Unlocking Python Magic: Discover the Hidden Tricks of Python Programming
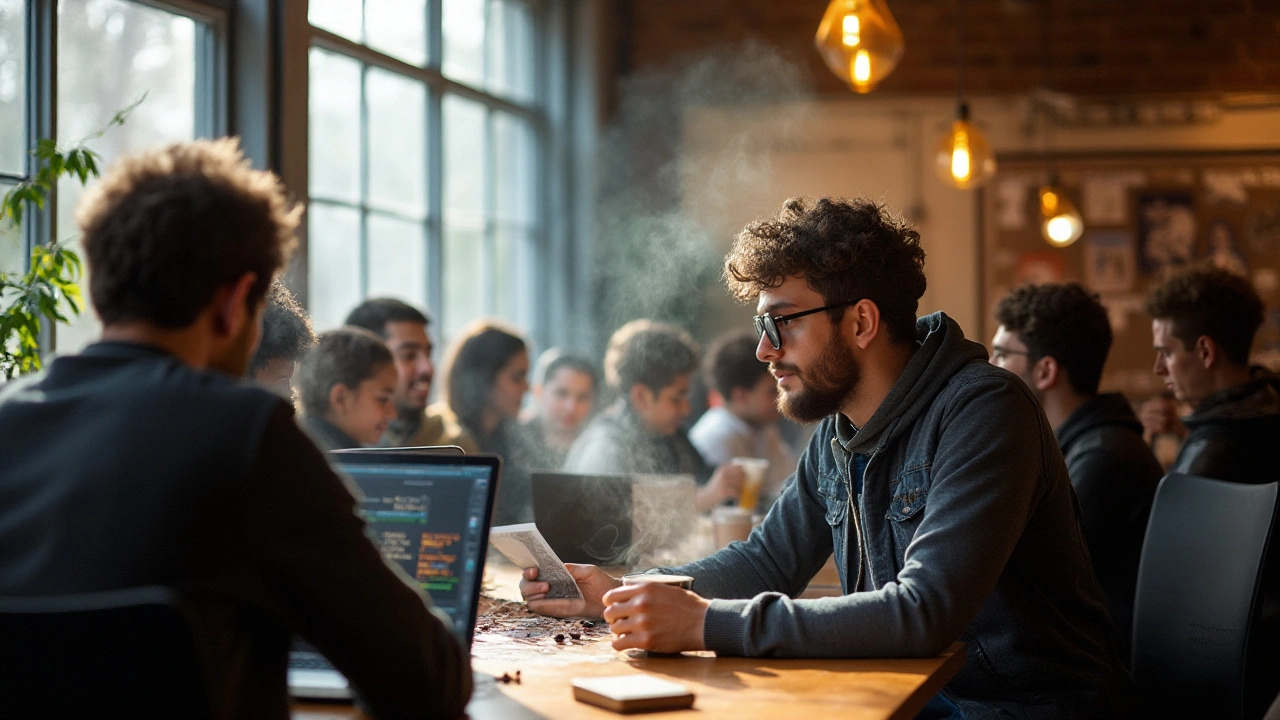
Python has emerged as one of the most popular programming languages in modern times, providing a seamless experience to developers across the globe. Known for its simplicity and readability, Python is not just about the fundamentals; it's about the hidden gems that can transform regular code into something extraordinary. In this guide, we'll take a journey beyond the basics to uncover some of the intriguing tricks that Python offers. These tricks are more than just snippets; they're pathways to crafting cleaner, more efficient code.
Whether you're starting your coding adventure or you're a seasoned Pythonista, these insights can be incredibly beneficial. We're going to walk through various topics, from the elegance of list comprehensions to the efficiency of generators, each segment designed to resonate with anyone looking to up their Python game. With the wealth of libraries available and Python's dynamic nature, there's always something new to learn. Dive in, and let's start this exploration into Python's hidden potential!
- Understanding Pythonic List Comprehensions
- Leveraging the Power of Generators
- Mastering the Art of Decorators
- Exploring the Wonders of the itertools Library
- Harnessing Python's Dynamic Typing
- Debugging Techniques with Python
Understanding Pythonic List Comprehensions
List comprehensions in Python are not just a way to create lists quickly. They represent a paradigm that captures the essence of Python: concise, expressive, and readable code. Originating from the set-builder notation in mathematics, list comprehensions allow developers to construct lists in a single line, offering a more understandable syntax over conventional loops. Imagine needing a list of squares for numbers 0 to 9; instead of writing a generative loop, a list comprehension does the job in an elegant way: [x**2 for x in range(10)]
. This expressiveness not only reduces the code length but also enhances clarity, showing at first glance the intent of the code. The neatness and efficiency are the hallmarks of Python, making it a favorite for cleaner code enthusiasts.
Beyond simplicity and elegance, list comprehensions in Python are also about speed. Benchmarks often suggest that using list comprehensions can significantly improve execution times compared to traditional loops – a fact that reinforces their popularity among time-sensitive applications. This speed factor arises because list comprehensions are optimized at a lower level, making them a fascinating subject of study in computer science courses focused on Python. As we've seen from various Python communities, experienced developers often encourage beginners to embrace list comprehensions as a step towards writing more "Pythonic" code, a term that stands for idiomatic, canonical, and efficient Python code writing.
There is more under the hood when it comes to list comprehensions; they support conditional logic, empowering developers to filter lists on the fly. For instance, if you needed to filter out even numbers while squaring those from 0 to 9, it would look like this: [x**2 for x in range(10) if x % 2 != 0]
. This conditional feature introduces another layer of utility and readability, allowing Python scripts to handle data and decisions in a powerful yet straightforward manner. The inclusion of conditions also makes the comprehensions adaptable and versatile for different scenarios – from simple to complex data transformations, list comprehensions have got you covered.
"Code is like humor. When you have to explain it, it’s bad." – Cory House
Embracing list comprehensions does not mean compromising on other advanced data manipulations. They can be effortlessly combined with functions within Python, such as sum()
or len()
, allowing for complex operations inside comprehensions. Consider the task of calculating the sum of squares of odd numbers up to 9: sum([x**2 for x in range(10) if x % 2 != 0])
. Such is the versatility of list comprehensions, which become the building blocks for rapid and efficient data manipulation in everyday coding challenges. As such familiarity grows, the opportunities to optimize one's code become apparent, unveiling the true potential of Python programming through a single, powerful construct.
As powerful as they are, list comprehensions aren't without limits. They are best used for simple and moderately complex operations. When overused, particularly with nested comprehensions, readability can suffer—a drawback that any seasoned coder advises against. For more intricate logic, Python offers alternatives like generator expressions and map/filter functions that could be more appropriate. Therefore, the balance must be struck where list comprehensions are applied to achieve desired clarity and performance levels without sacrificing maintainability. Nevertheless, they remain one of Python's best-loved features, appreciated for their role in maintaining the delicate performance-readability equilibrium.
Leveraging the Power of Generators
Generators in Python are like special artists in the world of programming, capable of creating large datasets without consuming much memory. They are a unique kind of iterator, and unlike normal functions, they don’t just spit out an answer all at once. Instead, they've got this cool power of 'yielding' values one at a time. This is particularly handy when dealing with large sequences of data where memory efficiency is a priority. Generators work like a charm because the data is 'generated' on the go instead of loading everything into memory at once. Imagine reading a massive book but only one page at a time while cleverly bookmarking every page you leave. That’s essentially what generators do with your data.
So, how do we tap into this magic? Well, the keyword 'yield' plays the lead role in any generator function. Unlike 'return' that ends the function, 'yield' pauses it, saving the function’s state for resumption later. This literally ‘hands the baton’ back to the runtime just right there. When the function is invoked again, it picks up from where it left off. An example could be a generator that creates an infinite sequence of numbers. This wouldn’t be feasible using a normal list due to memory constraints, but with generators, it’s smooth sailing. This is what makes them irreplaceable in creating sequences where memory is a tight squeeze.
Additionally, the performance gains you get from using generators are often surprising. When large dataset processing speed becomes a bottleneck, generators save the day with their lazy evaluation. This delayed execution helps you avoid the time delays and memory pitfalls associated with evaluating entire data structures at once. Generators find their niche in scenarios demanding high efficiency, such as streaming data or handling real-time analytics. “Generators had a profound effect on the Python programming language” remarked Guido van Rossum, the creator of Python. It’s their ability to usher in performance without overhauling existing logic that makes them a prized tool in a developer’s toolkit.
Below is an example showcasing the strength of generators. Consider this generator function that efficiently handles large file processing:
def read_large_file(file_object):
for line in file_object:
yield line
Notice how we use the 'yield' keyword for producing lines one at a time? This snippet could be a lifesaver when working with colossal log files, keeping memory usage to a bare minimum. Here, instead of loading entire lines into memory, Python smartly keeps memory footprint low by only loading a line at a time. When performance is the game, generators provide solutions that are often as elegant as they are effective.
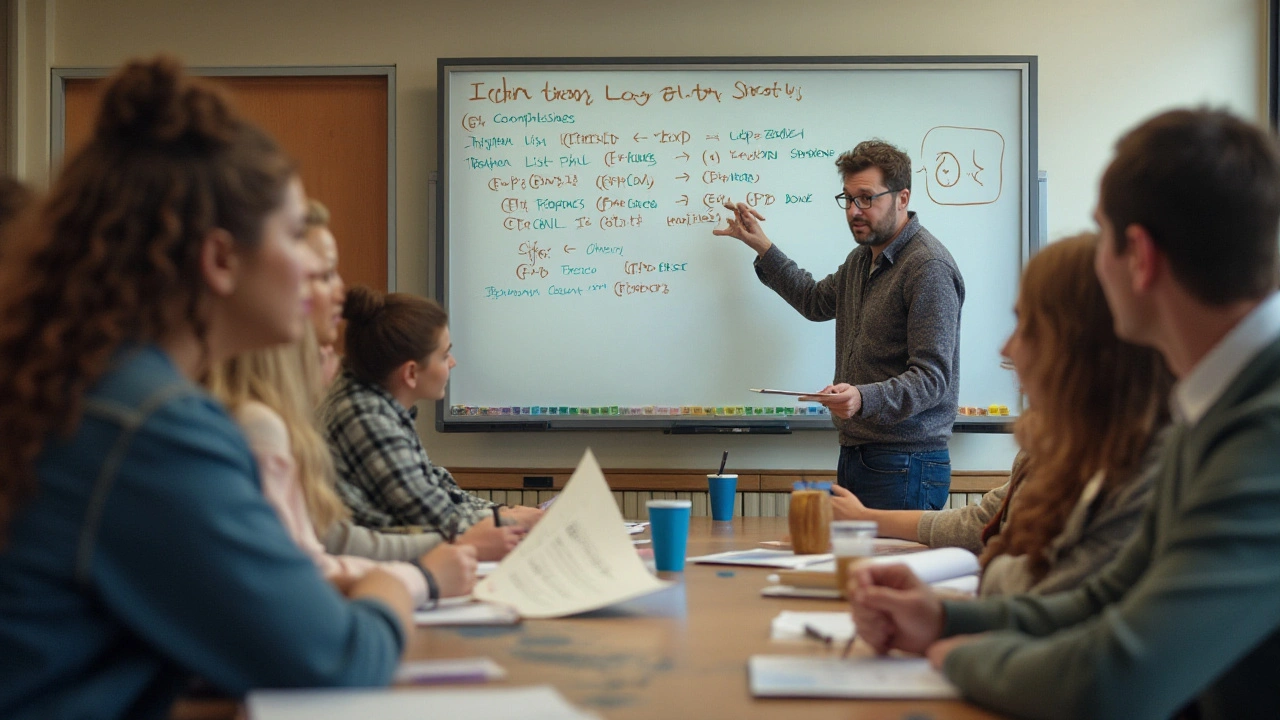
Mastering the Art of Decorators
When it comes to elevating a Python program from merely functional to truly ingenious, decorators emerge as a favorite tool among developers. They allow you to add functionality to an existing code in a nuanced way that keeps your code DRY— Don't Repeat Yourself. Decorators are essentially functions that take another function and extend its behavior without explicitly modifying it. This makes them an invaluable asset, particularly when you're working on scalable applications that demand flexibility and reuse.
Understanding the syntax and application of decorators can initially be daunting, but it's akin to understanding the first chords on a guitar. Start with your function. Define a wrapper function within it that manipulates the behavior you want to enforce. From there, the Pythonic '@' symbol is used, preceding the decorator name above your function. This simplicity in applying decorators belies the complex and powerful feature it represents. As design patterns, decorators aid in clear separation between business logic and systematic concerns like logging or authorization.
Common Use Cases for Decorators
One might wonder, precisely where do decorators shine? Common use cases include logging, which is vital for debugging and monitoring processes, and enforcing access control checks. A developer can also create their own decorators to add retries in case of failure, timing a function for performance analysis, or even caching results to optimize performance. By designing a decorator to wrap around essential functions, users can build modules with increased efficacy and elaborate interconnected processes.
As stated by Raymond Hettinger, a distinguished Python core developer, "Decorators abstract away simple patterns in an extensible and reusable way." This succinctly captures the importance of decorators in crafting streamlined and effective Python code.
Feature | Use Case |
---|---|
Function Logging | Tracks function calls for debugging purposes. |
Authentication | Restricts access to ensure authorized use. |
Implementing decorators to handle repetitive cross-cutting concerns leads to a cleaner, more organized codebase. The decorator's ability to quickly modify the behavior of existing functions means that even legacy code can reap the benefits of modern developments. Importantly, decorators should be applied thoughtfully. Overuse or creating overly complex decorators can muddy the code, defeating their primary benefit of simplicity and enhanced functionality.
The path to mastering decorators lies in iterative practice and explorative learning. Delve into open-source projects and see how decorators are used in real-world situations. Experiment in your personal projects by starting simple, and gradually integrating complex decorators that push the boundaries of your understanding. This way, decorators transform from mysterious constructs to reliable instruments in a developer's toolkit.
Exploring the Wonders of the itertools Library
When delving into the realm of efficient and elegant code, the itertools library stands out as a powerhouse in the Python standard library. Designed to create fast and memory-efficient code, especially useful in scenarios with large datasets, itertools acts like a Swiss army knife for iterator operations. At its core, the library provides functions that work on iterators to produce complex iterators, simplifying the creation of advanced iteration patterns without requiring custom generator functions.
One of the most compelling features is the function chain, which allows you to take several iterators and treat them as a single long one. Imagine having multiple lists that you want to process in sequence; instead of manually iterating over each one, chain stitches them together seamlessly. Another gem is combinations, which is ideal when you need to generate all possible pairs or tuples from a set of elements. This can be particularly valuable in algorithm design where state-space exploration is required. The elegant syntax and flexibility allow programmers to solve problems that would otherwise require more verbose code, demonstrating Python's ethos of simplicity and power.
Stepping further into the library, the permutations function comes into play when order matters, generating all permutations of a list. Consider the scenario in a puzzle game development where you need to evaluate every possible order of steps; permutations can automate this process easily. Alongside permutations, product offers Cartesian products, crucial for scenarios demanding matrix-like combinations of elements. These functions, together, highlight the dense capability of itertools in handling combinations and permutations effectively.
Alex Martelli once remarked, "The itertools module is invaluable for any serious Pythonista exploring the depths of what Python can achieve with minimal syntactical overhead." His words resonate with many experienced developers who find itertools an indispensable tool in their coding arsenal.
Beyond simple operations, itertools also incorporates some fascinating infinite iterators that can handle infinite sequences. The count function, for example, is the equivalent of an endless loop counting forever, ideal in scenarios like rollovers or cyclic workflows where operations need to repeat indefinitely. Paired with cycle, which repeats an iterator’s contents endlessly, these tools can dramatically simplify managing repetitive, deterministic sequences in applications such as simulation models or continuous integration pipelines.
In practical applications, combining itertools functions yields remarkable results. Imagine a table configuration part of a seating arrangement tool where every potential seat choice needs to be considered. By applying product alongside combinations_with_replacement, developers can effortlessly map out seating possibilities respecting constraints like table size or guest preferences. The result is not just optimal code but clarity and efficiency that can positively influence both development time and runtime performance. Plus, by leveraging Python's dynamic ecosystem, itertools fits seamlessly with other libraries like pandas or numpy, expanding its reach and capability in data analysis realms too.
To illustrate the flexibility and ease of use, consider the following small, practical example that demonstrates itertools' chain and combinations at work:
- Imagine you have items = ['A', 'B', 'C', 'D']. Using combinations, you can effortlessly generate all possible pairs.
- Invoke itertools.chain([1, 2, 3], [4, 5, 6]) to treat multiple lists as a single iterable.
- This sequential human-like narration brings you closer to understanding the unconventional elegance of itertools.
The wonders of the itertools library rest in its ability to transform cumbersome tasks into bite-sized solutions, elevating a programmer's efficiency and sense of achievement. As you continue to unlock the myriad possibilities that Python offers, never underestimate the profound simplicity encapsulated in the itertools module, an indispensable part of any Python-based exploration.
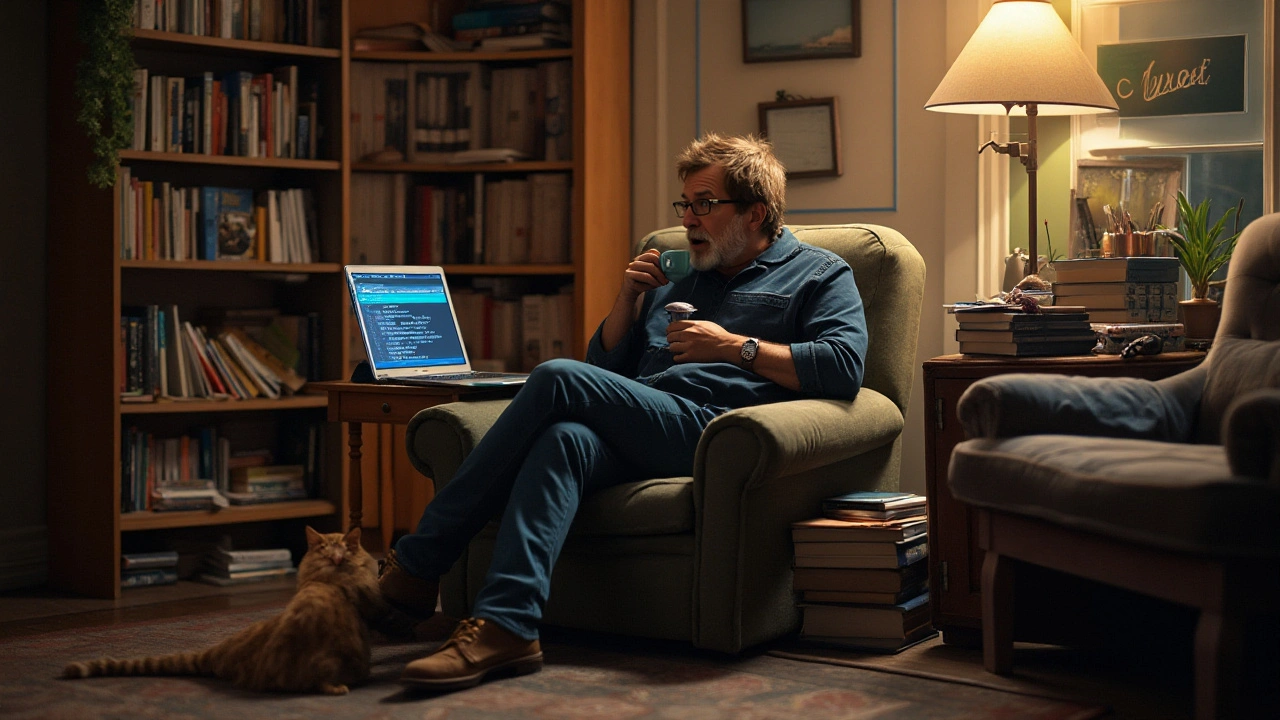
Harnessing Python's Dynamic Typing
Python, renowned for its simplicity and readability, owes much of its charm to dynamic typing. This feature, which sometimes piques curiosity, allows variables to change their type during execution. Unlike statically-typed languages such as Java or C++, where the type is defined during compilation, Python determines the type at runtime. This flexibility means developers can write code more swiftly and succinctly, without specifying data types explicitly. Whether you're dealing with integers, strings, or complex objects, Python's dynamic nature permits a seamless transition. This capability, coupled with Python's robust error-checking at runtime, empowers developers to focus more on crafting logic and less on syntax constraints.
With Python’s dynamic typing, you can interact with a variable in different ways throughout its lifecycle. While it might seem like a recipe for chaos, seasoned developers know it's a powerful ally. Embracing dynamic typing enables unique programming techniques. For example, functions designed to accept various argument types without strict definitions often result in cleaner, more versatile code. The absence of fixed data types fosters creativity, enabling developers to explore novel solutions to complex problems. This freedom often sparks innovation in the tech world. As Mona Gupta, a renowned software engineer at Tech Innovations, once remarked, "Python’s dynamic typing is akin to the invention of the automobile—it freed us from the rails of static typing, opening up new horizons in programming."
Yet, this liberty also requires responsibility. Dynamic typing could potentially lead to unexpected bugs if not managed carefully. Seasoned programmers suggest using type hints as a safeguard. Introduced in Python 3.5, type hints allow developers to specify expected variable types, providing a template for future reference. This blend of flexibility and caution ensures code remains maintainable and understandable, even as project complexity grows. Tools like mypy can enforce type checking at a later stage, offering a best-of-both-worlds scenario where you enjoy dynamic flexibility and the reassurance of type safety. The key lies in balancing innovation with discipline, allowing Python's features to streamline your development process while maintaining clarity.
Benefits of Python's Dynamic Typing
Dynamic typing in Python extends beyond mere comfort—it contributes meaningfully to faster prototyping and iterations. In an environment where time-to-market is pivotal, Python's flexibility often translates to decreased development cycles. Developers can pivot the direction of an application without rewriting significant portions of the codebase. This agility lowers costs and accelerates feature delivery, making Python an attractive choice for startups and processes demanding rapid changes. Interestingly, a recent survey showed that 86% of Python developers reported improved coding efficiency due to dynamic typing. Such statistics underscore the broader industry acknowledgment of its practical advantages.
Finally, working with Python tips and tricks that leverage dynamic typing not only optimizes current coding practices but also fosters a deeper understanding of this elegant language. As projects scale, the ability to iterate quickly and apply creative solutions becomes increasingly crucial. By mastering Python’s dynamic typing, developers equip themselves with a versatile tool that's indispensable in today's evolving programming landscape.
Debugging Techniques with Python
Debugging is an essential skill that can make or break your Python programming journey. While writing code may engage your creative side, debugging taps into your analytical skills, transforming you into a detective of sorts. Python offers a multitude of ways to debug code effectively, allowing developers to streamline their workflow and catch errors before they spiral out of control. One of the simplest ways to begin your debugging journey is by using print statements strategically. By placing these statements at critical points in your code, you can monitor the flow of your program and identify where things may be going awry. This method may seem rudimentary, but it's often a programmer's first line of defense against unseen errors.
For those looking to delve deeper, Python's built-in pdb
module offers an interactive tool that can be a game changer. The Python Debugger lets you set breakpoints and examine program execution one line at a time. Unlike print statements, it doesn’t require modifying the code's logic. You can step through your script, check variable values, and make on-the-fly changes, gaining profound insight into your program's behavior. As David Malan of Harvard University famously pointed out, "Programming isn’t about what you know; it’s about what you can figure out." This interactive approach fits that ethos perfectly.
Another modern approach in debugging is employing logging instead of, or alongside, simple print statements. The logging
module provides a flexible framework for adding log statements to code. Unlike print statements, logs can be categorized into different severity levels, such as DEBUG, INFO, WARNING, ERROR, and CRITICAL. This adds a layer of scalability; as your application grows, these logs help maintain an organized record of runtime events. They not only help track down bugs but also provide insights into application use and operations.
If you want more automated solutions, consider using an Integrated Development Environment (IDE) with built-in debugging features like PyCharm or VSCode. These IDEs offer sophisticated tools like visual breakpoints, variable watch windows, and call stack views, making them invaluable for serious developers. Within these environments, you can manipulate code execution and backtrack through failure points efficiently. They may come with additional features like real-time code analysis and quick-fix suggestions, too.
For complex applications running on multiple servers or environments, remote debugging can be a life-saver. In Python, this can be achieved using packages like rpdb
, which extends Python’s native debugger to facilitate remote connections. By attaching to a running process from a different machine, developers can diagnose live systems without causing downtime or halting operations. This technique is particularly useful for distributed systems where traditional debugging methods may fall short.
According to Guido van Rossum, Python's creator, "Often, the best way to figure out a problem is to step away from the code and take a fresh look at it." Dynamic languages like Python encourage iterative bug fixing, steering developers towards progressively refining their solutions rather than jumping directly to an incorrect conclusion.
In summary, mastering debugging techniques doesn’t just make you better at fixing bugs; it also enhances your overall problem-solving skills and intuition as a programmer. Whether it's through simple print statements, leveraging advanced IDE tools, or implementing sophisticated logging practices, Python offers versatile options to enrich your debugging repertoire. Embrace these tools, and take your coding craft to the next level.