Mastering PHP: Time-Saving Coding Techniques for Web Developers
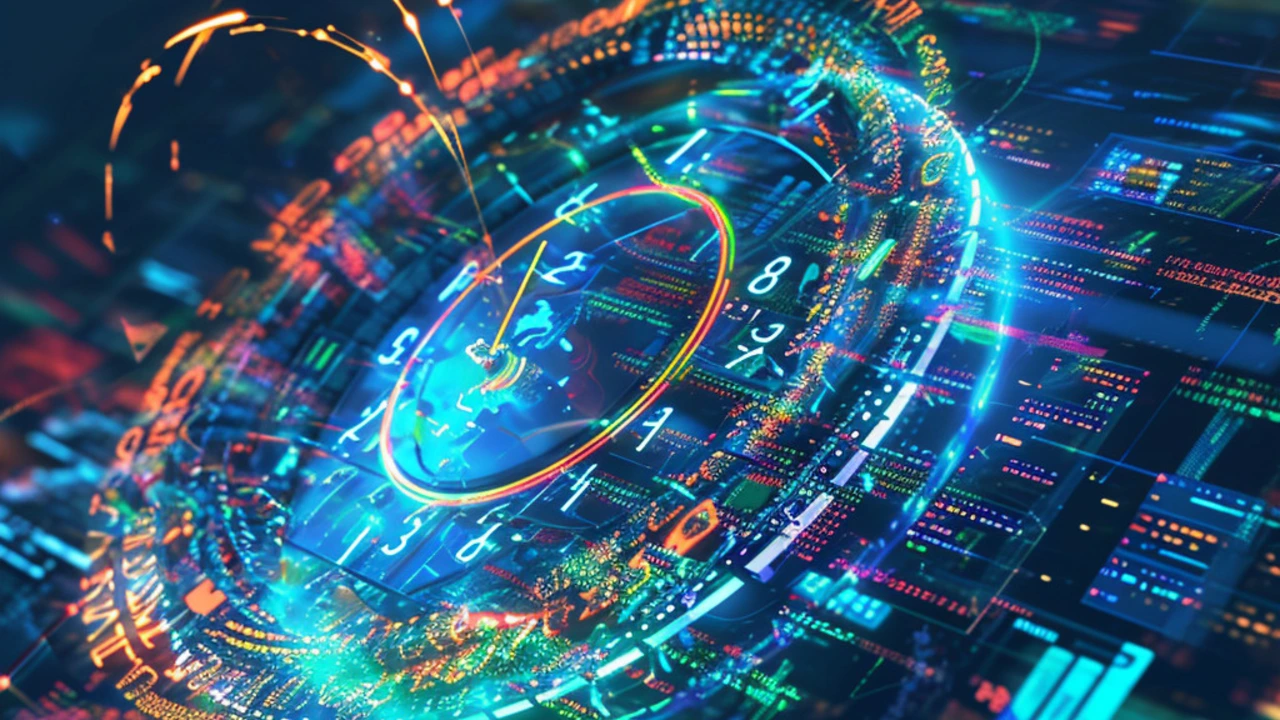
Unlocking the Power of Built-in Functions
Let's dive right into one of the biggest treasures of PHP - its wealth of built-in functions. PHP offers functions for nearly every need you can think of, from array manipulation to variable testing. It's easy to reinvent the wheel, but why spend hours coding a function from scratch when PHP probably has one ready to use? For example, array_filter() and array_map() can save you countless lines of code for complex array data handling. Proper use of these functions can mean the difference between a script that zips along and one that plods through tasks.
In addition to general functions, PHP also has numerous built-in functions tailored for specific uses, such as date() for date and time, and gd library functions for image processing. Knowing what's available in the PHP standard library is essential, as it can greatly decrease development time and increase the reliability of your code. A good practice is to regularly check the PHP manual for the latest functions and updates, ensuring your toolkit is always up-to-date.
String Manipulation Mastery
Strings are a cornerstone of web development, and in PHP, they're a breeze to manipulate if you know how. There's a function for almost every operation you might want to perform on a string, like concatenation, splitting, or even regular expression matching with preg_match(). Understanding the nuances of string functions like strpos(), strstr(), and substr() will empower you to write succinct and readable string operations.
However, remember that strings in PHP can work with different encodings, and some functions are not multibyte-safe. This is where mb_* functions come into play, ensuring that your application handles strings correctly in various character encodings. String manipulation is not just about tweaking texts; it's about data integrity and user experience. Therefore, mastering string functions will help you to not only code efficiently but also to uphold the quality of your application's output.
Efficient Database Interaction
Interacting with databases is often a sizable part of a PHP project. But it's also an area where efficiency can make a substantial impact. Using PDO (PHP Data Objects) can create a database-agnostic application, allowing you to switch the underlying database without rewriting your data access code. Prepared statements, another feature of PDO, not only improve security by preventing SQL injection but can also boost performance when executing similar queries multiple times.
Additionally, understanding how to leverage database indexing, caching results with memcached or Redis, and using transactions can significantly reduce the load on your database server and speed up your application. It's not enough to just retrieve data; knowing how to do it well is essential. Efficient database interactions are about planning queries, understanding your database's strengths, and utilizing the right PHP features to get the job done quickly and securely.
Effective Error Handling and Debugging
Error handling and debugging are critical for creating robust PHP applications. It's not just about locating and fixing errors, but also about predicting where things might go wrong and handling those situations gracefully. Using try/catch blocks and setting an effective error handler with set_error_handler() can transform a potential page-breaking moment into a controlled response. Get comfortable in using exceptions and practicing proper error logging; this can save you hours of troubleshooting down the line.
Moreover, tools like Xdebug can help you step through your code and find problems quickly. Remember, a good developer not only knows how to write code but also how to fix and optimize it. Exception handling and debugging are part and parcel of efficient programming, and PHP provides all the necessary tools to make this process as pain-free as possible.
Maximizing Performance with Caching Techniques
Caching is a must-know for any PHP developer looking to speed up their applications. Implementing caching with PHP can be surprisingly simple with tools like OPcache for opcode caching, which can drastically reduce the time taken to run PHP scripts. Caching your data with APCu for storing application variables or using HTTP cache headers to inform browsers how to cache your pages can dramatically decrease load times for your users.
There's also object caching, which involves storing data objects in memory to be reused by PHP without requiring a new database query or computation. This can be particularly effective in reducing the load on your database and speeding up user experience. By strategically using these various caching techniques, your PHP application can benefit from a considerable performance boost, ensuring users are not left waiting.
Leveraging PHP Frameworks for Rapid Development
Now, onto frameworks. They're not just a trend; they're a smart way to reduce development workload and produce cleaner, more standardized code. Frameworks such as Laravel or Symfony provide a structure for developing your PHP applications along with tons of prewritten components. Embracing a framework can lead to significant efficiency gains. For instance, using Laravel's Eloquent ORM for database operations or Symfony's Console component to create CLI tools might just change your life.
Remember, frameworks also bring best practices to your development process and often come with built-in security features, keeping you and your end-users safer. However, each framework has its own learning curve, and it's important to choose one that fits your project's needs. Don't overlook the power of PHP frameworks in your quest for efficient coding; they can be mighty allies.
Final Thoughts on PHP Productivity
Alright, let's wrap this up. Becoming efficient in PHP coding is about more than just pumping out code; it's about writing smart code. With the tricks and techniques discussed here—from taking full advantage of built-in functions and mastering string operations, to efficient database interactions, error handling, caching, and utilizing frameworks—you're setting yourself up for PHP success. These practices can shrink your development time, beef up performance, and result in cleaner, more maintainable code.
Remember, every project brings new challenges and learning opportunities. Keep refining your skills, stay in touch with community best practices, and don't be afraid to refactor and improve existing code. After all, efficient coding is a journey, not a destination. Here's to writing PHP that's as powerful as it is eloquent!