Programming Tricks to Achieve Coding Mastery
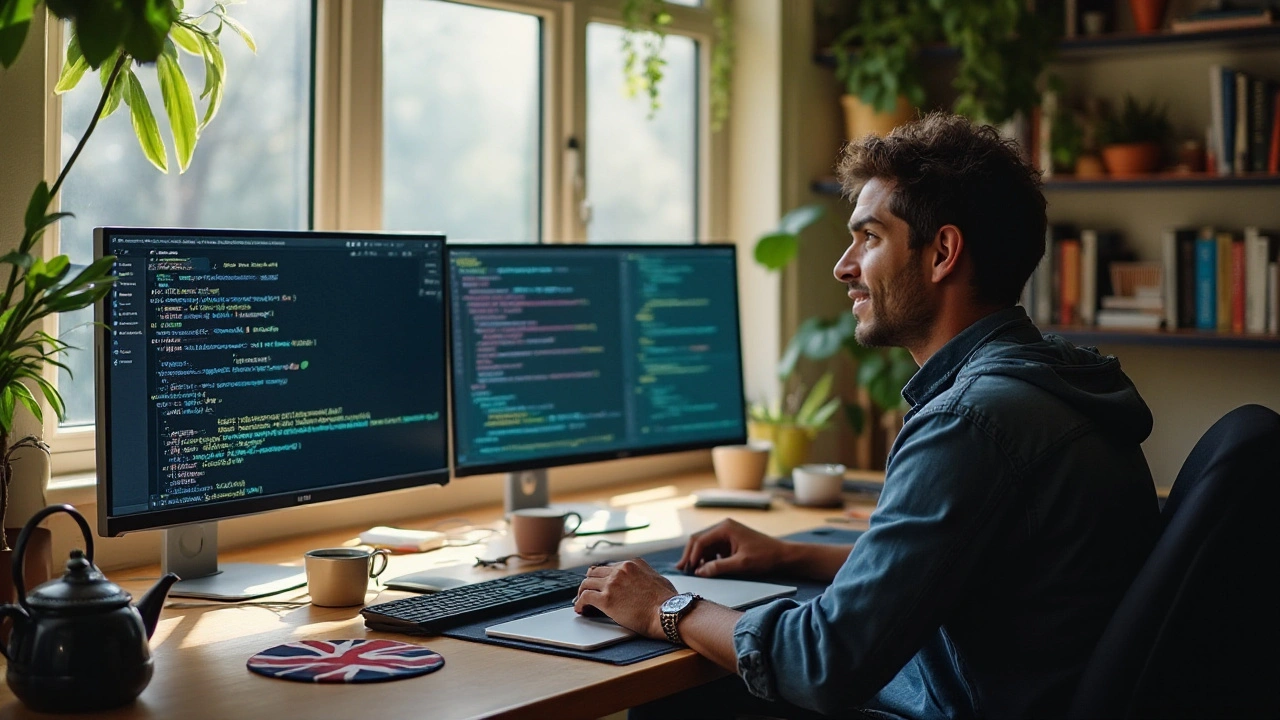
Have you ever felt overwhelmed by the complexities of coding? You're not alone. Whether you're just starting out or have been coding for years, there are always new tricks to learn that can make the process smoother and more efficient.
In this article, we're diving into some of the most effective programming tricks that can set you on the path to coding mastery. These tips aren't just for beginners; even seasoned developers might find a nugget of wisdom they've overlooked. From writing clean code to staying updated with the latest trends, these tips will make your coding life easier and more enjoyable.
- Understanding Clean Code Principles
- Mastering the Art of Debugging
- Optimizing Performance
- Staying Updated with Latest Trends
Understanding Clean Code Principles
Writing clean code is the foundation of effective software development. But what exactly does it mean for code to be clean? Simply put, clean code is easy to read, maintain, and extend. One of the most crucial aspects of clean code is its readability; if another developer can pick up your code and understand it without a hassle, you've done a good job. A well-known principle here is the KISS principle—Keep It Simple, Stupid. This means you should avoid unnecessary complexity and aim for simplicity in your solutions.
One of the most famous advocates of clean code is Robert C. Martin, also known as Uncle Bob. He highlights in his book “Clean Code: A Handbook of Agile Software Craftsmanship” that clean code should be elegant and efficient. Uncle Bob mentions that every piece of code can be improved, and refactoring should be a continuous process. He notes,
“Clean code always looks like it was written by someone who cares.”This belief underscores the importance of taking pride in your work and striving for excellence.
Consistency plays a vital role in clean code. Whether it's naming conventions, indentation, or comment styles, consistency helps other developers navigate your codebase more effortlessly. When naming variables, functions, and classes, take the time to choose descriptive names. For example, instead of naming a variable 'x,' opt for a name like 'userAge.' This makes it immediately clear what the variable represents.
Another critical aspect is minimizing dependencies. Dependencies can make your code more complex and harder to maintain. The goal is to write decoupled modules that can function independently. This enhances modularity and makes your code more versatile. Dependency Injection (DI) is a common technique to manage dependencies efficiently.
Comments are often a contentious topic in the world of clean code. While comments can be helpful, the aim should be to write self-explanatory code that minimizes the need for comments. If you find yourself repeatedly adding comments to explain what the code does, it might be a signal that your code needs refactoring to become more readable.
Adopting Test-Driven Development (TDD) can also lead to cleaner code. With TDD, you write the tests for a function before writing the function itself. This approach can help to clarify the function's purpose and catch bugs early. It also forces you to think through the design of your code before diving into implementation.
Here's a quick overview of key principles for writing clean code:
- Keep your functions small and focused on a single task.
- Use meaningful names for variables, functions, and classes.
- Favor readability over cleverness.
- Write comments only when necessary.
- Avoid code duplication.
- Refactor your code regularly.
- Test your code thoroughly.
Lastly, always remember that clean code is a journey, not a destination. Regularly revisiting and refining your codebase will help you to continually improve both your coding skills and the quality of your software. As you progress in your coding career, these principles will help you to write more effective, maintainable, and high-quality code.
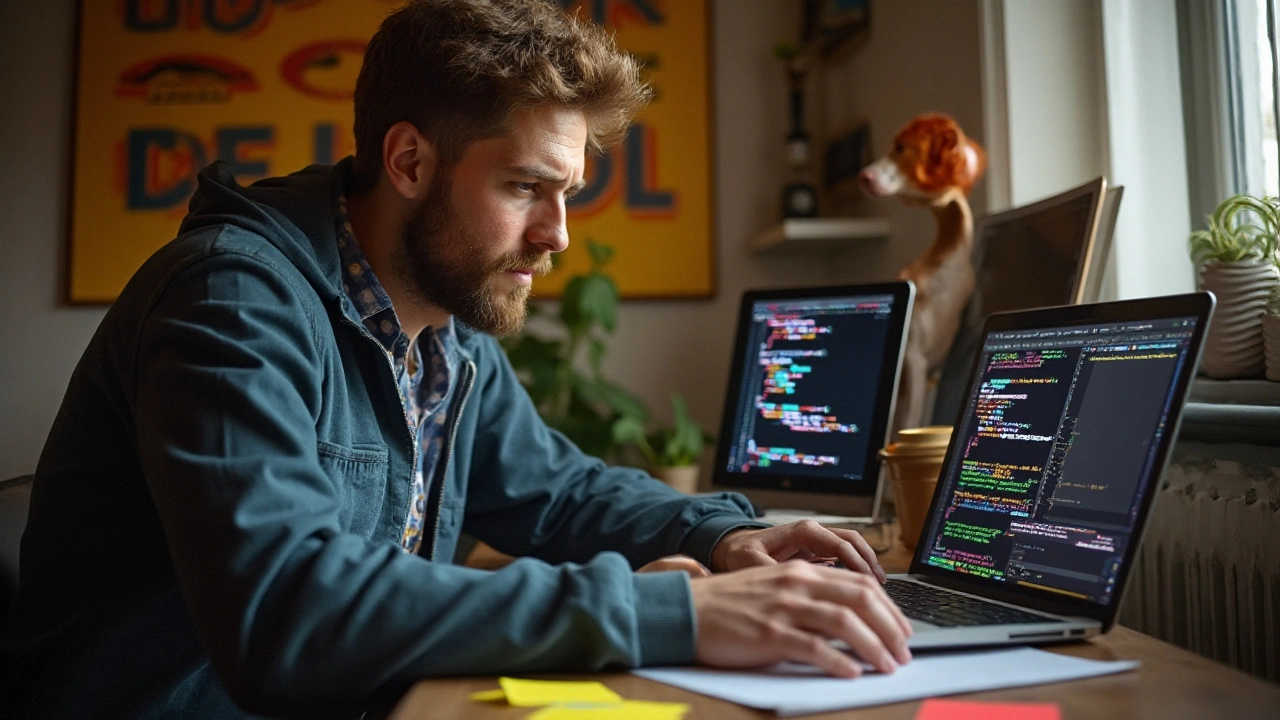
Mastering the Art of Debugging
Debugging can often feel like an art form rather than a science. It's the process of finding and fixing bugs, or errors, in your code. The approach you take can significantly influence how efficient and effective you are. A good starting point? Understanding your codebase through and through. This familiarity turns your coding problems into solvable puzzles rather than unsolvable mysteries.
An essential first step in debugging is to recreate the bug. You can't fix what you can't see. Identifying the exact conditions that lead to the bug can save an enormous amount of time. This often means testing various scenarios until you pinpoint the one that causes the issue. Once you've identified the bug, you can begin examining the specific segment of code responsible for it.
Using a debugger tool is incredibly helpful. Most integrated development environments (IDEs) come with built-in debuggers that allow you to step through your code line by line, monitoring variable values and program states. This granular level of control can make the debugging process far less stressful. Popular examples include Visual Studio Code for JavaScript and PyCharm for Python. These tools offer rich features that can help you quickly identify and solve issues.
Another useful approach is adding logging statements to your code. Simple print statements can help you track the flow of your program and see exactly where things are going wrong. Logging libraries like 'log4j' for Java or 'logging' in Python provide more advanced features, allowing for various levels of logging like debug, info, and error. This detailed insight can simplify the debugging process.
Software development isn't a solitary task; pair programming can significantly help in debugging. Two pairs of eyes are often better than one. Engaging with a colleague to review problematic code can offer fresh perspectives and solutions you might not have considered. External viewpoints are invaluable, especially when you are stuck on a particularly challenging bug.
"Ninety percent of the time, debugging a problem translates to finding and understanding the piece of code that might have gone wrong." - Robert C. Martin.Prioritize good coding tricks and practices to minimize bugs from the get-go. Writing clean, modular code makes it easier to identify issues. Follow a consistent coding standard throughout your application and avoid convoluted logic that can lead to hard-to-find bugs. Meaningful variable names and comments can make a world of difference when you're deep in the trenches of debugging.
Error messages are your friends. They often tell you precisely what's gone wrong, whether it's a syntax error or a logical flaw. Don't just skim over them; read and understand each error message you encounter. Search forums, documentation, and online communities like Stack Overflow to see if others have encountered similar issues.
Lastly, always stay patient and methodical. Debugging can become frustrating, but approaching it with a systematic mind can make the process smoother. Break down the problem into smaller, manageable pieces, and tackle each one step by step. Doing so not only helps in solving the current issue but also builds your debugging skills for future challenges.
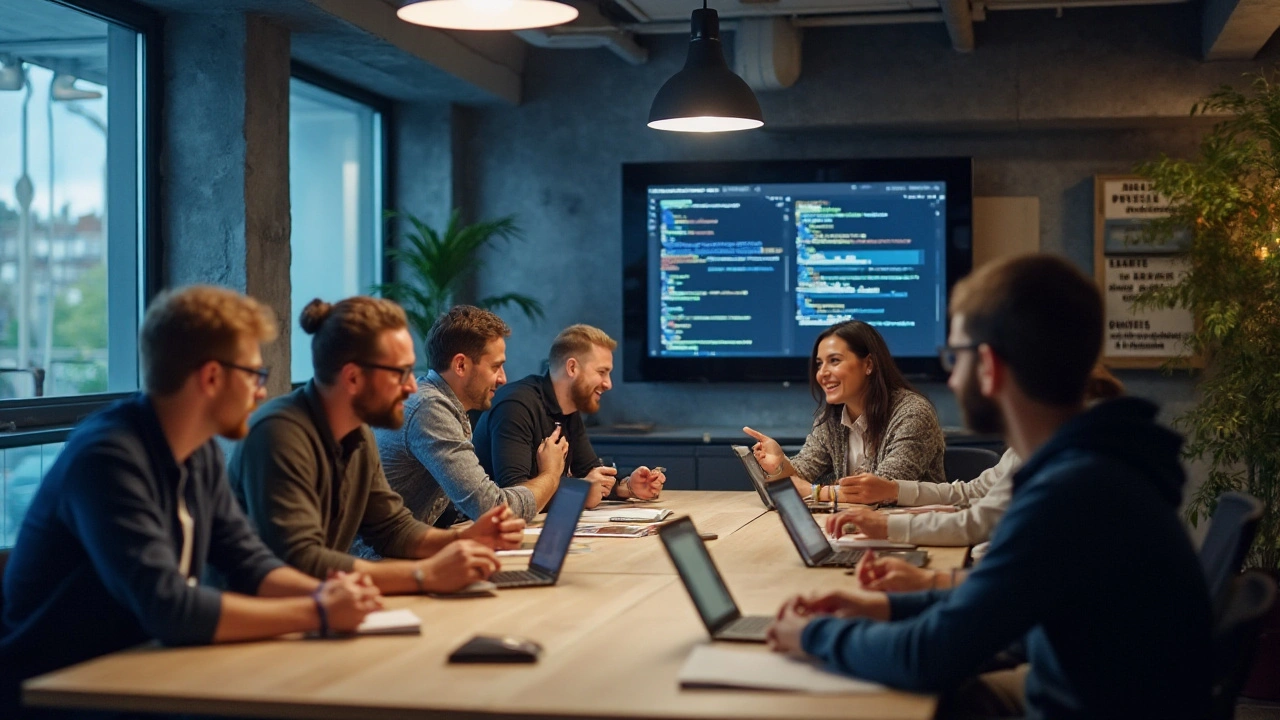
Optimizing Performance
When it comes to programming, one of the most vital elements is ensuring that your code runs efficiently. Optimizing performance isn’t just about making things faster; it’s about using resources wisely and ensuring your software functions smoothly under various conditions. This is especially crucial in today's world where users expect quick responsiveness from every application they use.
One of the first steps in optimizing performance is understanding the bottlenecks in your code. Profiling tools are invaluable here. They help you identify which parts of your code are taking the most time to execute. By focusing on these areas, you can make significant improvements without having to overhaul your entire system. Tools like Visual Studio's Profiler or Python’s cProfile are excellent places to start.
Another fundamental tactic is improving your algorithms and data structures. Often, the choice of algorithm can make a substantial difference in the performance of your application. For example, using a binary search instead of a linear search can drastically reduce search times. Data structures like hash tables offer average time complexity of O(1) for search operations, making them extremely efficient for large datasets.
Memory management is another critical area for optimizing performance. Ensure that you are not holding onto memory longer than necessary. Languages like C++ offer fine control over memory allocation, but even higher-level languages like Java and Python benefit from mindful memory management. Garbage collection can introduce pauses or delays in an application, so knowing how to manage these processes can be very helpful.
Parallelism and concurrency can also significantly enhance performance. Leveraging multiple cores of a processor through multithreading or multiprocessing can lead to faster execution times. However, it is crucial to handle these with care to avoid issues such as race conditions or deadlocks. Languages like Go and Python have robust concurrency models, making it easier to implement parallelism without much hassle.
As highlighted by Donald Knuth, a respected computer scientist, "Premature optimization is the root of all evil."
Let performance optimization be a part of your later stages in the development cycle so you don’t compromise the readability and maintainability of your code early on.It's essential to strike a balance between optimizing performance and maintaining clean, understandable code. This principle ensures that your code remains flexible and adaptable to future changes.
Tips for Better Optimized Code
Here are some handy tips to keep in mind:
- Minimize I/O operations: Disk and network I/O are much slower than in-memory operations. Batch your reads and writes where possible.
- Avoid redundant calculations: Store the results of expensive operations if they are going to be used multiple times.
- Use lazy loading: Don’t load data until it’s needed. This can save a lot of memory and processing power.
- Profile before and after: Always measure the performance before and after making changes to know if your optimizations are making a difference.
- Cache wisely: Caching can greatly enhance performance but ensure cache eviction policies are effectively managed.
Even the best developers continuously learn new ways to optimize their code. Staying up-to-date on the latest trends and technologies can provide fresh insights and techniques to ensure your applications run at peak efficiency. By focusing on optimizing performance, you can create more responsive, reliable, and enjoyable software.
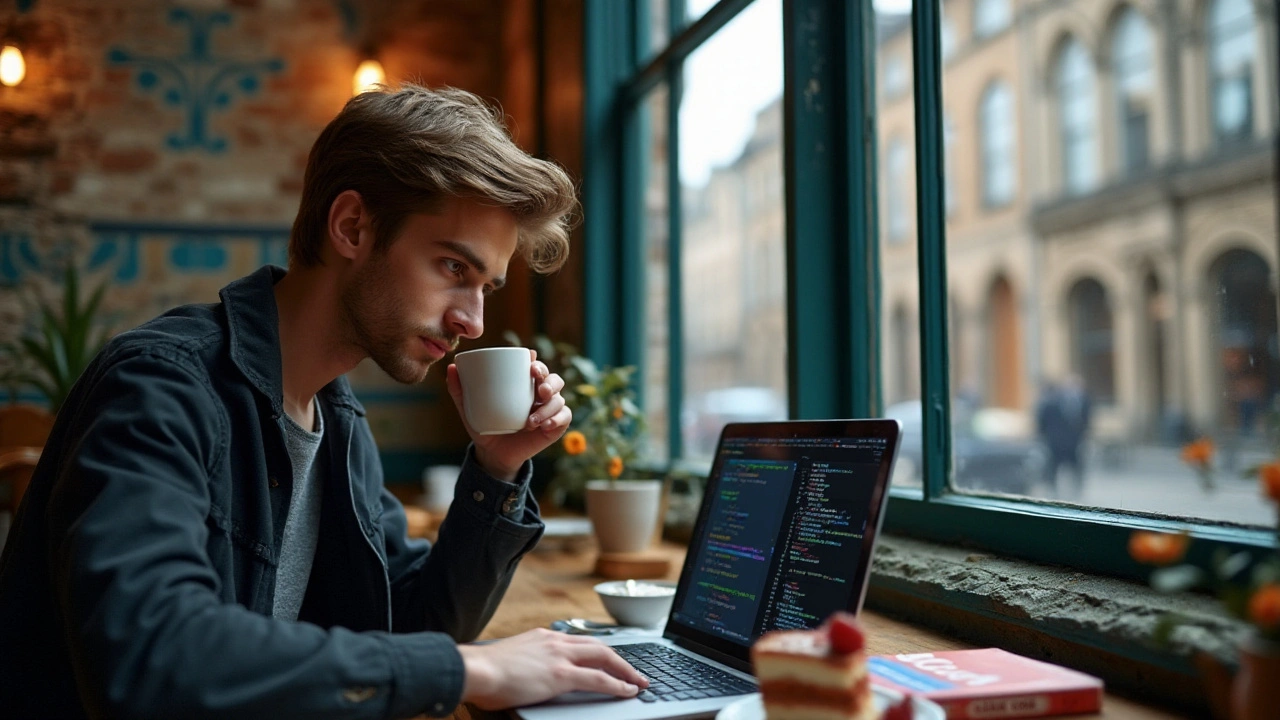
Staying Updated with Latest Trends
In the fast-paced world of technology, staying updated with the latest trends can be challenging yet incredibly rewarding for developers. Given the rapid changes in the field, it is essential to keep learning and adapting to maintain an edge. One of the most effective ways to stay ahead is by engaging with reputable online communities and forums such as Stack Overflow, GitHub, and Reddit. These platforms are teeming with discussions from other developers who are constantly sharing insights, new tools, and best practices.
Another crucial method involves subscribing to industry newsletters and following trustworthy blogs. Websites like TechCrunch, Ars Technica, and Hacker News often post timely articles about the latest advancements and shifts in the tech world. Regularly reading these can provide a wealth of information and often highlight emerging trends before they become mainstream. Attending webinars and online courses is also beneficial. Websites like Coursera, Udemy, and edX offer a plethora of courses on various programming languages and technologies.
An often overlooked yet highly valuable resource is attending coding meetups and conferences. These events present an opportunity not only to learn but also to network with other professionals in the field. Platforms like Meetup.com frequently list such events, ranging from local coding bootcamps to international tech conventions. Don't underestimate the power of face-to-face interaction; it can lead to new opportunities and collaborations.
As Bill Gates once said, "The best way to be at the forefront is to continually learn and adapt."In addition to all of these, diving into open-source projects is another excellent way to stay current. Participating in or even just observing projects on platforms like GitHub can expose you to various coding styles, tools, and workflows that you might not encounter otherwise. It's a hands-on approach to learning that can be incredibly effective.
Utilizing social media smartly is another tool in your arsenal. Following key influencers on Twitter and LinkedIn can provide a steady stream of the latest information straight from industry leaders. These platforms also offer an opportunity to participate in relevant discussions, ask questions, and share your own insights. Just be mindful of information overload; it's important to filter your sources to retain valuable insights only.
Lastly, coding practice platforms like LeetCode, HackerRank, and Codewars are excellent for staying sharp and learning new tricks. These platforms offer a wide range of challenges that cover various aspects of programming, from algorithm puzzles to real-world tasks. Regularly solving such problems can improve your coding skills and introduce you to new techniques.