Essential Programming Tricks: A Guide for Aspiring Software Developers
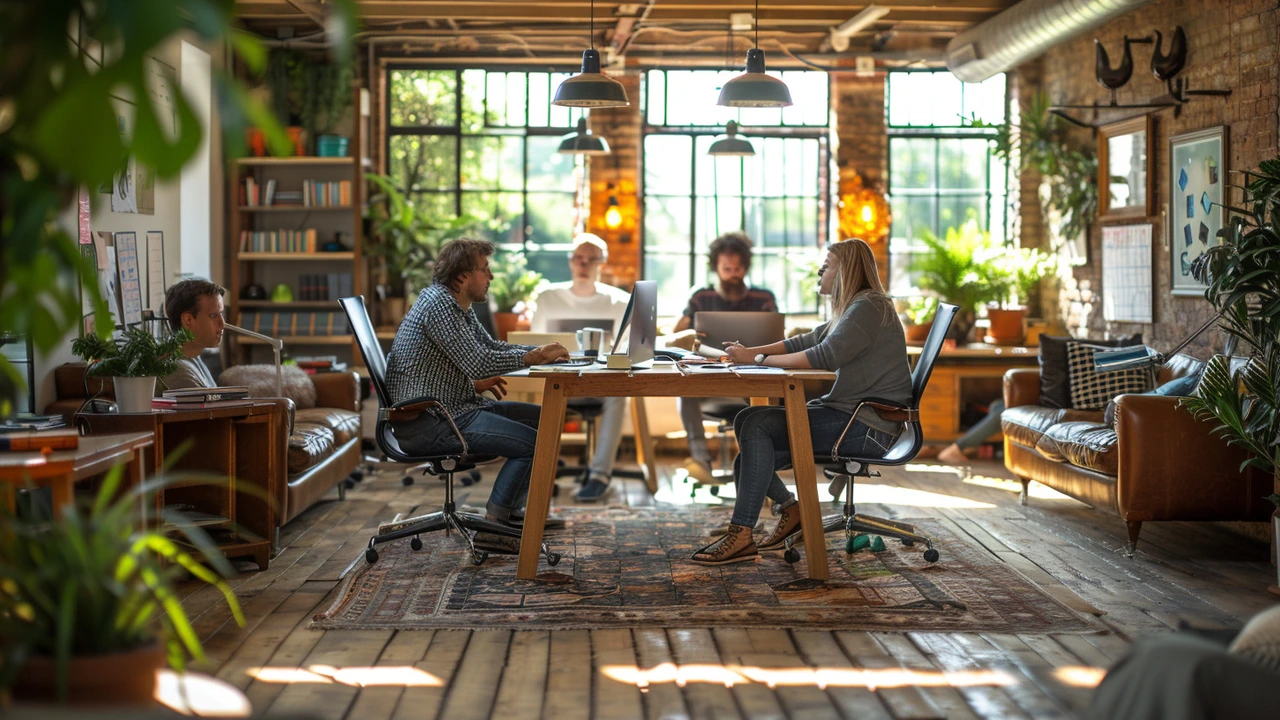
Diving into software development can be quite an adventure, filled with both challenges and triumphs. Whether you're just starting out or looking to sharpen your skills, having a reliable set of programming tricks up your sleeve can make a world of difference.
In this guide, you'll find practical tips to streamline your coding process, effective debugging methods to quash those pesky bugs, shortcuts to save you precious time, and best practices to keep your code clean and maintainable. Let's jump right in!
Quick Coding Tips
Kicking things off with some handy coding tips, there's nothing quite like a few shortcuts and efficient practices to elevate your programming game. One of the best ways to enhance your coding speed and accuracy is by mastering your favorite text editor or Integrated Development Environment (IDE). Whether it's Visual Studio Code, Sublime Text, or any other tool, spend some time learning the keyboard shortcuts and customization options it offers. These small adjustments can save you countless hours.
Speaking of time-saving hacks, get into the habit of writing clean and readable code from the get-go. This isn't just about aesthetics. Clean code is easier to debug, maintain, and scale. Follow consistent naming conventions, use comments judiciously to explain complex logic, and break down large chunks of code into smaller, reusable functions. This will make your life, and the life of anyone else who might work with your code, significantly easier.
Another tip is to make use of version control systems like Git. Not only does version control help you keep track of changes, but it also allows you to collaborate seamlessly with other developers. If you haven’t yet, get comfortable with basic Git commands such as commit
, pull
, push
, and merge
. Git can be a bit daunting at first, but investing time in mastering it will pay off big time.
While we’re at it, don't shy away from leveraging libraries and frameworks. They can save you from reinventing the wheel. Whether it's a front-end framework like React or a back-end framework like Express, these tools come with built-in functionalities that can streamline your development process. Remember, using libraries is not cheating; it's being smart with your time and resources.
Regularly practicing code review can also be very beneficial. By reviewing others' code, you’ll learn different approaches and techniques that you can incorporate into your coding style. Similarly, getting your own code reviewed by others can provide you with valuable feedback and insights. It’s a win-win situation.
“Code is like humor. When you have to explain it, it’s bad.” - Cory House
Don’t overlook the importance of unit testing. Writing tests for your code ensures that each part of your application works as intended. It might seem like extra work upfront, but it can save you from headaches down the line when bugs inevitably pop up. Use testing frameworks compatible with your language of choice, such as JUnit for Java or Mocha for JavaScript.
Finally, stay curious and keep learning. Technology evolves rapidly, and keeping up-to-date with the latest trends, tools, and best practices can give you a significant edge. Follow industry blogs, participate in developer communities, and try to work on personal projects outside of your main job. Continuous learning is a hallmark of a great developer.
In summary, improving your coding skills is a blend of mastering the tools of the trade, writing clean and maintainable code, leveraging existing resources, and continuously seeking knowledge.
Debugging Strategies
Debugging can be one of the most frustrating yet rewarding aspects of programming. When your code isn't working as expected, it can feel like hunting for a needle in a haystack. But with the right strategies, you can turn debugging into a more manageable and even enlightening experience.
First and foremost, it's crucial to replicate the bug. If you can't reproduce the issue consistently, solving it becomes a guessing game. Start by identifying the exact conditions under which the bug appears. This might involve using different sets of input data, running the program on different machines, or simulating specific user actions. Once you can reliably reproduce the problem, you're halfway to finding a solution.
Another effective method is to use print statements. It may seem old-school, but sprinkling your code with print statements can give you insight into what your program is doing at different execution points. It's often helpful to print out the values of critical variables at various stages of your program. This straightforward technique can give you clues about where things are going wrong.
“The most effective debugging tool is still careful thought, coupled with an inquisitive mind.” – Robert C. Martin
For more complex bugs, you might want to lean on debugger tools. Most modern development environments come with integrated debuggers that allow you to set breakpoints, step through code line by line, and inspect variable states in real-time. This hands-on approach can help you see exactly where your code deviates from expected behavior and pinpoint the root cause more efficiently.
Additionally, don't underestimate the power of code reviews. Sometimes, a fresh set of eyes can spot issues you've overlooked. Pair programming can be particularly effective here, as it involves actively collaborating with a colleague to go over problematic code. This collaborative approach not only helps in identifying bugs quicker but also promotes knowledge sharing and improves code quality.
Another valuable tip is to break the problem down. When faced with a large, complex bug, it can be overwhelming to tackle it all at once. Instead, try to isolate the issue by breaking your code into smaller, testable segments. Focus on one segment at a time, and use unit tests to verify that each part behaves as expected. By systematically narrowing down the problem, you can often identify the faulty component more easily.
For those of you working on web applications, browser developer tools can be exceptionally useful. These tools offer a suite of features for inspecting HTML, CSS, and JavaScript. You can use them to monitor network requests, debug JavaScript code, and even simulate mobile environments. With browser dev tools, you can gain a deeper understanding of how your web application operates, making it easier to identify and fix issues.
If you are dealing with performance-related bugs, consider using profiling tools. These tools can help you analyze your program's performance, identifying areas where your code is consuming too much time or memory. By focusing on these bottlenecks, you can make targeted optimizations that enhance the overall performance of your application.
Finally, remember that documenting your findings can be incredibly beneficial. Keep a log of the bugs you've encountered, the steps you took to reproduce them, and the strategies you used to resolve them. This documentation can serve as a handy reference for future debugging sessions and can be a valuable resource for your team members.
Time-Saving Shortcuts
Software development often feels like a race against the clock. Time-saving shortcuts can significantly boost your efficiency and productivity, making a big difference real fast. One of the most effective ways to cut down on coding time is to master keyboard shortcuts specific to your development environment. For example, in Visual Studio Code, using Ctrl + Shift + P opens the Command Palette, a powerful tool for accessing a multitude of commands and features. Similarly, Ctrl + B can swiftly toggle the sidebar. Knowing these shortcuts off by heart can save you plenty of minutes that add up quickly.
Beyond keyboard shortcuts, using code snippets can massively speed up repetitive coding tasks. For instance, in most modern IDEs, you can simply type a short keyword and auto-complete it into a block of code. This is particularly useful for repetitive, boilerplate code such as loops, classes, and method definitions. Tools like Emmet make writing HTML and CSS a breeze by expanding abbreviations into full code structures. Mastering these tools can increase your coding speed manifold.
Utilizing Templates and Libraries
Another excellent way to save time is by leveraging pre-built templates and libraries. Instead of starting from scratch for common functionalities, there are a plethora of libraries available for almost every programming need. For instance, if you're developing a web application, libraries like Bootstrap can help you create responsive designs quickly. Similarly, for JavaScript, using frameworks like React or Vue can not only save time but also bring efficiency into your coding with their powerful and reusable components.
Additionally, regularly updating and organizing your project templates can make your coding life easier. Having a go-to set of templates for different projects allows you to set up the basics in a matter of minutes. This is particularly useful if you're juggling multiple projects or working in a dynamic environment. According to a study by the Harvard Business Review, developers who utilize templates and libraries report a 27% increase in productivity.
"The right set of shortcuts and tools can be a game-changer. They aren't just about saving time; they're about making development work smoother and more enjoyable." — Kent C. Dodds, software engineer and educator.
Automation and Scripting
When it comes to saving time, automation is your best friend. Writing small scripts to automate repetitive tasks can free up a lot of your schedule. For example, using shell scripts to automate the build process or set up deployment pipelines can significantly cut down manual work. Tools like Jenkins or GitHub Actions can be used to automate testing and deployment, ensuring that every single change in your codebase is correctly tested and deployed without manual intervention. This is not only a time-saver but also reduces the risk of human error.
Moreover, automation can extend to regular code maintenance habits like code formatting and style checks. Tools like Prettier and ESLint can automatically format and lint your code according to the rules you've defined. This keeps your codebase clean and consistent without you having to worry about it constantly. Using these tools can save you the trouble and time of manually ensuring that your code adheres to the set guidelines every time you commit changes.
By incorporating these time-saving shortcuts, you'll not only enhance your productivity but also make your coding practices much more efficient. Remember, the ultimate goal is to work smarter, not harder. Utilizing these tips will help you reduce unnecessary steps, automate repetitive tasks, and focus more on solving complex problems and developing innovative solutions.
Best Practices for Code Maintenance
Maintaining the quality and readability of your code is paramount for long-term success in software development. Good code maintenance practices not only help in keeping your code clean and understandable but also make it easier to troubleshoot and modify in the future. Let's explore some essential practices to ensure your code remains in top shape.
Consistent Naming Conventions
One of the foundational practices for code maintenance is using consistent naming conventions. Names should be descriptive and follow a clear pattern that makes the code self-explanatory. This helps other developers (or future you) understand the purpose of each variable or function without needing extra documentation.Comment and Document
Proper documentation is the key to good code maintenance. While code should be self-explanatory as much as possible, well-placed comments can clarify why certain decisions were made or what the function of a complex algorithm is. Consider using docstrings in languages like Python or XML documentation comments in languages like C#.Modular Code
Breaking down your code into smaller, reusable modules can make a huge difference in maintainability. This means writing functions or classes that have single responsibilities and can be tested independently. Such modularity not only aids in easier debugging but also in refactoring and scaling your code.Version Control
Using version control systems like Git is an indispensable practice. It allows you to track changes, revert to previous states, and collaborate with team members without stepping on each other's toes. Platforms like GitHub or GitLab provide additional tools for code reviews and project management, making it easier to maintain your codebase.Automated Testing
Incorporating automated tests into your development process can save countless hours of manual testing and significantly reduce bugs. Unit tests should cover as many aspects of your code as possible, ensuring that each part works as intended. Integration tests will help confirm that different parts of the system work together smoothly.Refactoring
Regularly dedicating time to refactor your code can pay off in the long run. Refactoring aims to improve the code structure without changing its functionality, making it easier to read and maintain. Martin Fowler, a renowned software developer, once said,“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Code Reviews
Engaging in code reviews is a practice that can significantly enhance code quality. Having another set of eyes review your code can catch errors and suggest improvements that you might have missed. Peer reviews foster a culture of learning and continuous improvement within a development team.Continuous Integration/Continuous Deployment (CI/CD)
Implementing CI/CD pipelines can automate much of the tedious work involved in building, testing, and deploying code. This practice ensures that your code is always in a deployable state and reduces the risk of last-minute bugs or integration issues. tools such as Jenkins, Travis CI, or GitHub Actions can help automate these tasks.Keep Dependencies Updated
Another crucial aspect of maintaining your code is keeping all dependencies up to date. Outdated libraries can introduce security vulnerabilities and compatibility issues. Regularly check for updates and apply them in a controlled manner, ensuring that you test your code thoroughly after each update.By following these best practices, you can create a robust and maintainable codebase that will stand the test of time. Maintenance is not a one-time task but an ongoing process that should be integrated into your regular development routine. Embrace these habits, and your future self (and your teammates) will thank you.