Mastering Programming: From Basics to Advanced Techniques
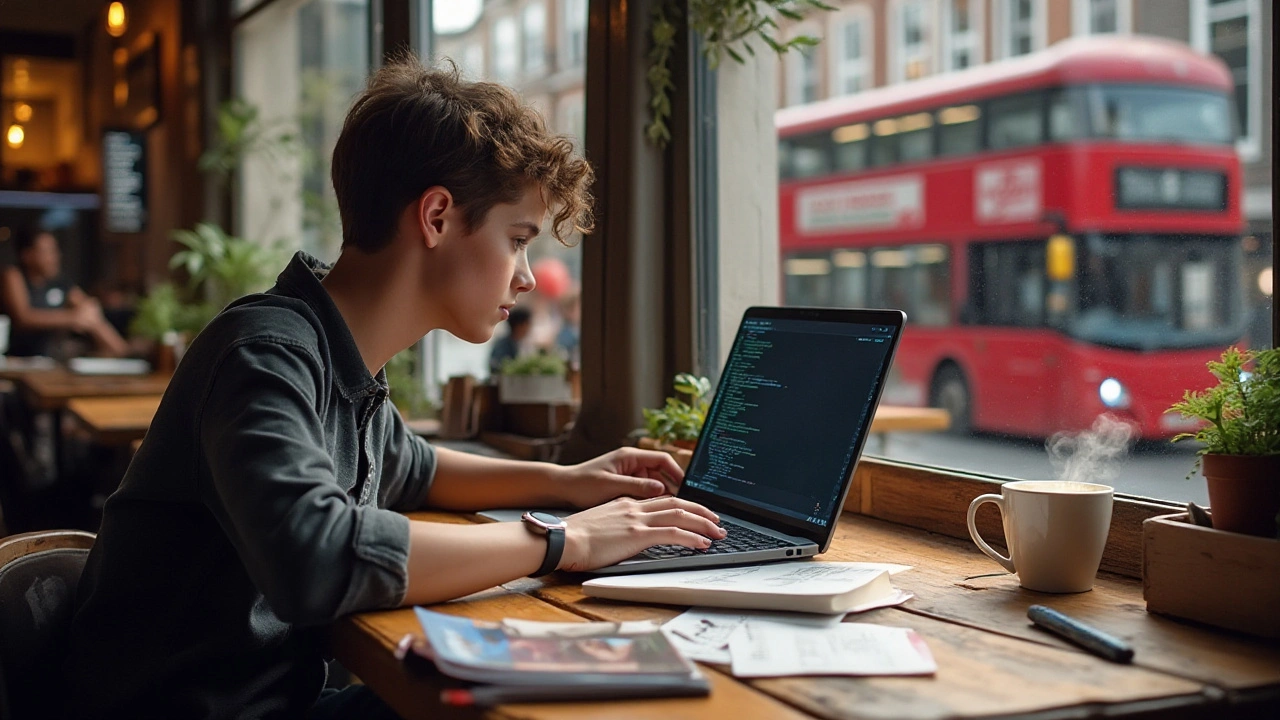
Programming can seem daunting at first, but with the right approach, anyone can master it. Whether you're just starting out or trying to scale your skills, programming opens up a world of possibilities. This article covers everything from setting up your environment to advanced techniques.
We'll start with basic programming concepts and gradually move on to more complex topics, offering practical advice and real-world examples along the way. So, buckle up as we embark on this interesting journey!
- Introduction to Programming
- Setting Up Your Environment
- Basic Programming Concepts
- Intermediate Techniques
- Advanced Programming Techniques
- Best Practices and Tips
Introduction to Programming
Programming, at its core, is about giving instructions to a computer to perform specific tasks. It's a skill that is incredibly valuable in today's digital age, and it forms the backbone of almost every modern technology we use. Whether it's your smartphone, the latest video game, or even the operating system on your computer—programming is what makes it all work.
The beauty of programming lies in its logic. If you can think logically and solve problems step-by-step, you're on your way to becoming an excellent programmer. One fascinating aspect of programming is its versatility. You can use it for a wide range of applications, from web development to software engineering, and even data analysis.
Learning programming languages like Python, Java, or JavaScript opens up numerous doors. Python, for instance, is known for its simplicity and readability, making it a great starting point for beginners. Java is extensively used in enterprise environments, while JavaScript adds interactivity to websites. According to the 2024 Stack Overflow Developer Survey, Python continues to be the preferred language for beginners because of its simplicity and the vast resources available for learning, such as tutorials and community support.
When you first begin programming, some concepts might seem confusing. Don't be discouraged. Even seasoned programmers face challenges and have to refer to documentation regularly. As the legendary computer scientist Donald Knuth once said,
“The process of preparing programs for a digital computer is especially attractive because it not only can be economically and scientifically rewarding, it can also be an aesthetic experience much like composing poetry or music.”
One of the best ways to learn programming is by doing. Start with small projects and gradually increase the complexity as you become more comfortable with the language. Online platforms like Codecademy and freeCodeCamp offer interactive projects and exercises that make learning engaging. Additionally, participating in coding challenges on websites like HackerRank or LeetCode can hone your skills further.
Another crucial aspect is understanding the development environment. Setting up a proper environment can make a massive difference in your programming journey. Tools such as Integrated Development Environments (IDEs) like VS Code or PyCharm can significantly enhance your productivity by providing code completion, debugging tools, and version control support.
More often than not, beginner programmers overlook the importance of writing clean, readable code. Always comment your code to explain what each part does. This practice is beneficial not only for others who might read your code but also for you when you look back at it after some time. Moreover, following naming conventions and keeping your code modular can immensely improve its readability.
In essence, anyone can become proficient in programming with dedication and practice. It's not reserved for geniuses or mathematics prodigies; it's for anyone willing to learn and explore. With the wealth of resources available today, it's easier than ever to start your programming journey. And as you advance, you'll realize that the potential applications of programming are virtually limitless.
Setting Up Your Environment
Before diving into the world of programming, setting up your environment is a crucial step. This typically involves selecting and installing software that makes coding easier and more efficient. Many beginners make the mistake of overlooking this step, but professionals understand its significance. The right environment can save you hours of headache and streamline your workflow.
First things first, you'll need a computer with a reliable internet connection. While almost any modern computer can be used for programming, having a machine with at least 8GB of RAM and a decent processor is advisable. These specifications ensure you don’t experience lag, especially when running more demanding applications or developing complex projects.
Next, you will need a text editor or an Integrated Development Environment (IDE). Tools like Visual Studio Code, Sublime Text, and Atom are popular for their user-friendly interfaces and powerful features. Many programmers prefer Visual Studio Code because it is open-source, customizable, and supports a wide range of programming languages.
Once you've chosen an editor or IDE, the next step is to install any necessary compilers or runtimes. If you're working with languages like C++ or Java, you'll need a compiler compatible with your system. For web development, you might need Node.js and npm (Node Package Manager). These tools help manage libraries and dependencies, making your life much easier.
Some developers find it helpful to use version control systems like Git. Git lets you track changes in your code, collaborate with others, and even roll back to previous versions if something goes wrong. Platforms like GitHub and GitLab provide hosting services for Git repositories and offer additional features for collaboration and workflow management.
It's also wise to set up a virtual environment, especially if you are working with multiple projects that require different dependencies. Tools like Virtualenv for Python and Docker for containerized environments can keep your projects isolated, avoiding conflicts between libraries and packages.
“The sooner you automate the repetitive tasks in your environment setup, the closer you'll get to mastering software development,” advises Martin Fowler, a prominent author and software developer.
For those diving into specific areas like data science or mobile app development, some specialized software might be necessary. For instance, you may need Jupyter Notebooks for Python-based data science, or Android Studio for Android app development. Each of these tools comes with its own set of requirements and setup guidelines, which you should follow for the best results.
Lastly, make sure your environment is conducive to long coding sessions. Ergonomics matter—a comfortable chair, a good desk, and proper lighting can go a long way toward reducing physical strain and maintaining productivity. Some developers also use ergonomic keyboards and mice to further enhance their setup.
Remember, the goal is to make your programming environment as streamlined and efficient as possible. This means not just picking the right tools and software, but also organizing your workspace in a way that maximizes comfort and productivity. Setting up may seem like a trivial job, but it lays the foundation for effective and enjoyable coding sessions.
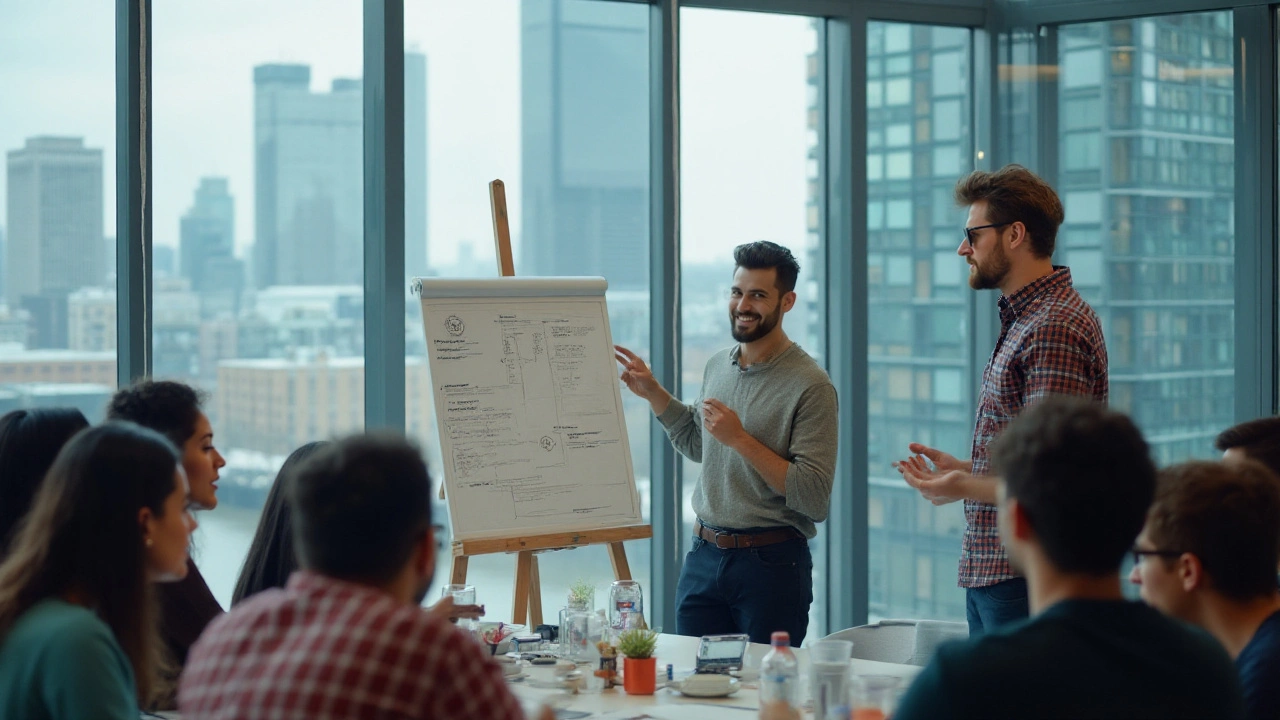
Basic Programming Concepts
Programming is built on fundamental concepts that form the backbone of any coding language. These basic concepts are essential for any aspiring programmer to grasp before diving into more complex topics. In this section, we will delve into variables, data types, control structures, functions, and more. By understanding these foundational elements, you set yourself up for success in your programming journey.
Let's start with variables. Variables are essentially storage containers for data values. They allow programmers to create a script that can manipulate data and produce dynamic results. For example, in Python, you can declare a variable like this:
"Variables are an important part of programming and allow you to create complex functions that can manipulate and store data dynamically. Mastering them is key to moving on to more advanced topics." - Python.org
name = "John"
age = 30
print(name, age)
The code above creates two variables, name and age, and prints their values. It's a simple example, but it illustrates how you can store and manipulate data using variables. The concept of variables is universal across all programming languages, although the syntax may be different.
Next, let's talk about data types. Data types define the kind of data a variable can hold. Common data types include integers, floats (decimal numbers), strings (text), and booleans (true/false). Understanding the different data types and how to manipulate them is crucial for coding efficiently. Here's a quick example in Python:
number = 10 # Integer
pi = 3.14 # Float
message = "Hello, World!" # String
is_active = True # Boolean
Each data type has its own set of operations and functions. For instance, you can concatenate strings or perform arithmetic operations on integers and floats. Misusing data types can lead to errors, so it's important to be mindful of what type of data you are working with.
Now, let's explore control structures. These are constructs that control the flow of execution in a program. Common control structures include if-else statements, loops, and switch-case statements. For example, an if-else statement allows a program to execute certain code only if a specified condition is true. Here’s how an if-else statement looks in Python:
if age > 18:
print("You are an adult.")
else:
print("You are a minor.")
Control structures are powerful tools that enable you to write efficient and effective programs. They help you manage complex logical flows and avoid repetitive code.
Finally, let's touch on functions. Functions are reusable blocks of code designed to perform a specific task. They help in breaking down complex problems into manageable pieces. In Python, you define a function using the def keyword:
def greet(name):
return "Hello, " + name + "!"
print(greet("Alice"))
The greet function takes a single argument, name, and returns a greeting message. By using functions, your code becomes cleaner and more organized, making it easier to debug and maintain.
To sum up, understanding these basic programming concepts—variables, data types, control structures, and functions—is crucial for any programmer. Having a strong grasp of these fundamentals will set you on the right path as you continue to learn and tackle more advanced topics.
Intermediate Techniques
Once you have a good grasp of basic concepts, it's time to explore intermediate techniques. These skills will help you build more complex and efficient programs, making your code more robust and scalable.
One important area to focus on is data structures. Understanding arrays, linked lists, stacks, and queues is crucial. These structures help you organize and store data efficiently. For example, arrays are useful when you need to quickly access elements by their index, while linked lists are better for scenarios where you frequently insert and delete elements.
Another essential topic is algorithms. Sorting algorithms like quicksort, mergesort, and heapsort are important because they affect the performance of your applications. Learning how to implement these algorithms and understanding their time complexity can make your programs run faster.
Object-oriented programming (OOP) is another critical skill at this level. This paradigm helps you organize your code into reusable components. Understanding concepts like classes, objects, inheritance, and polymorphism can dramatically improve your coding efficiency. For instance, OOP allows you to create blueprints for objects which can simplify large projects.
"The measure of a great developer is not how much code they can write, but how elegantly they can solve a problem." - Robert C. Martin
Error handling is often overlooked, but it's vital for creating reliable programs. Learn to use try-catch blocks and understand the types of errors you might encounter. This approach will help you build programs that can gracefully handle unexpected situations.
Moving on, APIs (Application Programming Interfaces) are essential tools for modern development. They allow different software systems to communicate with each other. Knowing how to interact with various APIs, whether for social media, payment gateways, or data services, is essential.
Version control systems like Git should also be on your radar. These systems help you keep track of changes in your codebase, making it easier to collaborate with others. Learning Git commands and understanding how to manage branches and merges will streamline your workflow.
If you are working on large projects, understanding design patterns can be invaluable. Patterns like Singleton, Factory, and Observer provide proven solutions to common problems. They help you write code that is easier to comprehend and maintain.
Additionally, learning about testing is crucial. Unit testing and integration testing ensure your code works as expected, which can save you hours of debugging down the road. Tools like JUnit for Java or PyTest for Python can be very helpful.
Finally, don't overlook the importance of documentation. Well-documented code is a sign of professionalism and makes it easier for others (and your future self) to understand your work. Tools like Javadoc or Doxygen can generate comprehensive documentation from your code comments.
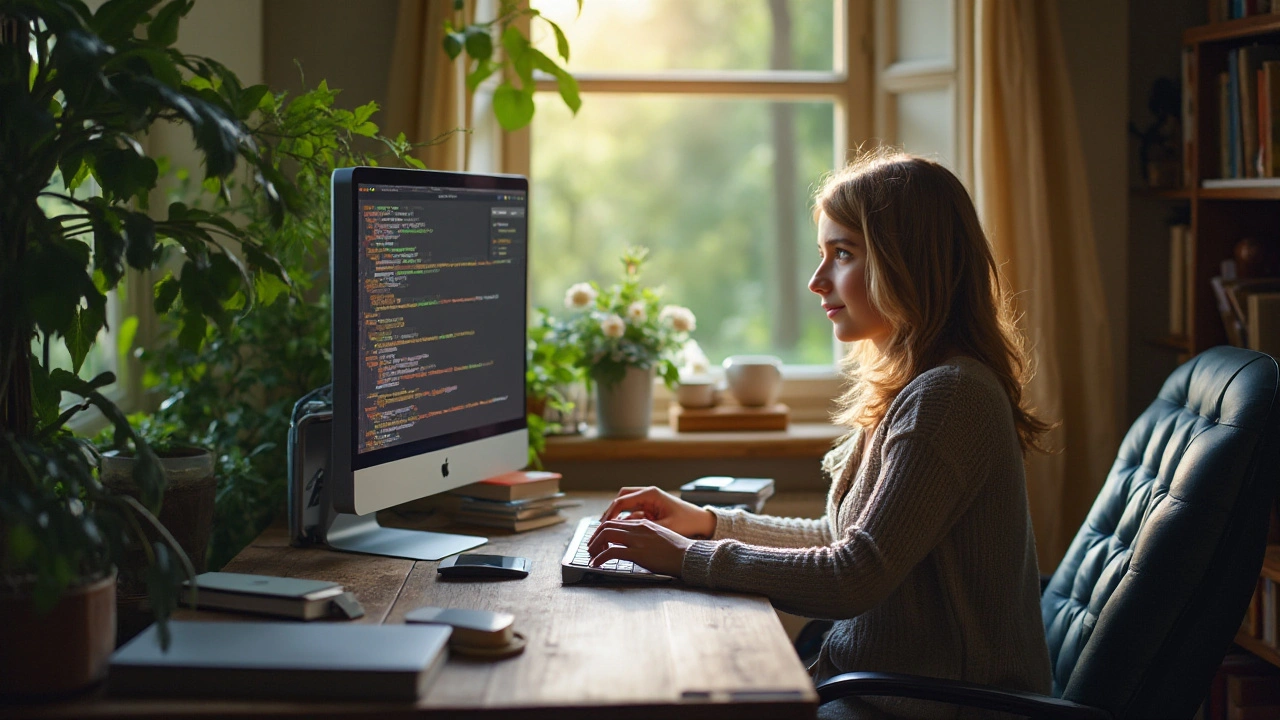
Advanced Programming Techniques
Once you’ve got a handle on the basics and started exploring intermediate concepts, it’s time to dive into advanced programming techniques. These methods don't just elevate your coding skills; they open up a new range of possibilities for project development. One such technique is learning about design patterns. Design patterns are tried and tested solutions for common software problems. They might seem abstract at first, but they can significantly streamline your coding process. For instance, the Singleton design pattern ensures a class has only one instance, which is particularly useful for managing shared resources in your applications.
Another crucial skill to master is understanding and implementing algorithms and data structures. While lists and loops can get you far, grasping more complex structures like trees and graphs will enable you to solve problems more efficiently. Think of Dijkstra's algorithm for finding the shortest path in a graph—this is fundamental for network routing and geographical mapping services. Similarly, learning sorting algorithms like QuickSort can drastically improve the performance of your code. As British computer scientist Tony Hoare once said, "There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies; the other way is to make it so complicated that there are no obvious deficiencies."
Concurrency is another area that separates advanced programmers from the rest. Modern applications often need to perform multiple tasks simultaneously, requiring a solid understanding of multi-threading and parallel processing. Mastering concurrency can lead to more robust and efficient applications, whether it's for web servers, video games, or data processing. Synchronous and asynchronous processing techniques are critical in managing tasks and resources in an optimal way.
Another technique worth mentioning is functional programming. While it may seem like a niche area, functional programming paradigms are gaining popularity for their ability to manage state and side effects cleanly. Languages like Haskell and Scala promote immutability, first-class functions, and higher-order functions, making your code more predictable and easier to debug.
Memory management is another crucial aspect of advanced programming. Understanding how memory allocation works, along with garbage collection mechanisms, is vital for creating efficient applications. Tools like Valgrind can help you detect memory leaks and optimize resource usage. This is particularly important in languages like C++ where manual memory management is required.
If you’re into web development, mastering advanced JavaScript frameworks like React or Vue can take your skills to the next level. These frameworks offer sophisticated features for creating dynamic, high-performance web applications. For server-side development, delve into Node.js with a focus on leveraging its asynchronous nature for building scalable network applications.
Lastly, let's not forget about testing and quality assurance. Implementing unit tests, integration tests, and employing test-driven development (TDD) ensures your code is reliable and maintainable. Continuous Integration (CI) and Continuous Deployment (CD) pipelines automate the testing process, reducing the chances of introducing bugs during updates. Employing code review practices and using tools like Git for version control can significantly improve the quality of your codebase.
Remember, advanced programming techniques are not just about writing complex code; it’s about writing efficient, readable, and maintainable code. As you continue to learn and apply these techniques, you'll find yourself becoming more confident and versatile in your coding abilities.
Best Practices and Tips
When it comes to programming, adopting best practices can significantly improve your productivity and code quality. One foundational practice is adhering to consistent code formatting rules. Using consistent styles makes your code easier to read and debug. Whether you're working in Python, Java, or JavaScript, different languages have their own standards. For instance, Python emphasizes readability with its PEP 8 style guide.
Another critical tip is writing comments and documentation. While it may seem tedious, good documentation ensures that you and others can understand your work later. Comments should be concise and explain the purpose of the code, not the mechanics. Excellent documentation also includes inline comments, docstrings, and README files.
Testing can't be stressed enough. Writing unit tests for your functions and methods helps catch bugs early and ensures your code behaves as expected. Automated testing frameworks like JUnit for Java or pytest for Python can facilitate the process. This makes your testing routine easier and more efficient.
One interesting fact is that experienced programmers often spend more time reading code than writing it. Keeping that in mind, make your code as clean and understandable as possible. Techniques like refactoring—rewriting existing code to improve structure without changing its behavior—can make a huge difference.
Effective use of version control systems such as Git is vital for managing changes to your codebase. Git allows you to track changes, collaborate with others, and revert to previous versions if something goes wrong. Platforms like GitHub or GitLab also provide a collaborative environment where team members can review each other's code.
According to a study conducted by Microsoft, developers who employ pair programming—where two programmers work together at one workstation—often produce higher-quality code. While one person writes the code, the other reviews each line of code as it’s written. This practice reduces errors and fosters better problem-solving strategies.
“Programs must be written for people to read, and only incidentally for machines to execute.” — Harold Abelson, computational thinking pioneer
Incorporate error handling in your code by anticipating potential issues and managing them gracefully. Robust error handling ensures that your program doesn't crash and provides useful feedback to the user. In Python, you can use try-except blocks, while Java offers try-catch mechanisms.
Security is often overlooked but is paramount. Ensure you write secure code by validating inputs, avoiding static passwords, and using secure protocols. Staying updated with the latest security practices can mitigate risks of vulnerabilities and attacks.
Finally, don’t underestimate the power of keeping up with the latest trends and technologies. The field of programming evolves rapidly. Staying current with new languages, libraries, and frameworks can open up new opportunities and keep your skills relevant. Engaging with the community through forums, attending webinars, or joining coding boot camps can provide continuous learning and inspiration.