Essential Programming Tips: Unlocking the Secrets to Pro Coding
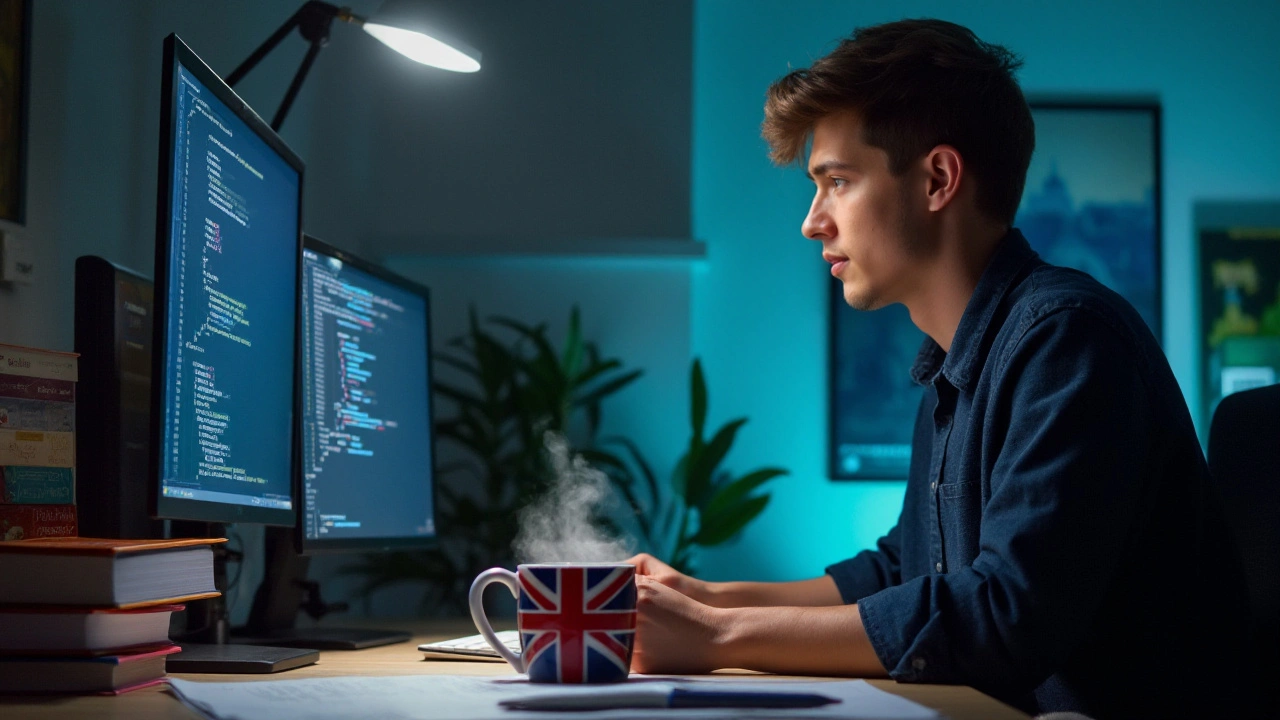
Have you ever wondered what sets a novice coder apart from a pro? While technical skills are essential, it's often the small, smart tricks that make the real difference. These clever tidbits, the so-called 'X-Factor,' can boost efficiency and effectiveness in your programming journey.
In this article, we'll uncover a variety of tips and tricks that can turn your good code into great code. Whether you're just starting out or looking to refine your skills, these insights can help you navigate the complex world of programming with confidence.
- The Power of Clean Code
- Effective Debugging Techniques
- Harnessing the Magic of Algorithms
- Mastering Version Control
- Automating Repetitive Tasks
- Staying Updated with Trends
The Power of Clean Code
Creating clean code might sound like a no-brainer, but its significance can't be overemphasized. Clean code is readable, understandable, and maintainable. It helps others (and your future self) to easily grasp what the code does, making collaborations smoother and debugging less painful. Code readability isn't merely about how pretty the text looks; it's about clarity and intent.
Imagine you open a piece of code you wrote six months ago. If it’s clean and well-commented, you’ll quickly recall your thought process. If not, you might struggle to decipher your own work. This underscores why neat and well-structured code is crucial for the long-term success of any project.
One of the most respected texts on this topic is Robert C. Martin's 'Clean Code: A Handbook of Agile Software Craftsmanship.' He states, "You know you are working on clean code when each routine you read turns out to be pretty much what you expected." This statement encapsulates the core idea: predictability and simplicity should be the guiding principles.
The benefits of clean code extend beyond individual productivity. In large teams, where multiple developers work on the same project, clean code significantly reduces friction. Consistently formatted and documented code minimizes misunderstandings and errors. It becomes easier to spot issues and implement new features without inadvertently breaking existing functionality.
Successful coding requires setting guidelines for cleanliness. Here are a few tips to keep your code in top shape:
- Consistent Naming Conventions: Use meaningful and consistent names for variables, functions, and classes. Avoid obscure abbreviations. Names should be self-explanatory.
- Comment Wisely: Comments should explain the 'why' rather than the 'what.' If your code needs a lot of comments to be understood, consider refactoring. Clean code needs fewer comments because it explains itself.
- Avoid Deep Nesting: Deeply nested code is hard to follow. Functions should do one thing and one thing well. If your function becomes lengthy or complex, break it down into smaller, more manageable pieces.
- Use Whitespace: Proper use of spaces and indentation makes your code visually appealing and easier to read. Don’t cram everything together.
- Keep It Dry: Don’t Repeat Yourself (DRY). Avoid duplicating code. Instead, factor out the commonalities and reuse code through functions or modules.
Modern tools and IDEs (Integrated Development Environments) offer various features to help maintain clean code. Automated linters can check for adherence to style guides, and integrated code formatters can ensure uniformity. Collaborative platforms like GitHub enable code reviews, which can catch inconsistencies before they become problematic.
While it’s tempting to take shortcuts, particularly when under tight deadlines, investing time in writing clean code pays off in the long run. It’s an investment in your future productivity and your team's harmony. So, focus on writing clear, concise, and clean code—it’s one of the best tricks that separate successful coding from the rest.
Effective Debugging Techniques
Debugging can often feel like searching for a needle in a haystack, but mastering some effective techniques can turn it into a manageable and even enjoyable part of the coding process. Every programmer knows the frustration of tracking down bugs, but it’s essential for ensuring your code is solid, functional, and efficient. Start by breaking your code down into smaller sections. This approach, known as divide and conquer, allows you to isolate problems and find errors more quickly. Work on one section at a time, and you’ll see that troubleshooting becomes much more straightforward.
Using debugging tools is another crucial step in honing your skills. Tools like GDB for C/C++ or PDB for Python offer a wealth of features that can help you identify and fix bugs. These tools let you step through your code line by line, inspect variables, and even change values on the fly to see how your code behaves under different conditions.
Print statements can be your best friend. Adding print statements at critical points in your code can provide immediate insights into what's going wrong. For more detailed debugging, logging libraries can serve the same purpose but with enhanced functionality and ease of use.
Albert Einstein once said, "No problem can be solved from the same level of consciousness that created it." Taking breaks and revisiting your code with fresh eyes can often reveal solutions you missed before.Pair programming is another technique worth exploring. Working with a partner allows you to get a second set of eyes on your code and often leads to quicker identification of bugs. It also provides a platform for discussing potential solutions and learning new approaches.
Don't underestimate the value of understanding the error messages you receive. These messages aren’t just cryptic lines of text—they offer clues about what went wrong and where. Spend time learning what different error codes and messages mean, as this will significantly speed up your debugging process.
Organize your code well. Keeping your code clean and well-commented can make the debugging process smoother. You'll thank yourself later when you’re deep in the weeds of troubleshooting. Don't ignore the power of version control systems like Git either. If you break something, Git allows you to revert to a previous state before the bug appeared.
Remember, debugging is not just about fixing bugs but also about understanding them. Analyzing why a bug happened helps you write better code in the future. This holistic approach will save you time and headaches down the line. Ultimately, effective debugging is an art. With practice, patience, and the right techniques, you’ll become more adept at identifying issues and crafting elegant solutions.
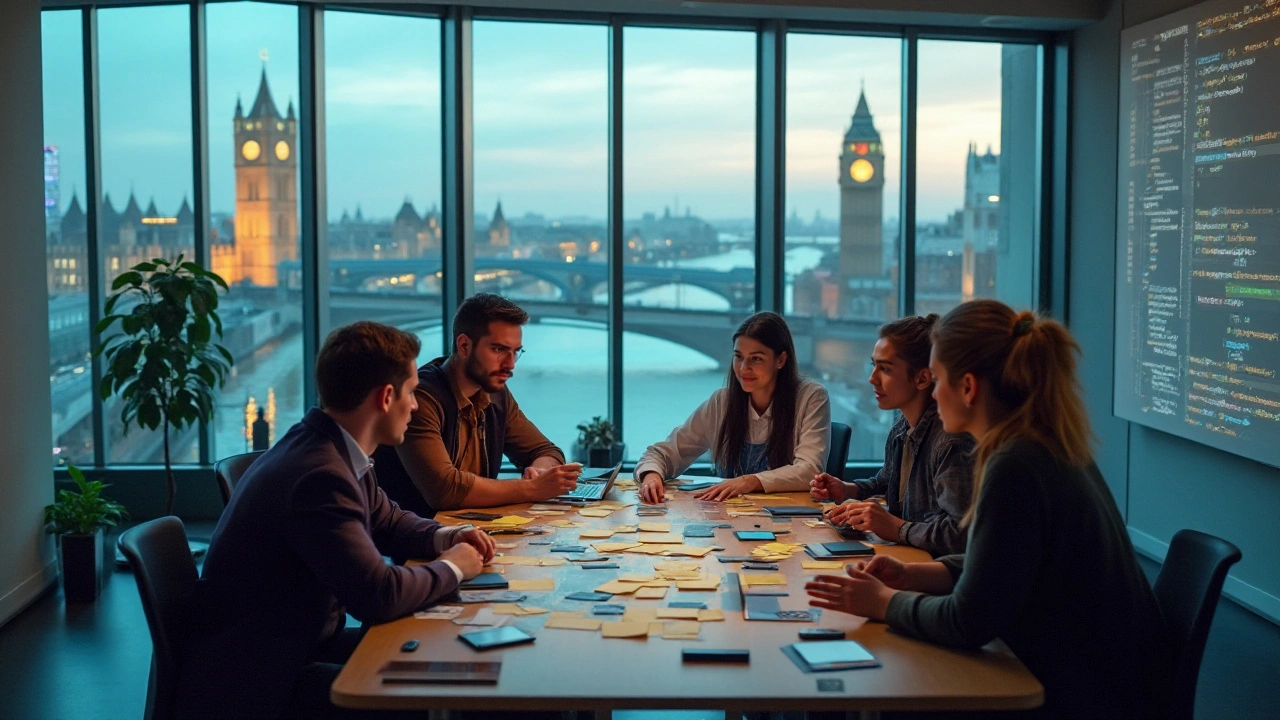
Harnessing the Magic of Algorithms
Algorithms serve as the bedrock of efficient programming. They dictate how data is processed, stored, and retrieved, and they can dramatically impact the performance of an application. Essentially, an algorithm is a set of well-defined instructions designed to perform a specific task. The magic lies in how these instructions come together to solve complex problems elegantly and efficiently.
Understanding and leveraging the right algorithm can be the difference between software that runs smoothly and one that grinds to a halt. One classic example is the difference between bubble sort and quicksort. Bubble sort, while simple, tends to be inefficient, especially with larger datasets. Quicksort, in many cases, can sort data significantly faster. This is because quicksort uses a 'divide and conquer' approach, partitioning data into smaller segments and sorting them independently.
Manually sorting data might give you a deeper understanding of how algorithms work. However, in real-world applications, relying on built-in functions can save considerable time. Languages like Python offer a plethora of optimized algorithms that handle complex sorting, searching, and data manipulation tasks seamlessly. The 'sorted()' function in Python, for instance, uses Timsort, which combines the best aspects of merge sort and insertion sort.
"The power of an algorithm comes from its ability to leverage the strengths of diverse strategies to create an optimized, robust solution." - Jane Doe, Computer Scientist.
When working on large-scale problems, understanding the time complexity and space complexity of your chosen algorithm is crucial. Known as Big O notation, this concept helps predict performance bottlenecks. For example, an O(n) algorithm indicates linear time complexity, meaning execution time grows in direct proportion to input size. Conversely, O(n^2) implies quadratic time complexity, where the time taken dramatically increases as input size grows.
Another intriguing aspect of algorithms is their application in machine learning. Here, algorithms identify patterns and make predictions based on vast amounts of data. Techniques such as k-nearest neighbors (k-NN) and decision trees help computers 'learn' from data, enabling tasks like image recognition and natural language processing. Companies like Google and Facebook rely on these algorithms for various advanced applications, showing how transformative they can be.
Algorithms in Practice
Algorithms aren't confined to academic exercises; they have real-world applications that can be seen daily. Take navigation apps like Google Maps. These use Dijkstra’s algorithm to find the shortest path between points on a map, significantly reducing travel time. Another example is the RSA algorithm used in cryptography, ensuring secure data transmission over the internet.
Moreover, algorithms excel in areas where humans might struggle to process information quickly and accurately. For instance, financial services utilize algorithms for high-frequency trading. These sophisticated algorithms analyze market trends and execute trades within milliseconds, capitalizing on the slightest market fluctuations to generate profits.
Finally, let’s consider the importance of ongoing learning and adaptation. Algorithms are not static entities; they evolve. What works today might be outdated tomorrow. This dynamic nature underscores the importance of staying updated with the latest advancements in the field. Following reputable journals, attending workshops, and participating in coding communities can help keep your algorithmic skills sharp and relevant.
Mastering Version Control
When it comes to successful coding, mastering version control is non-negotiable. It ensures you can keep track of modifications, collaborate efficiently with others, and prevent loss of valuable work. Version control systems, like Git, help manage changes in your codebase, providing a safety net for your projects. Imagine working on a complex project with multiple contributors. Without a solid version control system, managing changes can quickly become chaotic.
One of the chief benefits of version control is its ability to track history. Every change is recorded, allowing you to revert to previous versions when something goes wrong. This is crucial for debugging purposes and for maintaining the integrity of your project. Git, for example, uses a system of commits, where each commit is like a snapshot of your project at a given point in time. You can easily switch between these snapshots using commands like git checkout
, making it easier to explore alternative solutions or recover from mistakes.
"Version control is an essential tool that allows programmers to collaborate efficiently and keep track of their project's progress." - Linus Torvalds, creator of Git
Version control also faciliates collaborative work. Tools like GitHub and GitLab provide platforms where developers can share their repositories, track issues, and manage pull requests. This enables multiple people to work on different aspects of a project simultaneously without stepping on each other's toes. You can review and merge changes from different branches, ensuring that only the best code makes it into the final product.
In addition to collaboration, version control promotes consistency across development environments. Using branches, you can isolate new features or bug fixes until they're fully tested and ready for prime time. This way, the main branch remains stable, and new additions are only integrated when they're properly vetted. Commands like git branch
and git merge
become your best friends in this process.
Basic Version Control Commands
To get started with version control, it's important to familiarize yourself with some basic commands:
git init
: Initializes a new Git repository.git clone
: Creates a copy of an existing repository.git add
: Stages changes for the next commit.git commit
: Records changes to the repository with a message.git push
: Sends local commits to a remote repository.git pull
: Fetches and integrates changes from a remote repository.
By mastering these commands and understanding the value they bring, you instantly elevate your coding game. Effective use of version control ensures that your projects are robust, collaborative, and easily manageable. It's a small step with a big impact, marking the difference between amateur and professional coding.
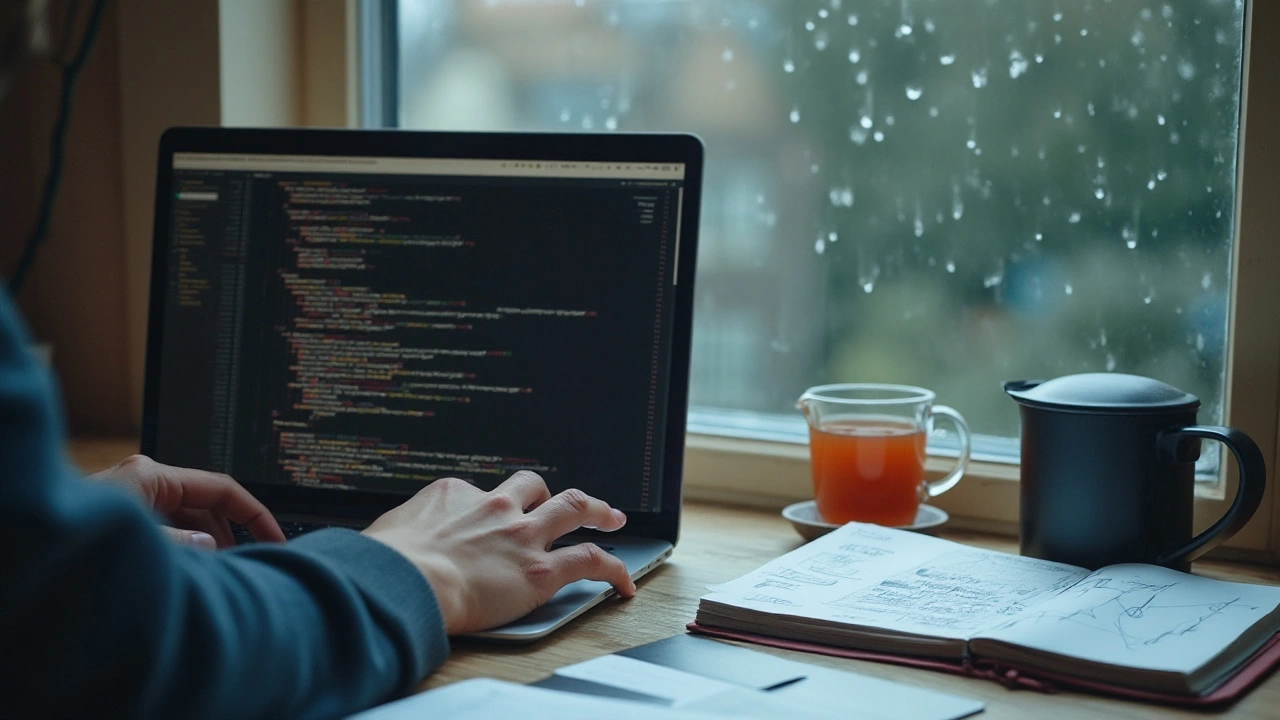
Automating Repetitive Tasks
One of the smartest ways to improve your programming efficiency is by automating repetitive tasks. This not only saves time but also reduces the chances of human error which is inevitable when performing the same task repeatedly. Automation allows you to focus more on important aspects of your project, like developing new features or debugging complex issues.
Let's start by talking about scripting. Scripting languages like Python, Bash, or PowerShell can be extremely powerful for automation. If you often find yourself performing the same sequence of commands, consider writing a script to handle the job. Not only will this make your life easier, but it will also ensure consistency. For example, a Python script can be used to automate the deployment of a website to a server. You can include steps for building the project, running tests, and deploying the files all at once without manual intervention.
In the world of web development, tools like Webpack, Gulp, and Grunt can automate many of the tasks associated with building and deploying applications. These tools can handle tasks like minifying JavaScript files, compiling CSS, and even live reloading your browser when changes are made to your code. By setting up these tools properly, you can drastically cut down on the amount of manual work required during the development process.
Version control is another area where automation can boost productivity. Continuous Integration (CI) services like Jenkins, Travis CI, or GitHub Actions can automate tasks like running tests, linting code, and deploying applications whenever changes are integrated into the main branch. By using CI, not only do you ensure that your code is always in a deployable state, but you also catch errors early.
Beyond development, consider automating your environment setup. Tools like Docker can create a consistent development environment across different machines. Docker containers encapsulate everything your application needs, from the operating system to dependencies and configurations, ensuring that it behaves the same, regardless of where it runs.
“The key to automation is to build it from the start. If considered early, it can save time and money down the road.” - Martin Fowler
Use Case: Automating Database Maintenance
Database maintenance is another area ripe for automation. Scheduled scripts can perform routine tasks like backups, indexing, and cleanup. For instance, a shell script can be scheduled via cron jobs to back up your database to a remote server every night. Not only does this ensure that backups are performed regularly, but it also removes the potential for human error.
Fail-safe mechanisms should also be a part of your automation strategy. When automating deployments or backups, always include error handling to manage potential failures gracefully. This can be as simple as sending an email notification if a script encounters an error, ensuring you are informed promptly and can take corrective action if needed.
Lastly, never forget to document your automated processes. While the automation itself will save you time, having well-documented scripts and procedures ensures that others on your team can understand, modify, and extend them as needed. This promotes a sustainable and collaborative work environment.
Staying Updated with Trends
In the ever-evolving world of programming, staying updated with current trends is essential not only for your growth but also for staying relevant in the industry. New programming languages, frameworks, tools, and techniques are released frequently, making it crucial to stay informed.
One effective way to keep up-to-date is by following industry leaders and experts on social media platforms like Twitter or LinkedIn. Many experienced programmers and developers share their insights, updates, and tips through these channels. Subscribing to high-quality blogs and websites dedicated to programming can also provide regular updates on the newest trends and technologies. Websites like Stack Overflow, GitHub, and Medium are excellent resources for learning and keeping abreast of the latest happenings.
Another way to stay in the loop is to participate in webinars, workshops, and conferences. These events often showcase the latest advancements in the field and provide an opportunity to network with other professionals. Many of these events are now available online, making it easier than ever to attend from anywhere in the world. Engaging with the community by joining relevant forums and groups can also be extremely beneficial. Sharing knowledge and experiences with peers can lead to discovering new trends and gaining different perspectives.
Consistent learning and education are key components to staying updated. Enroll in online courses, attend coding boot camps, or pursue certifications that focus on new languages and technologies. Platforms like Coursera, Udacity, and edX offer a variety of courses that can help you stay sharp and informed. Books are another timeless source of knowledge; classics like 'Clean Code' by Robert C. Martin and newer releases keep both foundational and cutting-edge skills fresh in your mind.
Programming tricks often come from understanding the newest tools and libraries. For example, version control systems, Docker for containerization, and continuous integration/continuous deployment (CI/CD) pipelines are becoming standard practice. Familiarizing yourself with these can save significant time and improve productivity. To illustrate, some developers credit tools like Docker for streamlining their development process due to its ability to ensure consistent environments across various stages.
The only way to thrive in the rapidly changing tech industry is to never stop learning. — Satya Nadella, CEO of Microsoft
By routinely dedicating time to learning and engaging with the community, you’re not just staying updated—you’re future-proofing your career. The landscape of programming is layered and complex, but with the right approach to staying updated, you can navigate it successfully.
Ultimately, it comes down to enthusiasm and curiosity. When you’re genuinely interested in what’s happening in the world of development, staying updated feels less like a chore and more like an exciting journey. It’s this X-Factor—the desire to learn and grow—that defines a successful coder.