Code Debugging: The Key to Software Stability
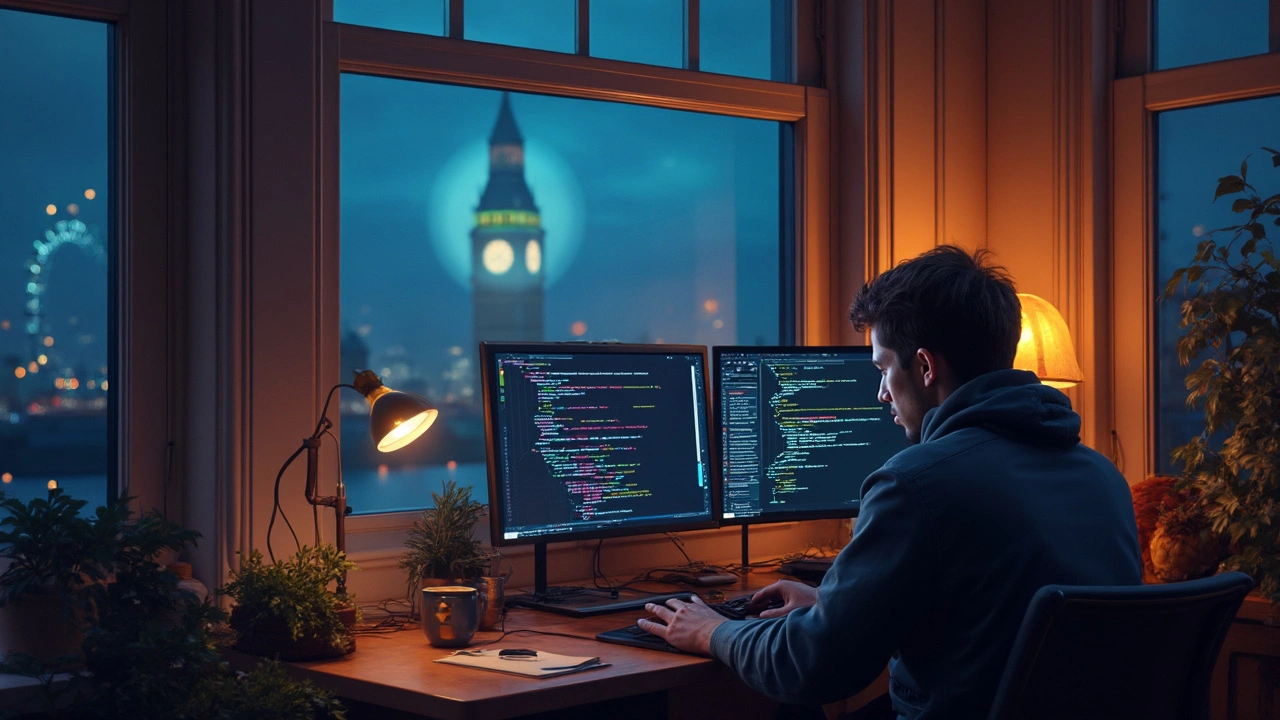
Ever stared at your code wondering why it's behaving like a rogue cat? We've all been there, and that's where debugging steps in to save the day. Debugging is not just a troubleshooting tool but a foundation that software stability rests on. Without it, software would be like a house of cards, waiting to crumble at any misstep.
From catching annoying bugs to unraveling complex logic errors, debugging ensures your code runs smoothly. It's not about writing perfect code from the get-go—it's about improving and learning from mistakes. When you debug, you're diving deep into understanding what your code actually does, which is crucial for creating solid and reliable software.
So, what's the trick to becoming a debugging master? It starts with embracing errors as learning opportunities. Every bug you fix is a step toward better code. But let's face it; some bugs are tougher than others. That's why having the right techniques and tools can make a world of difference. Stay tuned as we delve into these, giving you an edge in the coding world.
- Understanding Debugging
- Impact of Debugging on Stability
- Common Debugging Techniques
- Tools That Make Debugging Easier
- Debugging Best Practices
Understanding Debugging
In the world of programming, debugging is like detective work. Imagine you’re Sherlock Holmes, but instead of solving mysteries in London, you’re unraveling problems in lines of code. Debugging is the process where you carefully inspect your code, looking for the little bugs and errors that cause your program to misbehave.
Why is debugging so essential? Let's picture it this way: each bug you encounter is a small tear in the fabric of your software. Left unattended, these tiny breeches can lead to a full-blown meltdown, causing your software to crash. Bugs can arise from various issues such as syntax errors, logical mistakes, or resource management problems.
Types of Bugs
Understanding the different types of bugs is crucial in becoming proficient at debugging:
- Syntax Errors: These are like typos in your code, simple mistakes that prevent your program from running.
- Logical Errors: Your code runs, but it doesn't do what you expect it to. Like arriving at the wrong bus stop, these errors can be tricky!
- Runtime Errors: These occur when your program is running, often because of unexpected input or conditions.
The Debugging Cycle
Debugging isn't a one-shot deal; it’s an iterative process. You find a bug, fix it, and then test your code again. Here’s a simplified version of the debugging cycle:
- Identify the Bug: Know what’s going wrong. Often, reproducing the error helps pinpoint it.
- Analyze the Code: Study the code surrounding the bug for clues.
- Fix the Issue: Implement a solution to rectify the problem.
- Test the Code: Run your program to ensure the issue is resolved, and check for new bugs.
Fun Fact
Did you know the term 'bug' actually dates back to the 1940s? The story goes that a moth got stuck in a computer at Harvard, causing malfunctions. The team “debugged” it, literally removing the insect!
Understanding these fundamentals of code debugging sets you on the path to writing robust, stable software. It's all about becoming friends with your code and learning its ins and outs. And remember, each bug is a stepping stone to mastering the art of programming.
Impact of Debugging on Stability
Have you ever experienced a crash when using a software application? Most likely, it was due to a bug that slipped through the cracks. This is where the magic of code debugging comes in, playing a vital role in maintaining software stability. Debugging helps catch these pesky bugs before they wreak havoc on a user's experience.
Proper debugging leads to software that's not just stable but also reliable. When software is debugged thoroughly, it reduces the chances of unexpected crashes and the infamous 'runtime errors.' Users hate when a program suddenly closes, and regular debugging ensures that such mishaps are kept to a minimum.
Preventative Measures
One of the greatest benefits of effective debugging is that it acts as a preventative measure. Instead of constantly trying to put out fires, you fix the root issues. This approach significantly lowers maintenance costs and decreases the volume of support requests from disgruntled users.
Stability also correlates with application performance. A stable software will typically run faster and more efficiently since it's not bogged down by hidden errors. If you ignore debugging, you're likely to end up with a sluggish and frustrating user experience.
Debugging Statistics
According to recent research, almost 50% of software crashes are due to unhandled errors—a scenario that a proper debugging process can prevent. A consistent debugging routine not only stabilizes the software but also boosts user satisfaction, leading to higher retention rates.
Percentage of Crashes | Reason |
---|---|
50% | Unhandled Errors |
30% | Logic Errors |
20% | Other Issues |
In conclusion, debugging isn't merely an option; it's a necessity for any serious developer aiming to produce reliable software. It ensures that users have a smooth experience and developers can sleep peacefully at night, knowing they're unlikely to be woken by an urgent bug report at 3 a.m.
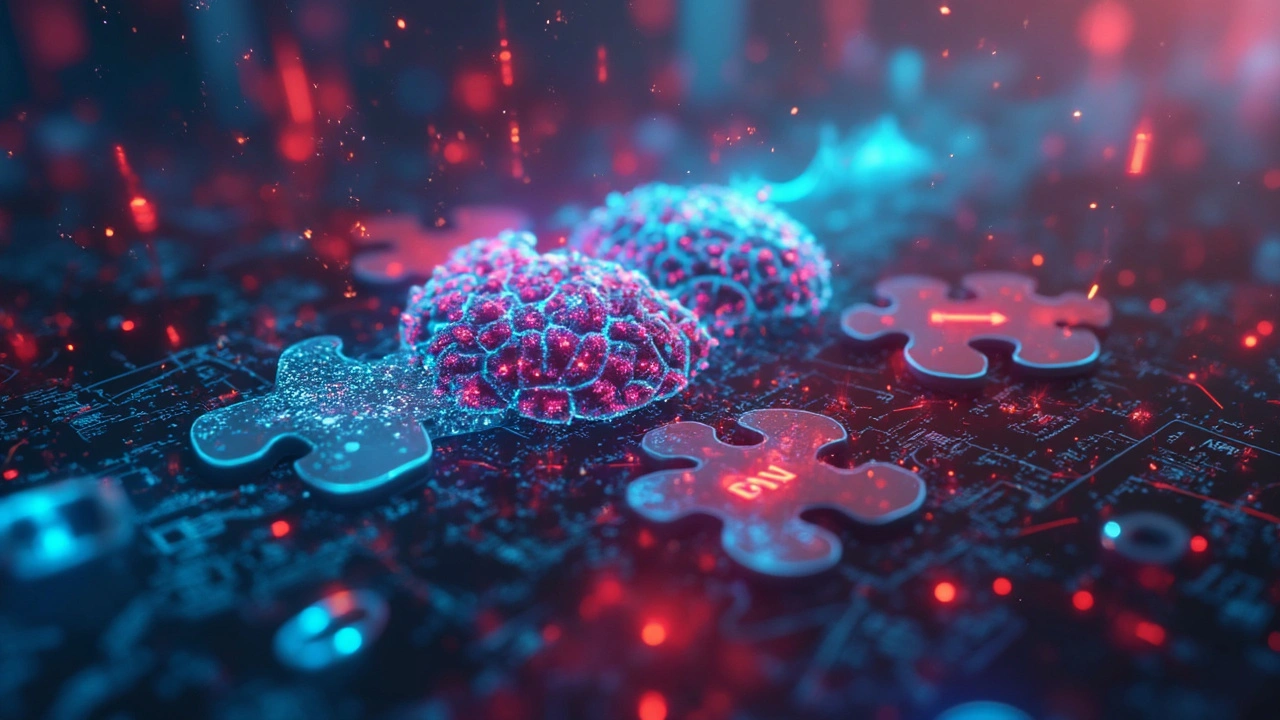
Common Debugging Techniques
Debugging might seem like a daunting task at first, but with the right techniques, it can become second nature. Everyone loves a good shortcut, right? Let's break down some tried-and-tested methods to make your debugging more effective and efficient.
1. Print Debugging
Sometimes the old ways are the best ways. Print debugging is all about inserting print statements in your code to track variable values and program flow. It's like leaving breadcrumbs to see where the code might be going wrong. Simple, yet effective.
2. Using a Debugger
Most IDEs come with built-in debuggers that allow you to step through code line by line. This tool lets you watch how your software executes in real-time, making it easier to catch those pesky bugs. You can set breakpoints, inspect variables, and modify values while the program runs.
3. Rubber Duck Debugging
Ever tried explaining your code line-by-line to a rubber duck? It might sound silly, but this technique forces you to slow down and consider each part of your program. Often, describing the logic out loud highlights errors you overlooked.
4. Logging
Implementing logging in your code helps you keep a record of events as your software runs. Choosing the right logging level (info, warn, debug) can provide insights into what happens under the hood, especially when tackling complex systems.
5. Divide and Conquer
If the issue is elaborate, break the problem into smaller parts. Test each section independently to isolate where the error might be hiding. This approach helps you narrow down the cause without overwhelming yourself.
Debugging Stats
Here's an interesting snippet: A study found that developers spend around 50% of their time debugging. With these techniques, you can cut down on that time, making your work more efficient and enjoyable.
Tools That Make Debugging Easier
Ah, the sheer frustration of chasing down a bug without any handy tools— it's like trying to catch fish with your bare hands. But thankfully, we live in a time where we've got a whole toolkit of debugging aids at our fingertips!
First up on the list is the trusty Integrated Development Environment (IDE). IDEs like Visual Studio, IntelliJ IDEA, and Eclipse aren't just there to write code but are packed with built-in debugging features. They let you set breakpoints, examine variables, and step through your code line by line. If you’ve ever tried to find a needle in a haystack, you know how useful that can be.
Specialized Debugging Tools
For those coding in web environments, don't overlook your browser's developer tools. Chrome DevTools, for instance, is like your handy Swiss Army knife. It offers a suite of functions including JavaScript debugging and performance profiling. This is ideal for front-end developers who need real-time debugging help.
On the other end, you've got tools like GDB for C/C++ developers. It's command-line heavy, but don't let that scare you. Once you get the hang of it, GDB unveils a wealth of information about what's going wrong in your compiled code.
Diagnostics and Profiling
Sometimes, keeping your code running smoothly means understanding its performance, not just its correctness. Enter profiler tools like Valgrind or JProfiler. Profilers help you measure your code's performance, diagnosing bottlenecks, and memory leaks. It’s like giving your software a health check-up.
- Valgrind: Fantastic for memory debugging, it detects memory leaks and other memory management bugs in C/C++.
- JProfiler: Primarily used for Java applications, it provides detailed CPU and memory metrics that can help optimize performance.
If diving into these tools feels daunting, remember, each one has a supportive community and plenty of tutorials to get you started. So, the next time your code throws a tantrum, grab these debugging tools, and you'll see the magic of software stability unfold!
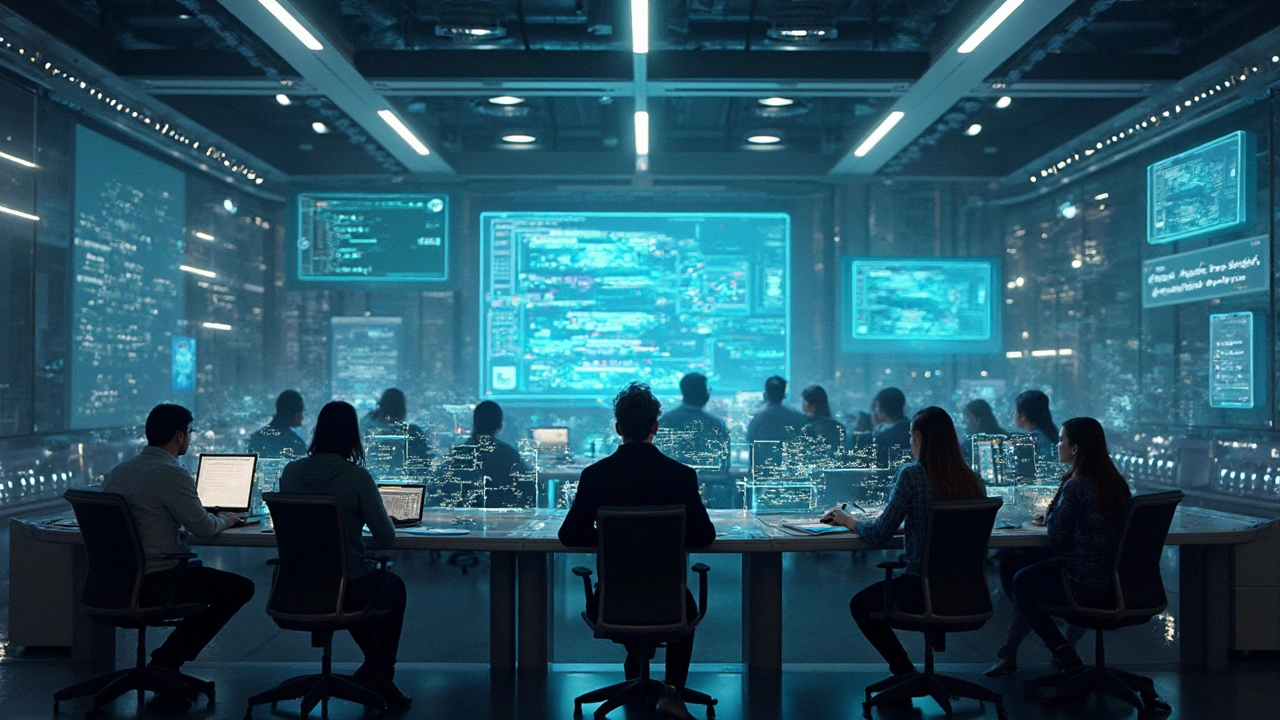
Debugging Best Practices
Let's dig into some tried-and-true practices that can make your code debugging journey a lot smoother. Debugging might feel like chasing a ghost sometimes, but the right approach can turn that hunt into a logical pursuit.
Start with Breakpoints
One of the most reliable tools in your debugging arsenal is setting breakpoints. These handy markers help you pause the program at certain spots, revealing its current state. It’s like pulling back the curtain to catch your code red-handed in the act. Use them strategically to home in on the root of the issue.
Keep a Detailed Log
Logging provides a breadcrumb trail of what your program is doing over time. By keeping insightful logs, you can identify where things went haywire. Make sure your logs are descriptive enough to be helpful but not so much that you're drowning in data.
Joan Harris, Senior Software Engineer at Code Solutions, once said, "Good logging is like a map; it guides you through the maze of your program's execution."
Adopt a Systematic Approach
When tracking down bugs, methodically eliminate possibilities. This structured method is often faster than wild guessing. Start by confirming what you know works, then move step-by-step to areas that might have issues.
Peer Reviews and Pair Debugging
Sometimes, two heads are better than one. Collaborating with fellow developers can offer fresh perspectives and insights. A colleague might spot something you've overlooked or suggest an alternative solution you haven't considered.
Use Debugging Tools
Utilize the powerful debugging tools offered by modern IDEs. These tools provide features like watch variables, call stacks, and memory snapshots. They might feel overwhelming at first, but mastering them can drastically shorten your debugging time.
Tool | Benefit |
---|---|
Console Loggers | Quick feedback on code execution |
Remote Debuggers | Essential for diagnosing server-side issues |
IDE Breakpoints | Interactively pause and inspect code |
By mastering these debugging practices, you not only make your software more stable but also sharpen your programming skills significantly. It's all about finding what works best for you and running with it.