The Ultimate Code Debugging Guide for Beginners: Mastering the Basics
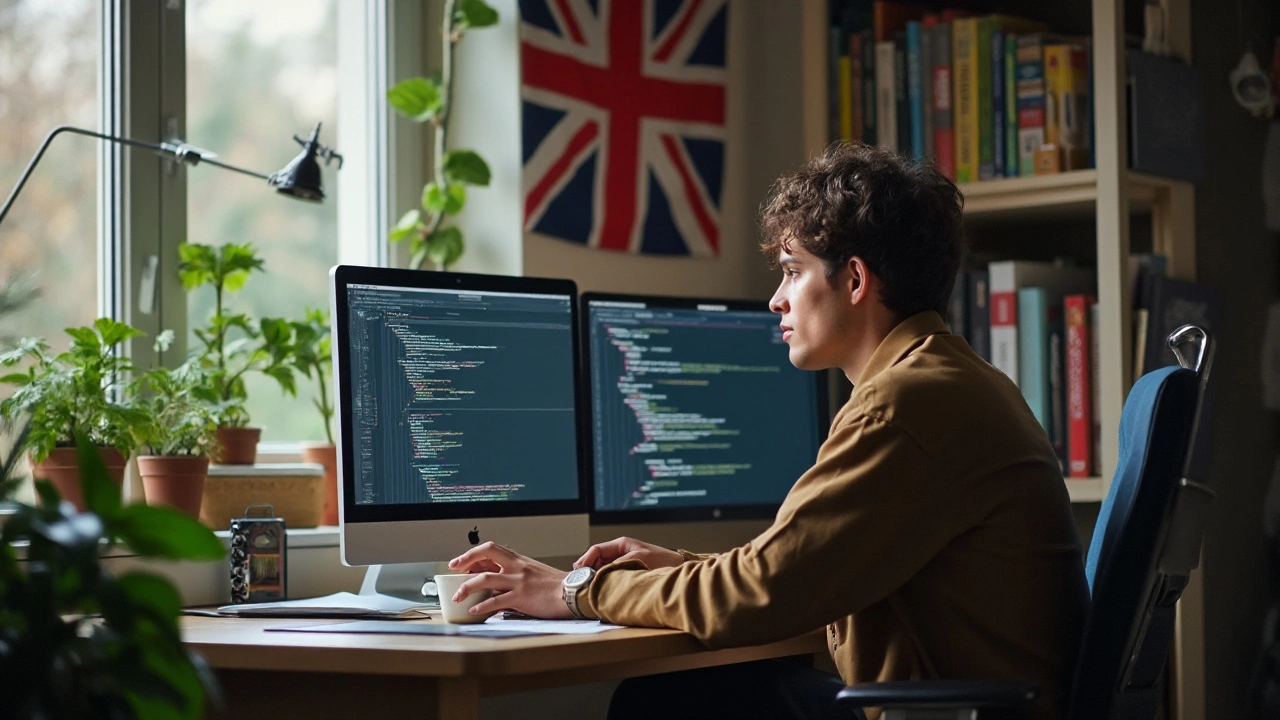
Diving into the world of programming, the first roadblock most beginners encounter is debugging. It feels baffling when your code doesn't work as expected, but don't worry; debugging is a skill you can develop. In this guide, we’ll explore essential techniques and tools to make your debugging process less daunting and more efficient.
Understanding common errors is a crucial step. Knowing the types of errors you might face—syntax errors, logic errors, and runtime errors—can save you a lot of time. Each error type requires a different approach, and understanding these can be like having a map in an unknown city.
Effective debugging techniques make a world of difference. From the classic 'print statement’ to modern integrated development environments (IDEs) offering powerful debugging features, choosing the right technique can significantly streamline your work.
Popular debugging tools are your best friends. Tools like Chrome DevTools, PyCharm, and Visual Studio Code can help identify and fix errors swiftly. Learning these tools will arm you with the capability to tackle complicated bugs.
Best practices for debugging can turn you from a frustrated novice into a confident problem-solver. Simple habits like writing clear code, regularly saving your work, and not being afraid to take breaks can have a big impact.
Finally, real-world examples will bring theory into practice. We'll walk through common scenarios and demonstrate how these techniques and tools can be used to troubleshoot real problems. By the end, you’ll have a solid foundation to start debugging your code with confidence.
- Understanding Common Errors
- Effective Debugging Techniques
- Popular Debugging Tools
- Best Practices for Debugging
- Real-World Examples
Understanding Common Errors
One of the first hurdles a beginner programmer faces is understanding what went wrong when a program doesn't work. The more you know about common errors, the easier it becomes to fix them. There are three primary types of errors that even experienced developers encounter regularly: syntax errors, logic errors, and runtime errors.
Syntax errors are mistakes in the code's structure. When the programming language's rules are not followed, you'll see syntax errors. These errors are relatively easy to spot since most IDEs and text editors underline or highlight them. Imagine you're writing a sentence but forgetting to put a period at the end. That’s a syntax error in programming terms. Misspelled keywords, missing semicolons, or unmatched parentheses are common culprits. Though they seem trivial, they can halt your code from running completely.
Logic errors are a bit more tricky. These occur when your code runs without crashing but doesn't do what you intended. It's like following a recipe perfectly but ending up with a burnt cake. For instance, you might be using a loop to sum numbers but accidentally subtract rather than add. Logic errors require a good understanding of your code's flow to catch.
Runtime errors are the third common type, often making an appearance only when the program is running. They might be easy to miss during the writing phase but can cause your program to crash. Examples include dividing a number by zero, trying to access a non-existent file, or running out of memory. Knowing how to handle runtime errors will make your code more robust and user-friendly.
“Programming today is a race between software engineers striving to build bigger and better idiot-proof programs, and the Universe trying to produce bigger and better idiots. So far, the Universe is winning.” – Rick Cook
Recognizing these errors is the first step, but maintaining good coding habits can reduce their frequency. Writing clean and well-documented code helps make the debugging process easier. Use meaningful variable names, stick to consistent formatting, and comment your code where necessary. These practices might seem like they slow you down, but they pay off when you encounter bugs.
Understanding the types of errors will not only help you fix them but also prevent them. Developing a practice of systematic debugging will make you a more efficient programmer. Remember, even the best programmers in the world make mistakes too, but what sets them apart is how efficiently they debug and learn from those errors. As you grow more familiar with the common pitfalls in your code, you'll find debugging to be less of a chore and more of a learning experience.
Effective Debugging Techniques
Debugging can sometimes seem like a daunting task, but by applying the right debugging techniques, you can make the process much smoother. Let's dive into some of the most effective strategies you can use to solve those pesky coding problems.
1. Print Statements
The simplest method to debug is by using print statements. Insert print statements in your code to display variable values and program flow at different stages. This technique might seem rudimentary, but it’s incredibly effective and widely used by both beginners and seasoned programmers. It helps you understand where the code is going wrong and why certain variables are not behaving as expected.
2. Commenting Out Code
Another useful technique is commenting out sections of your code to identify the source of the problem. By isolating different parts, you can quickly pinpoint where the error occurs. This method not only helps in finding bugs but also aids in understanding the flow and structure of your code.
“An important aspect of debugging is the skill to go like a doctor from symptom to root cause.” — Brian W. Kernighan
3. Using an Integrated Development Environment (IDE)
Modern IDEs come with built-in debugging features that can make a world of difference. Tools like breakpoints and watches allow you to pause the execution of your program and inspect variables in real-time. Some popular IDEs equipped with excellent debugging tools include Visual Studio Code, PyCharm, and Eclipse. These tools can automatically highlight syntax errors and offer suggestions, thus making the debugging process more efficient.
4. Rubber Duck Debugging
This quirky technique involves explaining your code, line by line, to an inanimate object like a rubber duck. It sounds funny, but voicing your thought process can often lead to a sudden realization of where you've gone wrong. It’s a method that capitalizes on the cognitive benefits of teaching and often unveils issues that you might have overlooked.
5. Using Linters
Linters are tools that automatically analyze your code for potential errors and stylistic issues. They can catch issues before you even run your program, saving you time on troubleshooting. Popular linters include ESLint for JavaScript, PyLint for Python, and RuboCop for Ruby. Employing linters can dramatically improve your debugging efficiency by pointing out mistakes early in the development process.
6. Divide and Conquer
When facing a complex bug, it’s often helpful to break your code into smaller, more manageable sections. Address each section individually to narrow down the source of the problem. By isolating smaller bits of code, you can methodically check their functionality and identify where things go wrong. This technique is particularly useful in larger projects with several interconnected components.
7. Online Forums and Communities
Never underestimate the power of a good community. Platforms like Stack Overflow and GitHub Discussions are treasure troves of information, where you can find solutions to similar problems or ask for help. Sometimes describing your issue to others can lead to new insights and solutions. Engaging with a community not only helps in solving the current problem but also broadens your knowledge base.
By incorporating these techniques into your workflow, you'll find that debugging becomes less of a chore and more of a learning experience. The next time you encounter an error, remember these tips, and you’ll be better equipped to tackle any coding challenge that comes your way.
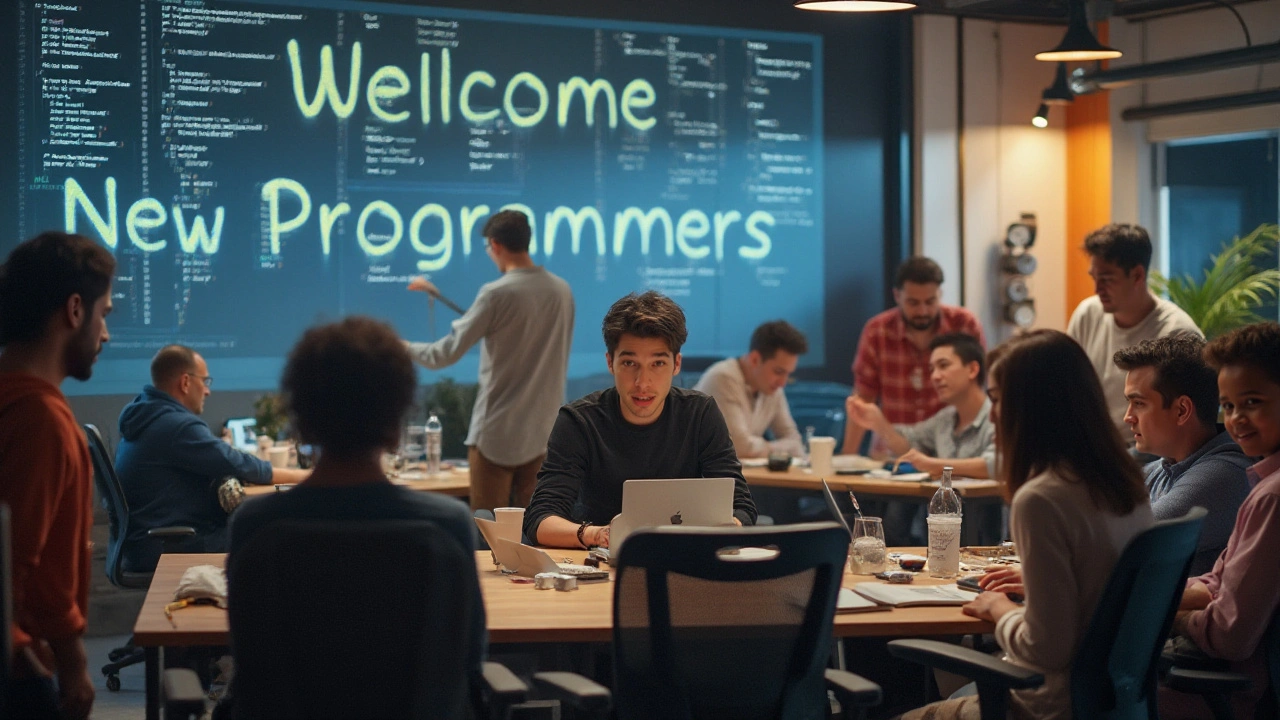
Popular Debugging Tools
When you’re just starting to code, choosing the right debugging tools can make a world of difference. The right tools not only help you identify bugs but also assist you in understanding why things go wrong, helping you become a better programmer in the process. Let's dive into some of the most popular debugging tools that are indispensable for any beginner.
Chrome DevTools tops the list, especially if you are working on web applications. It offers a rich suite of tools to inspect, debug, and profile your web application. You can access it by simply pressing F12 or right-clicking an element and selecting 'Inspect.' One standout feature is the ability to set breakpoints in your JavaScript code to inspect various states and variables without flooding your code with console.log statements.
Cate Huston, a notable software engineer, once said, “Chrome DevTools is like a magnifying glass for your web applications. If you want to ship great software, you need to learn how to use it.”
PyCharm is another fantastic tool, especially if you’re developing in Python. Used by professionals and beginners alike, PyCharm’s debugging capabilities are top-notch. You can set breakpoints, step through your code one line at a time, and even evaluate expressions at runtime. These features help you understand not just where your code fails but how it flows. Its integration with various Python testing frameworks like pytest makes it incredibly easy to debug tests.
Another essential tool is Visual Studio Code (VS Code). It's lightweight yet powerful, supporting multiple programming languages and frameworks. The built-in debugger works seamlessly across different languages. By adding extensions, you can tailor its functionality to suit your needs. For instance, the Python extension in VS Code turns it into a robust environment for Python development, complete with capabilities for debugging, testing, and code navigation.
For those working with server-side applications, GNU Debugger (GDB) is a classic tool that's been around for decades. Although it may not have the most visually appealing interface, it’s incredibly powerful. GDB lets you see exactly what’s going on in your program at any moment in your code. You can inspect variables, set breakpoints, and control the execution flow, making it invaluable for debugging more complex applications written in C and C++.
Lastly, let’s not forget about Postman if you’re dealing with APIs. It simplifies the process of developing APIs by allowing you to send requests and view responses with ease. Postman’s interface is intuitive, making it easy for beginners to get started. You can save and organize API requests, making the testing process smoother and more efficient.
Using these tools will not only help you fix errors faster but also understand your code's behavior better. While there are many tools available, these are particularly well-suited for beginners. They offer a good balance of functionality and ease of use, making the debugging process less intimidating and more manageable.
Best Practices for Debugging
Debugging can often feel like solving an elaborate puzzle, and having some best practices up your sleeve can make this process much smoother. These practices aren't just about finding and fixing bugs; they're about making your debugging process efficient and painless.
First, always write clear and readable code. It might seem like a no-brainer, but this practice will save you hours in the long run. Descriptive variable names, logical flow, and consistent formatting can make your code almost self-documenting. When your code reads like a simple narrative, finding the errors becomes less of a hunt.
Next, version control is your friend. Tools like Git allow you to keep track of every change. This way, if something breaks, you can roll back to the last working state without going through lines of code trying to remember what you changed. It's like having an undo button for your entire project.
Logging is another essential practice. Implementing comprehensive log statements helps you track the flow of your application and understand what went wrong. Logs can tell you what the program was doing right before it crashed or started behaving unexpectedly. Imagine having a CCTV capturing every action of your app; that's what logs can do for you.
Don’t rush through your code; take your time to study it. Sometimes, stepping away from your screen and taking a break can work wonders. Your brain continues processing problems in the background, and you might return with a fresh perspective that makes finding the bug much easier. Albert Einstein once said, "It's not that I'm so smart, it's just that I stay with problems longer."
Taking breaks gives your brain the opportunity to make those connections you might miss when you're too close to the problem.
Testing small sections of your code independently can also be incredibly helpful. Known as unit testing, this practice lets you isolate and verify different parts of your application. By focusing on small, manageable chunks, you can quickly identify where things are going off track.
Reproducing the error consistently is incredibly valuable. If you can make the problem happen on demand, solving it becomes a lot easier. It involves creating the exact conditions under which the bug appears. Think of it like an experiment where you control the variables to observe the outcome.
Finally, don't ignore the wealth of resources available online. Platforms like Stack Overflow, GitHub, and various programming communities are gold mines for debugging tips and solutions. Often, someone else has faced the same issue and can offer insight or even a solution.
Summary
Implementing these debugging practices can turn the process from a frustrating ordeal into a rewarding challenge. With clear code, version control, comprehensive logging, taking breaks, unit testing, reproducing errors, and leveraging online resources, you'll be well on your way to becoming a debugging pro.
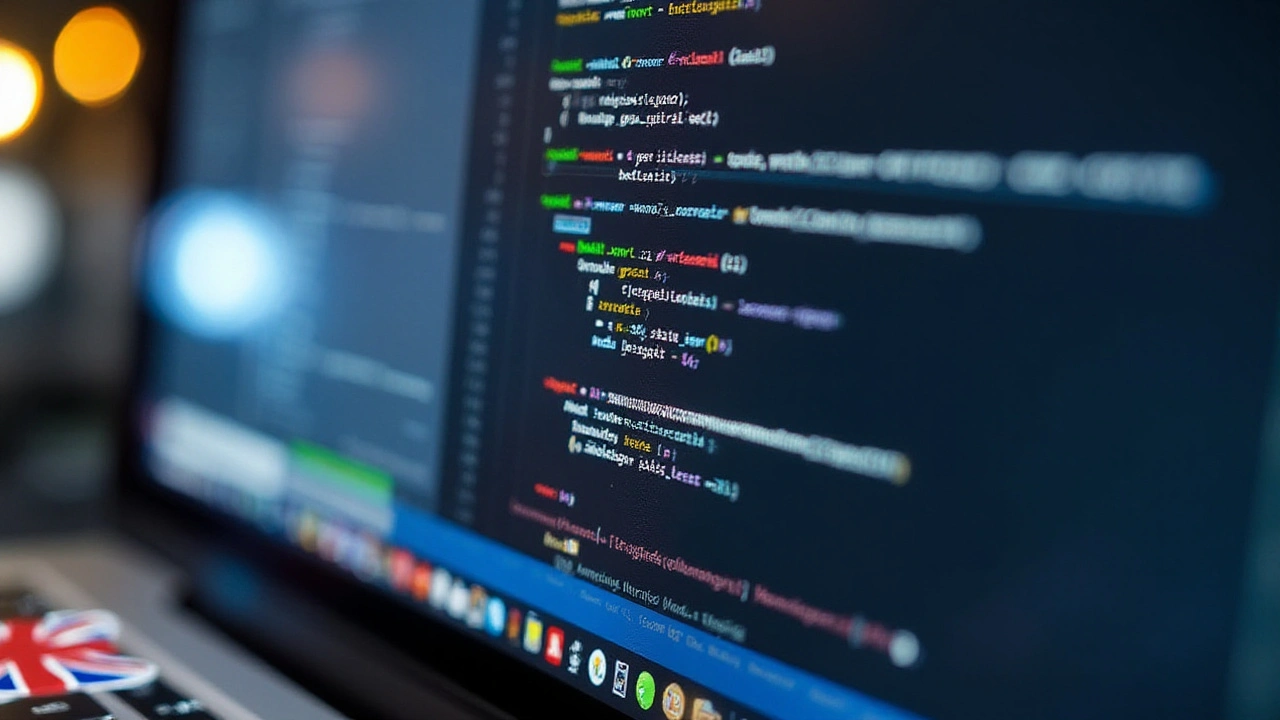
Real-World Examples
To make the process of debugging more tangible, let's walk through some real-world examples. These instances will show how to tackle common coding problems using effective debugging techniques and popular debugging tools.
One common issue beginners face is the infamous null pointer exception. Imagine you’re working on a Java application, and suddenly, your program throws a null pointer exception. This error often occurs when you try to access an object or call a method on a reference that is not pointing to any object. The first step is to locate where the exception is thrown. Using an IDE like Eclipse or IntelliJ IDEA, you can set breakpoints in your code and run the program in debug mode. As you step through the code, observe the variables and their values. This will help you pinpoint the exact location and cause of the exception.
For our second example, consider a situation where your web application is not displaying data as expected. Suppose you're using JavaScript to fetch data from an API but the data is not showing up. Here, a tool like Chrome DevTools becomes invaluable. Open Chrome DevTools by pressing F12 and navigate to the Network tab. Reload your page and observe the network requests. You might notice a failed request or incorrect data being returned. These insights can guide you to the part of your code that needs fixing, whether it's an issue with the API endpoint or how the data is being processed on the frontend.
“The greatest enemy of knowledge is not ignorance, it is the illusion of knowledge.” — Daniel J. Boorstin
Let's talk about a classic beginner's error: infinite loops. These can make your program hang, leaving you frustrated. Suppose you're coding in Python, writing a loop to iterate over a list. But instead of terminating, the loop goes on indefinitely. The best way to debug this is by using print statements strategically. Insert a print statement inside the loop to display the current value of your loop variable. You might find that your loop condition is always true due to a logic error. Fixing that condition will resolve your issue.
In many projects, managing dependencies can be challenging, especially when using package managers like npm for JavaScript or pip for Python. Imagine encountering a version conflict error while installing a new package. To debug this, check your project's dependency list in your package.json or requirements.txt file. Running `npm ls` or `pip list` will show all installed packages and their versions. Identifying and updating the conflicting packages often resolves these errors.
Another real-world scenario involves debugging database queries. Suppose your SQL query is running very slowly, affecting your application's performance. Use tools like PostgreSQL's EXPLAIN command to analyze the query execution plan. This command provides details about how the database executes your query, highlighting inefficient parts. By optimizing those, like adding appropriate indexes, you can improve query performance significantly.
Lastly, let’s explore an example where debugging goes beyond code – dealing with environmental issues. Imagine your application works fine on your machine but fails in production. Differences in configuration, missing environment variables, or different software versions can cause these issues. Using a tool like Docker to containerize your application ensures consistency across different environments. Containers encapsulate your application and its dependencies, making it easier to reproduce and debug any problems.
These real-world examples illustrate that with the right techniques and tools, you can effectively debug and resolve a wide range of issues. The key is to stay methodical and patient, breaking down the problem step by step until you reach a solution.