Essential Python Tricks: A Must-Have Guide for Every Programmer
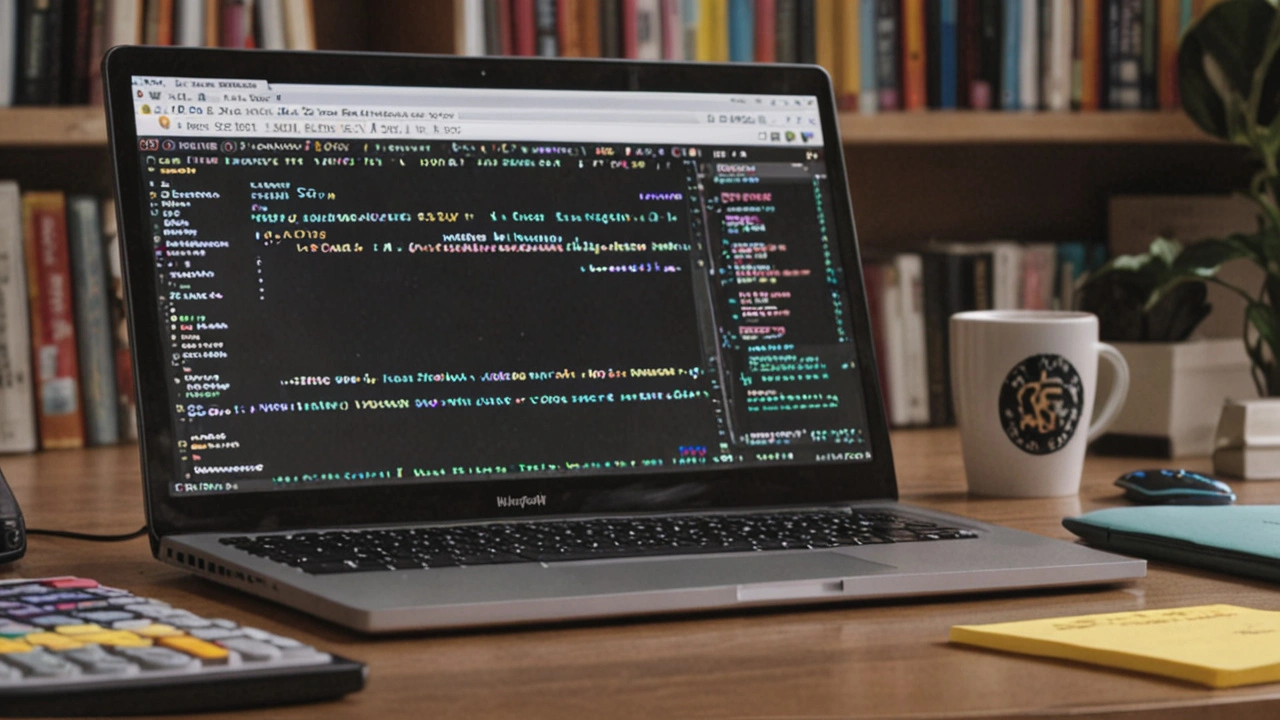
Diving into the world of Python can feel like opening the door to endless possibilities. Whether you're a seasoned developer or just starting out, there are always new tricks to learn that can make your coding journey smoother, faster, and more enjoyable.
This guide is designed to reveal those hidden gems – the practical tips and clever hacks that can transform the way you program in Python. From must-know functions to intriguing features, let's unlock the full potential of this versatile language.
- Introduction to Python Tricks
- Useful Functions and Methods
- Hidden Features and Shortcuts
- Practical Applications and Examples
Introduction to Python Tricks
Python isn't just a language, it's a toolkit that developers love for its simplicity and versatility. Whether you’re automating boring tasks, building robust web applications, or diving into data science, Python’s vast ecosystem has got you covered. But here’s something you might not know: with a few cool tricks, you can boost your efficiency and make your code not just work but shine.
One of the greatest things about Python is its readability. Guido van Rossum, the creator of Python, designed it to be readable by humans as plain English. This philosophy of simplicity is embedded in Python’s core, making it easier for developers to learn and implement new features quickly. But beyond the language’s structure, there are some techniques and features that even experienced programmers might overlook or not utilize fully.
Consider the 'Zen of Python,' a collection of aphorisms that capture the essence of what the language aims to achieve. If you type import this in a Python shell, you'll get a list of principles to guide you. Take, for example, “Simple is better than complex.” This principle pushes you to write clear, concise code that's easier to maintain in the long run. Keeping these principles in mind as you explore new tricks will ensure your code remains elegant and effective.
“A language that doesn't affect the way you think about programming is not worth knowing.” — Alan Perlis
One basic trick that can dramatically improve your debugging process involves using the built-in function dir(). By inspecting objects, you can easily see their attributes and methods, which is incredibly helpful for understanding unfamiliar code or libraries. Another handy tool is the help() function, providing a way to access the documentation directly from the shell. Imagine the time saved by not having to constantly switch between your coding environment and browser tabs.
And let’s not forget list comprehensions, one of Python’s most powerful and expressive features. They allow for manipulating lists in a cleaner and more concise way. Instead of writing multiple lines to filter and map through elements, a single line can achieve the same with better readability. For instance, converting a list of temperatures from Celsius to Fahrenheit could be done in one neat line.
Perhaps you’ve heard about Python’s magic methods, those special methods surrounded by double underscores. Magic methods can enchant your classes, allowing for overloading operators and customizing your objects' behavior. For example, __str__() and __repr__() control how objects are printed, which is invaluable for debugging and logging. These methods make your classes more intuitive and interactive during the development process.
Now, let’s talk about lesser-known libraries that can save you hours of coding. The itertools library offers numerous tools for efficient looping, while functools provides higher-order functions that work with other functions to optimize and streamline your code. By diving into these libraries, you unlock new ways to write more elegant and efficient solutions.
In essence, the tricks of Python are not just about clever snippets of code, but also about embracing the language's philosophy and leveraging its hidden strengths. Fully understanding the potential of these tricks will not only make you a faster coder but also a smarter problem solver. As you continue exploring Python, keep an eye out for those tips that can transform your coding practices and ultimately make your journey in programming a lot more enjoyable.
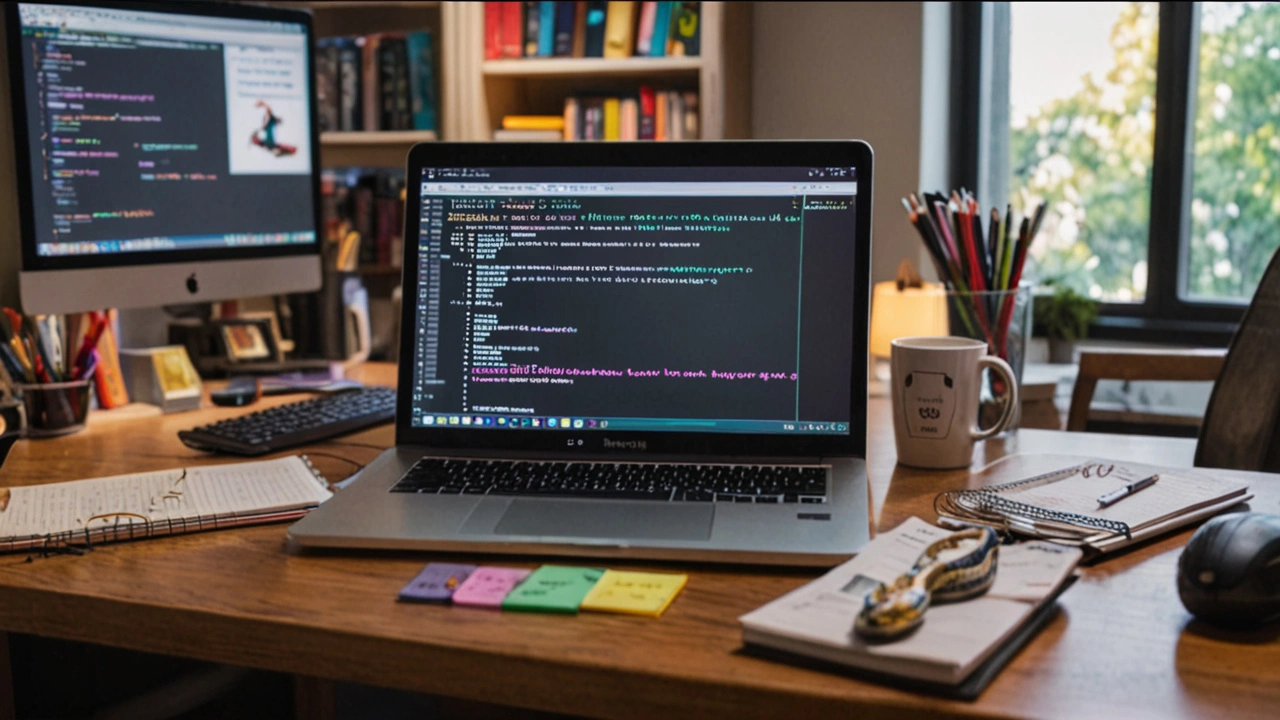
Useful Functions and Methods
When it comes to getting the most out of Python, it's essential to tap into the powerful functions and methods that come built-in with the language. These tools not only simplify your code but also enhance its performance and efficiency. One standout is the range() function. This is a staple for anyone working with loops, as it saves time and keeps your code clean. You can use range() to generate a series of numbers, which is quite handy when you need to iterate over a specific number of times.
Another fundamental function is zip(). This function is like a zipper, interlocking two or more lists into a single iterable. It's an elegant way to combine data from different lists without writing cumbersome loops. Imagine you have two lists: names and scores. With zip(), you can merge them together and effortlessly pair each name with the corresponding score.
The enumerate() function is another gem for its ability to turn an iterable into an enumerate object. This function adds a counter to an iterable and returns it as an enumerate object, so you can easily track the index of items within your loop. This is particularly beneficial when you need both the item and its index in your logic.
Next up is sorted(). This function is indispensable when you need to sort any iterable in Python. From lists to dictionaries, sorted() handles it all with grace. It's particularly powerful when combined with lambda functions, allowing custom sorting logic with ease. Want to sort a list of dictionaries by a particular key? sorted() is the tool for that job.
The filter() function is also worth mentioning. It constructs an iterator from elements of an iterable for which a function returns true. This function is incredibly useful for refining data sets within seconds. Whether you're handling user data or processing financial transactions, filter() helps you extract only the data you need.
One must not overlook map(). This function allows you to apply a specific function to every item in an iterable. It's a concise and readable way to transform data without resorting to more verbose loop structures. Whether you're scaling numbers or formatting strings, map() gets the job done efficiently.
List comprehensions are another Python trick that offers a beautiful blend of readability and functionality. These constructs let you create new lists by applying an expression to each item in an existing list. Readable and often faster, list comprehensions are a must-have in any Python coder's toolkit.
If you need to quickly convert data types, Python offers several conversion functions like int(), float(), str(), and list(). These functions are basic yet incredibly helpful when manipulating different types of data in your code. A common use case is reading input from users, which often comes in as a string, and converting it into the required data type for further processing.
Lastly, we can't forget about the reduce() function from the functools module. While a bit more complex, it's invaluable for cumulative operations. Say you have a list of numbers and you need to compute the product of all elements. reduce() makes this task a breeze, eliminating the need for loop-based accumulation.
"Mastering these functions and methods can genuinely transform your Python programming experience," asserts renowned programmer Michael Kennedy.
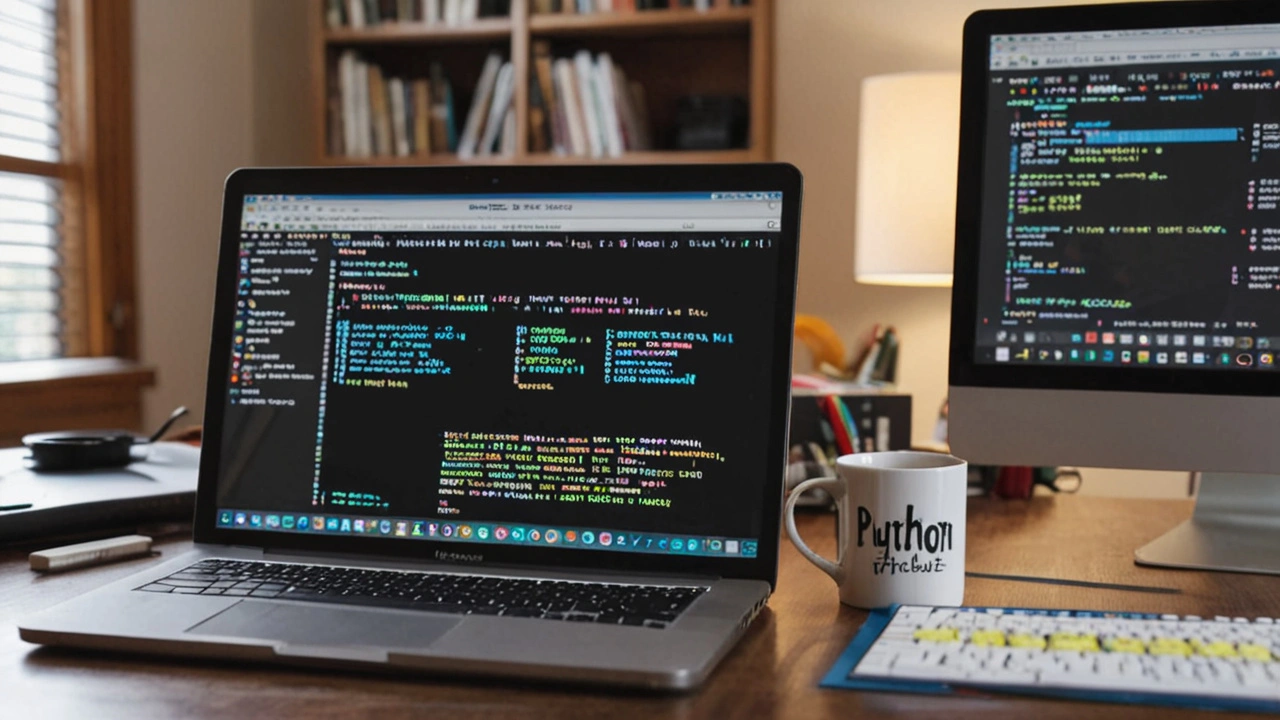
Hidden Features and Shortcuts
Python, by its nature, is a language that keeps on giving. Its straightforward syntax wraps a plethora of functionalities that might not be immediately obvious but are incredibly powerful once you get the hang of them. Let's kick things off by delving into some of the hidden features and shortcuts that can save you time and help you write cleaner, more efficient code.
One of the most intriguing aspects of Python is its ability to handle multiple assignments in a single line. For instance, if you want to swap the values of two variables, you don't need a temporary third variable. You can simply do:
a, b = b, a
This quick line of code simultaneously assigns the value of b
to a
and the value of a
to b
. It's a tiny trick that keeps your code concise and readable.
Another feature worth mentioning is Python's built-in enumerate
function. When you need both the index and the value from a list, enumerate
saves you from manually tracking the index. Here's how you can use it:
for index, value in enumerate(my_list):
print(index, value)
This function comes handy when you're working with loops and need the current index for logic or display purposes.
“Python has a vast standard library that's been built over the years by contributions from programmers all around the world. It’s like a Swiss army knife with a tool for almost any task you might want to do.” — Guido van Rossum, Creator of Python
Moreover, Python supports list comprehensions, which are a concise way to create lists. They can transform lengthy block code into small, readable lines. For example, to create a list of squared numbers, instead of using a loop, you can simply write:
squares = [x ** 2 for x in range(10)]
This not only makes your code cleaner but also more efficient. In a similar vein, there are also dictionary and set comprehensions.
An often overlooked, yet immensely useful feature is the collections
module, which provides alternatives to Python’s general-purpose built-in containers like tuples and dictionaries. For example, defaultdict
simplifies dictionary operations by avoiding key errors:
from collections import defaultdict
my_dict = defaultdict(int)
my_dict['key'] += 1
With defaultdict
, you don't need to check if 'key' exists before incrementing it.
If you’re into object-oriented programming, Python’s property decorators can help in cleaner, more maintainable code. Typically, you might start with a method to get a value, but turning it into a property later lets you access it like an attribute instead of a method:
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
This approach keeps the code cleaner and allows for easy updates if the way the value is calculated changes.
Finally, let's not forget one of the most useful shortcuts for every developer: using virtual environments. Virtual environments help manage dependencies for different projects, ensuring you don't run into version conflicts. With the following steps, you can set up a new virtual environment:
- Install virtual environment:
pip install virtualenv
- Create a new environment:
virtualenv myenv
- Activate it:
source myenv/bin/activate
Using virtual environments ensures a clean state for projects and keeps development smooth. These are just a handful of the many tricks Python offers. Exploring and incorporating them into your coding practice will definitely make you a more efficient programmer.
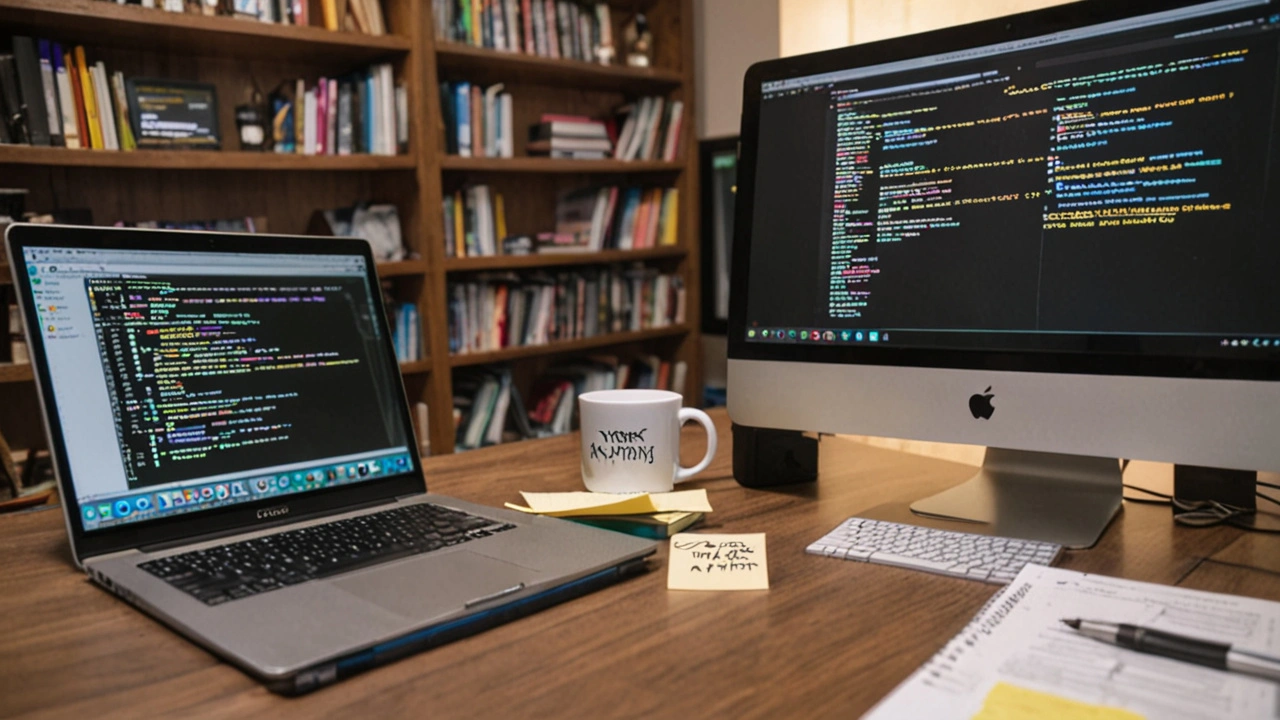
Practical Applications and Examples
The joy of programming in Python lies in the multitudinous ways it can be applied. From web development to data analysis, and even automation, Python's flexibility allows for diverse and innovative uses. Let’s delve into some practical applications and examples that highlight the effectiveness and versatility of this language.
One of the most notable uses of Python is in web development. With popular frameworks like Django and Flask, developers can create robust and scalable web applications. Django is often celebrated for its simplicity and 'batteries-included' philosophy. It comes with many built-in features, such as a user authentication system, which speeds up development time considerably. On the other hand, Flask is a micro-framework that provides the essential tools for web development but allows for more flexibility and control. These frameworks demonstrate Python's ability to cater to different requirements and preferences, making it a favorite among web developers.
Python has become indispensable in the field of data science. Libraries such as Pandas, NumPy, and Matplotlib make data manipulation, analysis, and visualization incredibly straightforward. Pandas, for instance, offers data structures like DataFrames which are perfect for handling structured data and performing operations with ease. Likewise, NumPy allows for efficient computation with its powerful array processing capabilities, while Matplotlib makes it simple to create informative and appealing visualizations. This combination has made it the go-to language for data scientists and analysts who need to handle large datasets and derive insights from them.
Another practical application of Python is in the realm of automation. The simplicity and clarity of Python’s syntax make it an ideal choice for writing scripts that automate repetitive tasks. For example, Python's Selenium library can be used for automating web browser interaction, making it invaluable for tasks like web scraping and automated testing. Another popular library, BeautifulSoup, allows for easy extraction of data from HTML and XML files. These tools empower developers to create efficient scripts that save time and reduce human error.
Let’s not forget Python’s role in artificial intelligence and machine learning. With powerful libraries such as TensorFlow and Keras, it has become a cornerstone for AI development. TensorFlow, developed by Google, provides a comprehensive ecosystem for building, training, and deploying machine learning models. Keras, an API running on top of TensorFlow, makes it easier to design and experiment with neural networks. These libraries have enabled advancements in AI, from image and speech recognition to natural language processing, solidifying Python's reputation as a critical player in cutting-edge technology.
Here is a simple example to illustrate the power of Python in automation:
- First, install the required libraries using the pip command:
pip install requests beautifulsoup4
. - Next, write a script to fetch data from a website:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
else:
print('Failed to retrieve the webpage')
This script uses the requests library to fetch web content and BeautifulSoup to parse it. Such scripts are widely used in data extraction and web scraping, showcasing Python’s practicality in real-world scenarios.
In the words of Guido van Rossum, the creator of Python,
“The ideal programming language will be Python, the ideal one and only perfect language for achieving your goals in technology.”
Engaging with these applications and examples not only enhances your skill set but also opens up new avenues for exploration, making programming in Python not just a task, but a delightful journey.