Mastering PHP: Essential Tricks for Efficient Coding
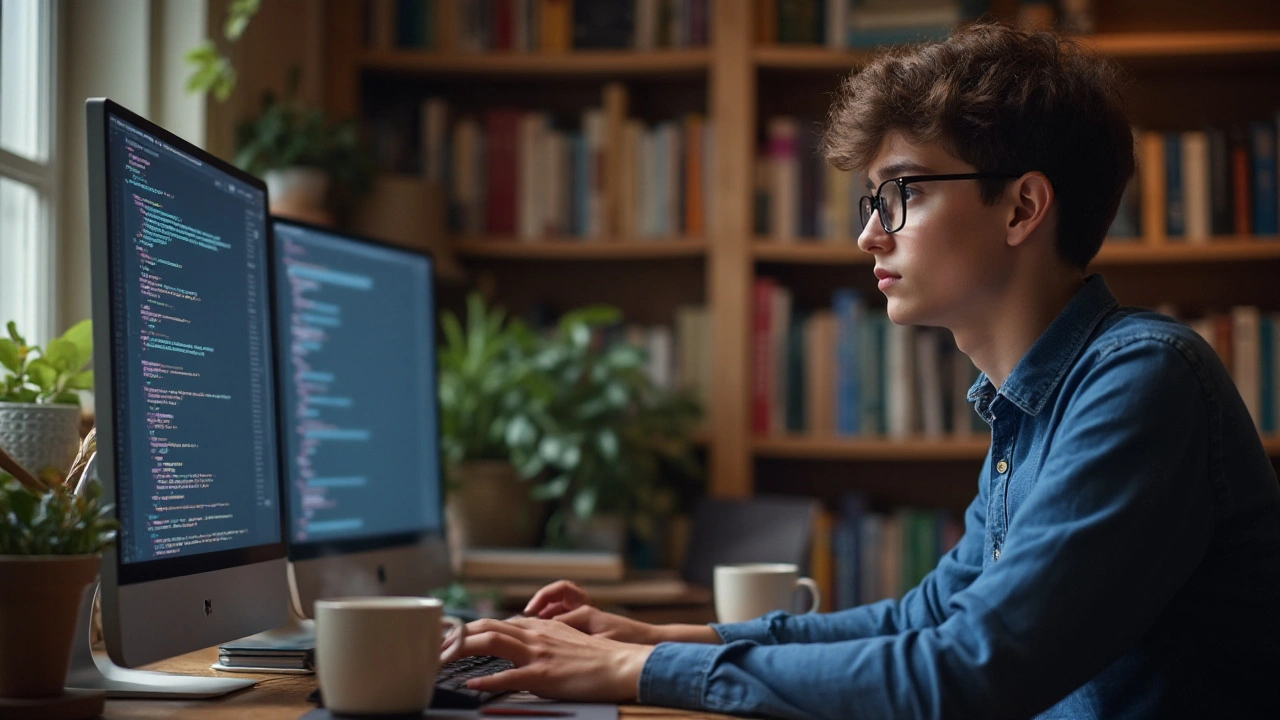
In the ever-evolving world of programming, efficiency is key. Whether you're a newbie or a seasoned coder, mastering the art of writing efficient PHP code can significantly enhance your workflow. But where do you start?
This article delves into some of the best PHP tricks and practices that will not only expedite your coding process but also improve the quality of your code. From organizing your scripts for better readability to leveraging PHP's powerful built-in functions, we'll explore a variety of strategies to help you code smarter, not harder.
Stay tuned as we dive into practical, time-saving techniques, ensuring that your journey through PHP programming is a smooth and successful one.
- Understanding PHP Basics
- Code Organization and Readability
- Optimizing PHP Scripts
- Effective Debugging Techniques
- Utilizing PHP's Built-in Functions
- Database Management with PHP
Understanding PHP Basics
PHP, or Hypertext Preprocessor, is a popular server-side scripting language primarily used for web development. It stands out for being easy to learn and widely adopted, powering millions of websites worldwide. One of the reasons for its popularity is its compatibility with numerous types of databases, offering a flexible foundation for web applications. Understanding the basics of PHP is crucial, as it sets the groundwork for more advanced coding techniques. The language is renowned for its embedded capabilities, allowing code to be seamlessly integrated with HTML, simplifying the process of creating dynamic web pages. PHP scripts are executed on the server, generating HTML which is then sent to the client. This structure guarantees that PHP code remains secure and out of the user's view, enhancing the security of web applications. Grasping these fundamental principles equips you with the tools to harness PHP's full potential.
As you dive deeper into PHP, appreciate its origins and evolution. Initially created by Rasmus Lerdorf in 1994 to track visits to his online resume, PHP has come a long way from its humble beginnings. By understanding its history, you can appreciate its functionality and why it incorporates certain features that make life easier for programmers today. PHP, over the years, has acquired extensive library support, enhancing its capabilities beyond simple scripting. Learning PHP basics involves mastering syntax, variables, control structures, and functions. The language supports popular paradigms such as procedural, object-oriented, and functional programming, making it versatile for diverse coding requirements. Master these core concepts to build a strong foundation in programming, laying the groundwork for more intricate projects. It may sound daunting, but breaking down these components into manageable sections allows you to progress confidently.
Why PHP Remains Popular
The longevity and continued use of PHP in the tech industry attest to its reliability and performance under various web development conditions. It thrives in situations where quick deployment of web services is necessary without sacrificing performance. PHP is open-source and boasts a large community, continuously contributing to its codebase and creating utilitarian extensions, making it one of the most practical options for developers seeking efficiency and adaptability. Many enterprises and content management systems, like WordPress, Drupal, and Joomla, rely heavily on PHP because it offers reliability that aligns with their need for high-traffic capability and user-friendliness.
"PHP is like a half-elf - a generalist language that, in the right context, becomes a master." - Jeffrey Way
Understanding variables, which may be thought of as containers for data, is pivotal in mastering PHP. Variables in PHP are dynamically typed, meaning their type is determined by the context in which they are used. This flexibility is boasted as an advantage, allowing developers to write adaptable code. Control structures, such as loops, conditionals, and switches, guide the program flow based on conditions or repetitive tasks. They are essential for writing efficient, logical code pathways. PHP also provides a rich set of built-in functions that tackle countless native operations, offering extensive libraries that reduce the time and effort needed to write code. These functions can handle everything from string manipulation to advanced mathematics. Embracing PHP's built-in capabilities is key to writing shorter, cleaner code.
Code Organization and Readability
When it comes to PHP programming, paying attention to code organization and readability can be a game-changer. Not only does it make your life easier when revisiting older projects, but it also tremendously aids anyone else who might be working with your code. A well-organized, readable codebase is like a neatly arranged library where every book is in its place, and labels are clear. One of the most effective strategies is to follow consistent naming conventions across your variables, functions, and classes. Choosing descriptive, intuitive names not only serves as a guide to what each part of your code is supposed to do but also significantly reduces the cognitive load. Putting thought into your naming conventions can transform an initially complex code into a logical narrative that is easy to follow and manage.
Breaking down your code into smaller, manageable functions is also crucial. This practice not only enhances readability but also promotes reusability, which is a cornerstone of efficient programming. There's a principle known as DRY, which stands for "Don't Repeat Yourself". It encourages developers to reduce the repetition of code by abstracting common functionality into reusable segments. Applying this principle effectively can save countless hours of coding and debugging. Moreover, modularizing code makes testing considerably easier because you can isolate pieces of your application and test them independently, ensuring each part is working correctly before integrating them.
"Programs are meant to be read by humans, and only incidentally for computers to execute." - Donald Knuth
Another tip for improving code organization is to diligently comment on your code. While this might seem like an additional effort, especially when you're on a tight deadline, detailed comments can be invaluable. They serve as breadcrumbs, explaining the logic behind complex segments or the purpose of certain variables. This practice is not just helpful but essential when working collaboratively since it bridges the gap between different coding styles and experiences. Additionally, many modern IDEs offer tools to automatically generate documentation from these comments, making them even more valuable.
Consistently using indentation and spacing also falls under best practices for code readability. This often overlooked aspect gives your code a clean structure, making nested conditions and loops easier to spot. This, paired with a logical file structure where related files and classes are grouped together, can turn a chaotic code directory into a clean workflow masterpiece. A good approach might be to always group your PHP code with similar scripts and assets, and maintain a clear hierarchy to avoid confusion.
Furthermore, employing a version control system like Git can substantially improve PHP code management. Not only does it track changes and record who did what, but it also facilitates seamless collaboration between developers. With practice, techniques like branching and merging become second nature, affording you greater agility in handling different features and fixes. This serves both as version control and as a parallel strategy for ensuring code quality, as it causes developers to be more mindful of the changes they introduce. In essence, a well-organized codebase is an investment in the future efficiency and scalability of your projects, effortlessly turning the task of programming into an art.
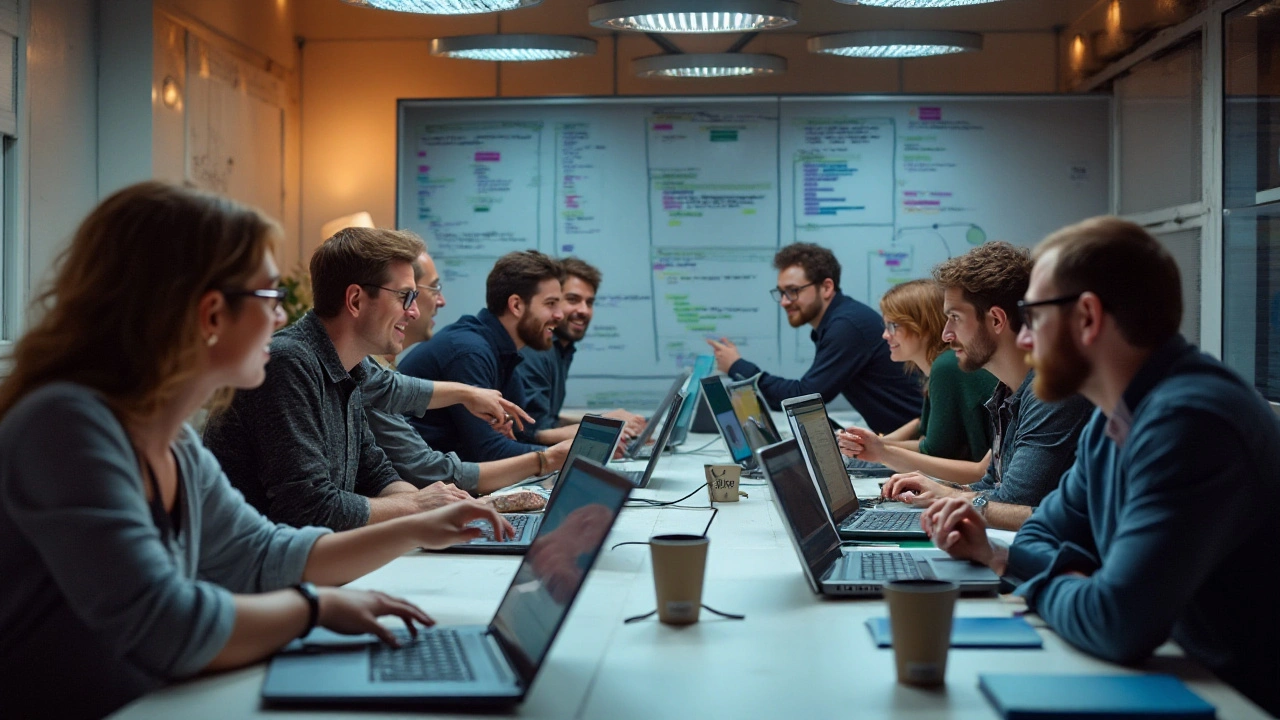
Optimizing PHP Scripts
When it comes to writing efficient PHP code, optimizing your scripts is an art that can both speed up execution time and reduce server load. This process not only entails refining the code itself but also understanding the environment in which it operates. Many programmers overlook the importance of minimizing overhead and maximizing resource efficiency, often leading to slower applications. A good starting point is to avoid the unnecessary repetition of database calls. Storing commonly accessed data in variables can significantly cut down on redundant database interactions, thus enhancing performance.
An often-cited adage is 'measure before you optimize.' This means taking a close look at the code that consumes the most resources. Using tools like Xdebug or profiling your scripts with XHProf can help gather invaluable insights. According to Martin Fowler, a respected software developer, "Premature optimization is the root of all evil," emphasizing that understanding bottlenecks should precede any optimization efforts.
Consider, for instance, avoiding the use of regular expressions unless absolutely necessary due to their complexity and resource consumption. Instead, opting for simpler string functions such as strpos()
or str_replace()
often yields faster results when handling string operations. Memory management also plays a critical role in script optimization. Use unset() to free up memory for large arrays or objects when they are no longer needed, releasing the server from holding unnecessary load.
Handling sessions wisely can have a profound impact on performance. By default, PHP sessions are file-based, which can become a bottleneck under heavy loads. Consider using a database or memory-based session storage for applications that need to handle vast concurrent users. On the topic of databases, query optimization is key. Reduce SQL query complexity and make sure to index the right columns in your tables. While learning to use JOINs efficiently might seem tedious, it's a game-changer for reducing server query processing times.
Embracing the power of PHP's built-in functions rather than reinventing the wheel can lead to substantial improvements as well. Built-in functions are optimized at a lower level since they are implemented in C. This often means they execute faster than custom implementations. Additionally, don't forget the impact of enabling PHP's OPcache extension. This caching engine stores precompiled bytecode in shared memory, eliminating the need for scripts to be loaded and parsed on each request.
Let’s not forget the importance of output buffering. Enabling output buffering can reduce the number of write operations by sending all output down to the browser at once. This can significantly speed up script execution, especially on systems with high I/O latency. As you continuously refine and optimize your scripts, your coding habits will evolve, often encouraging you to apply new strategies and principles in future projects, leading to faster, more efficient applications overall.
Effective Debugging Techniques
Debugging is an essential skill when it comes to programming in PHP. It can make the difference between long hours of frustration and a streamlined, efficient coding process. Understanding common pitfalls and learning how to effectively tackle them is crucial for any developer. For anyone who has experienced the bread crumb trail of error messages, a systematic approach to debugging is invaluable. Begin by isolating the problem; focus your attention on the specific section of code that might be responsible for the issue.
An effective method to do this is the use of breakpoints in your IDE or using PHP's built-in error messages. Make sure that error reporting is enabled during your development. This can be achieved by including the line error_reporting(E_ALL);
at the top of your script. It ensures that all errors, including notices and strict warnings, are displayed. This might seem intimidating at first, as it can flood you with information; however, it’s crucial for comprehensive debugging.
Another handy tip is employing PHP's var_dump()
and print_r()
functions. These are invaluable for examining the contents of variables and arrays. When used within the debugging process, they can give you clear insights into what data you're working with and where issues are arising. Additionally, consider leveraging the powerful Xdebug extension. Xdebug enhances PHP’s var_dump function and provides stack traces, memory allocation, and error notifications. This tool is popular among developers who prefer an integrated debugging experience.
"Debugging is like being the detective in a crime movie where you are also the murderer." — Filipe Fortes
It's worth noting that exhaustive logging can also be your best friend. Setting up a system where your application’s activities and potential errors are thoroughly logged ensures that problems can be identified even if they do not immediately manifest in physical errors or crashes. These logs can help trace back the steps leading to an issue, making the debugging process much more straightforward.
Debugging Approach
A structured approach to debugging involves a few key steps. Start by querying the problem - ask yourself what should happen versus what is happening. With each iteration, you might find another layer of the problem to resolve. Go step-by-step and adjust your code incrementally, testing between each modification.
Maintain a neat and organized codebase because cluttered code is prone to errors. This makes reading and understanding your code much faster, and you can pinpoint accuracy when debugging. Be diligent with your version control; tools like Git allow you to track changes and can be useful to revert back to a previous state if new code introduces issues. Implement unit tests for key components of your code. While testing doesn’t directly solve bugs, it acts as a preventative measure, ensuring that your code works as expected with given inputs.
Another important technique is to seek help when you’re stumped. The PHP community is vibrant and full of experienced developers willing to offer advice. Forums, chat groups, and discussion boards like Stack Overflow are great places to gain insights into a problem. One of the best ways to learn is by teaching, so explaining your problem to someone else can help illuminate the solution.
Lastly, embrace the power of documentation. Familiarize yourself with PHP’s rich documentation resources, which can provide vital insights into the quirks of different functions and libraries. Consistency and perseverance are your best allies in the journey of coding. Equipped with these debugging techniques, your experience will be less about firefighting and more about strategic problem-solving.
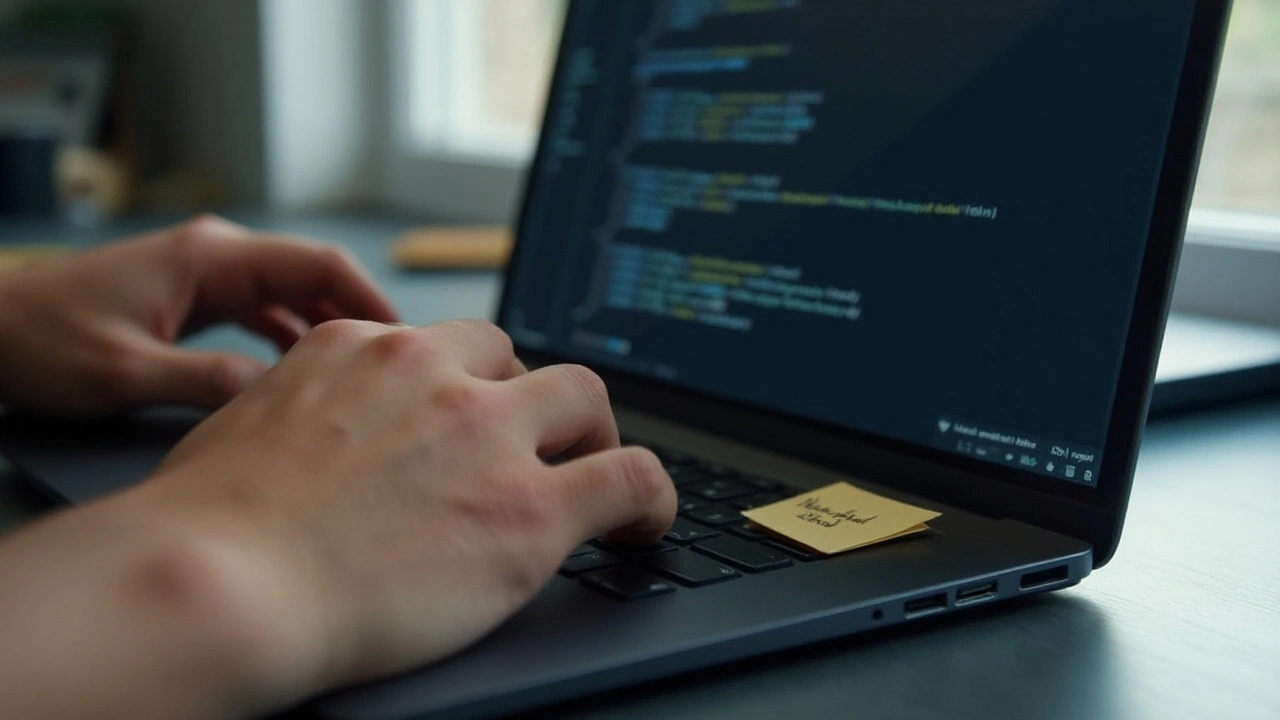
Utilizing PHP's Built-in Functions
Understanding how to harness PHP's built-in functions is akin to knowing the secret shortcuts in a game. These functions, ready to use at your fingertips, can dramatically streamline your programming process, eliminating the need to reinvent the wheel over and over again. With over a thousand native functions available, PHP offers a versatile toolbox that covers areas ranging from string manipulation and array handling to date formatting and data encryption. The trick lies not just in knowing about these functions, but in the adept application of them in your everyday code, saving time and reducing error rates.
Consider the everyday task of sorting an array. Sure, you could write a sorting algorithm from scratch, but why would you, when PHP provides built-in functions like sort()
and asort()
? These functions are optimized for performance and easy to implement, greatly simplifying your code. While sort()
sorts arrays by values, asort()
also maintains index association, which is particularly useful when working with associative arrays. It is in moments like these that the PHP ecosystem shows its strength, providing reliable, reusable solutions for common problems.
These functions also offer a level of consistency and reliability that homegrown alternatives may lack. For instance, PHP's hash()
function supports multiple hash algorithms, providing a straightforward solution for data encryption needs. Similarly, when handling date and time, the DateTime
class, with methods like format()
and modify()
, allows intricate manipulation without hassle. It allows intricate manipulation of dates without the hassle, ensuring your applications are future-proofed and locale-compliant. When you introduce built-in functions into your coding practices, you not only save time but also ensure your applications function securely and efficiently across a multitude of environments.
Some seasoned developers and PHP enthusiasts often share the sentiment captured by renowned author Rasmus Lerdorf, who once stated,
"I really don't like programming. I built this tool to program less so that I could add more accounting features to one of our applications at work."This encapsulates the essence of PHP's power: it's not about doing more with code, but doing more with less code.
Let us examine specific use cases. For example, when dealing with file handling, the file_get_contents()
function stands out as an efficient way to read a file into a string, minimizing the complexity and lines of code compared to traditional file read operations. Similarly, when exploring data types, functions such as is_array()
, is_string()
, and others ensure that your code remains robust against unexpected inputs, swiftly verifying the data types without manual type checks.
Function | Purpose | Example |
---|---|---|
array_merge() | Merge one or more arrays | array_merge($array1, $array2) |
explode() | Split a string by a string | explode(",", $string) |
json_encode() | Returns JSON representation of a value | json_encode($array) |
Utilizing the full range of PHP's built-in functions also means staying abreast of updates and improvements within the language. As PHP continues to evolve, so do its capabilities, often introducing new functions or enhancing existing ones to enhance performance and user customization options. Keeping your skills sharp and your code up to date guarantees that you're not just writing code, but crafting solutions with consistency, reliability, and a touch of elegance.
Database Management with PHP
When it comes to PHP and databases, the synergy is something every aspiring coder needs to harness to truly streamline their coding activities. Managing databases effectively is not just about knowing SQL or choosing the right database server; it’s about integrating them smoothly into your PHP applications. Whether you are working with MySQL, PostgreSQL, or any other database system, PHP provides powerful tools and extensions that can make your database interactions both efficient and secure.
Before diving deep, it's essential to underline the importance of using PHP Data Objects (PDO) over the now-deprecated mysql_ functions. PDO provides a uniform method of access across different types of databases, emphasizing flexibility and security. Notably, it supports prepared statements, which is a significant advantage for preventing SQL injection attacks—a common vulnerability that could expose sensitive data. Prepared statements not only increase security but also improve performance by allowing the database to optimize SQL query execution plans. A fascinating fact is the decline of traditional SQL injections as developers increasingly adopt PDO and other similar abstractions. In fact, according to a recent survey, websites that transitioned to prepared statements observed a marked reduction in potential security breaches.
The journey doesn’t end at just adopting PDO. Organizing your data access code by following best practices, such as implementing the Repository Pattern, ensures that your code remains clean and maintainable. This pattern essentially creates a layer of abstraction for your database interactions, meaning you can change your data source and underlying database-related code without altering your application's core logic. This approach not only improves maintainability but also makes unit testing much simpler, as databases can effortlessly be mocked out.
Optimizing database queries has a large impact on the application’s performance as well. An often overlooked practice is using indexes properly; they can drastically reduce query execution time. However, one must be careful, as excessive indexing can bloat the database and slow down data modification operations like insert or update. To help identify the right balance, tools such as MySQL’s EXPLAIN command are incredibly useful. This command reveals how MySQL executes queries, allowing you to tweak them for optimal performance. Furthermore, keeping your queries optimized ensures that your database remains responsive even under heavy load scenarios.
Managing databases isn't just about performance and security. Backup and recovery strategies are often undervalued until it’s too late. Regular backups, whether automated or manual, are critical in protecting data from unanticipated failures. With tools like mysqldump, snapshots can be taken with minimal hassle, ensuring continuity in case of server crashes or data corruption. A humorous industry quip also underlines this importance: 'People are divided into those who do regular backups and those who haven't yet lost data.' This statement, though amusing, underscores a hard-learned lesson in the tech community.
Lastly, monitoring and logging are two unsung heroes in efficiently managing databases with PHP. Continuous monitoring of your database provides insights that allow for proactive maintenance and resource allocation. Tools like phpMyAdmin or custom scripts utilizing PHP’s exec and shell_exec functions can be utilized to create alert systems that notify developers of anomalies, such as slow queries or failed connections, ensuring issues are addressed before they escalate into larger problems.