communities can make your beginner programming journey way smoother. Most programmers cut their teeth by asking questions, even really basic ones, on places like Reddit’s r/learnprogramming, Stack Overflow, and Discord servers dedicated to their favorite languages. It’s totally normal to post code snippets and say, “Why doesn't this work?” Someone’s usually around to help you see what’s wrong or offer a shortcut.
Stack Overflow is kind of the go-to spot for most programming tricks. It holds millions of real conversations about error messages, best practices, and clever hacks. Fun stat: On Stack Overflow, over 80% of all tech questions eventually get answered, which is huge when you’re stuck at 2 a.m. and need a nudge in the right direction.
Community | Main Benefit | Active Users |
---|---|---|
Stack Overflow | Troubleshooting and code samples | >14 million |
Reddit /r/learnprogramming | Beginner-friendly Q&A and advice | 3 million |
GitHub | Open-source projects and collaboration | >100 million |
Discord servers | Live chat and support | Varies (some 100k+) |
Some sites actually gamify learning with bite-sized puzzles. Try Codewars or LeetCode for daily challenges. You’ll face tasks that make you think, but also let you compare your coding tips with people around the world. Some of these folks have job offers land in their inbox because they ranked high on the leaderboards.
Don’t stop at Q&A. Open-source communities on GitHub are perfect for beginners to contribute, even on tiny things like fixing typos. Every little contribution counts, and you’ll pick up industry-standard workflow secrets while you’re at it.
- Ask questions often—don’t worry about sounding clueless, everyone started somewhere.
- Search first before posting; chances are, someone already solved your issue.
- Read answers carefully. Sometimes the real learning to code happens in the discussion, not the final answer.
- Join Discords or Slack groups for lightning-fast feedback if you hate waiting for replies.
Staying active in these hubs also keeps you on top of new programming hacks—and there’s always something new to learn.
Building a Daily Coding Habit
If you want your programming tricks and all those coding tips to stick, consistency is the secret sauce. Practicing every single day—even just for 20 minutes—beats a long, brutal Saturday grind session. Byte-sized practice lets your brain build real muscle memory, so you’ll remember more and get less frustrated with each new challenge.
Here’s the deal: Stack Overflow once posted a user survey showing that most developers learned best by tackling small daily problems, rather than marathon study sessions. That’s because coding is kind of like learning a musical instrument—the more often you pick it up, the less intimidating it feels.
- Pick a fixed time. Whether it’s before work, during lunch, or right before bed, making it part of your daily routine means you don’t have to talk yourself into doing it every single day.
- Set micro-goals. Don’t aim to build the next Facebook in one sitting. Try writing a function, learning a new programming trick, or solving a simple coding puzzle—something small enough to finish in one go.
- Track your progress. Old school paper charts or modern apps like Habitica and GitHub’s contributions graph can make it feel a bit like a game (and a bit less like homework).
- Mix it up. Don’t just repeat the same thing over and over. Switch languages, try a random API, or follow along with a new coding tutorial, so you don’t get bored.
And here’s some simple math for motivation: if you spend just 20 minutes every day coding, that adds up to about 120 hours a year. Check out this breakdown:
Daily Coding Time | Weekly Total | Yearly Total |
---|---|---|
20 minutes | 2 hours 20 minutes | ~120 hours |
30 minutes | 3 hours 30 minutes | ~182 hours |
1 hour | 7 hours | ~365 hours |
That’s a whole lot of learning to code and picking up programming hacks without ever burning out. Remember, small steps daily beat heroic effort once a month every time.